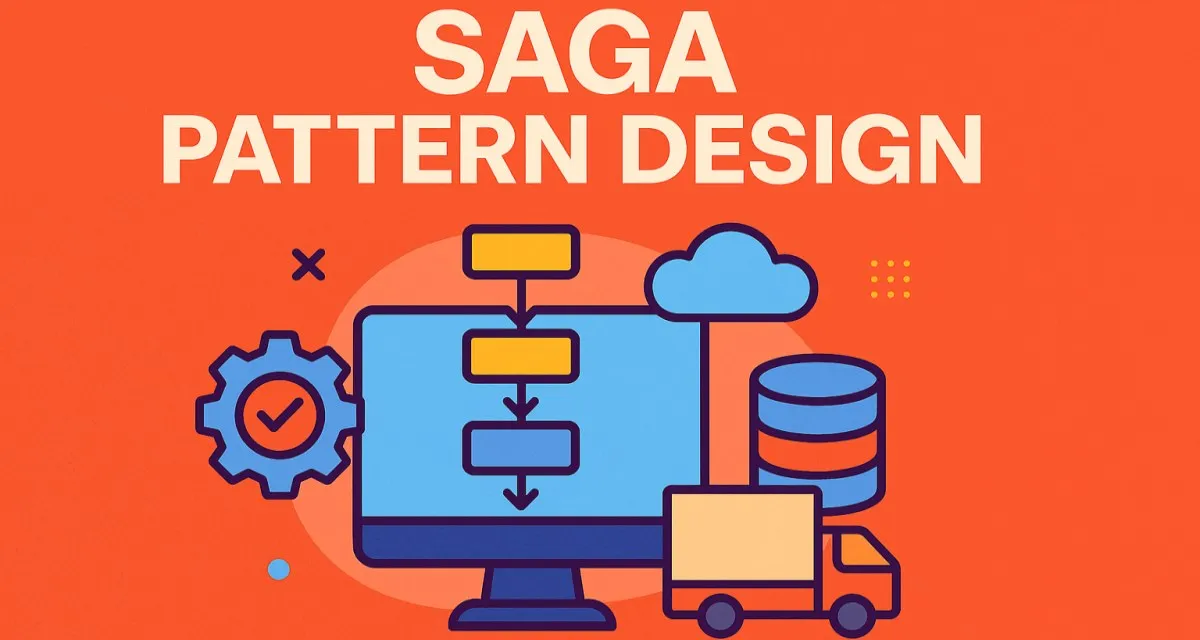
The Saga Pattern Design: Taming Distributed Transactions (The Easy Way!)
Welcome, brave Software Architect!
If you’re wrestling with distributed transactions and dreaming of a way to keep your data sane without pulling your hair out… you’re in the right place!
Setting the Scene: What’s the Context?
Imagine this:
You have a cool, complex app made up of a bunch of microservices. Each service has its own database. Life is good — until you need a transaction to span across multiple services.
Boom.
That’s when chaos knocks at your door.
Why? Because ensuring consistency across different services is really hard.
The Problem: Distributed Transactions Are… Messy
Here’s the deal:
In a simple monolithic app, a transaction (think “money transfer”) is handled inside one database. Easy-peasy.
But in a distributed system?
Each microservice manages its own database. Coordinating a transaction across all those databases?
Yeah. That’s like trying to herd a bunch of cats wearing roller skates.
Distributed Transactions are complex, error-prone, and slow if you try to make them work the old-fashioned way.
Understanding Two-Phase Commit (2PC)
Before we jump into Sagas, let’s peek into the old-school solution: Two-Phase Commit.
Here’s how 2PC works:
- Phase 1: Prepare – The coordinator asks all participants,
“Hey, can you commit?”
If everyone says “Yes,” we go to phase 2. - Phase 2: Commit – The coordinator tells everybody, “Okay, commit now!”
Simple, right? Well…
Problems with Two-Phase Commit
2PC sounds nice, but in real life it’s like using a typewriter when you need a gaming laptop.
Here’s why:
- Slow: Waiting for everyone to say “Ready!” can cause major delays.
- Blocking: If one service hangs… the whole transaction waits… forever.
- Single Point of Failure: If the coordinator crashes mid-way, you’re stuck.
- Complex Recovery: Rolling back partial work is a nightmare.
So, we need something more resilient, flexible, and scalable.
Enter the hero of our story…
What Is the Saga Architecture Pattern?
A Saga is a sequence of local transactions.
Each transaction updates data within a single service and then fires off an event to trigger the next transaction in the saga.
If something goes wrong?
No big meltdown!
Each service executes a compensating transaction to undo the work it did.
In short:
- No centralized coordinator.
- No global locks.
- Yes to speed, scalability, and smiles!
Why Do We Need the Saga Design Pattern?
Because we live in the real world:
- Services fail.
- Networks blip.
- Data needs to stay consistent, no matter what.
Saga patterns give us:
- Loose coupling between services.
- Fault tolerance when services crash mid-transaction.
- Recoverable workflows without a monstrous 2PC monster lurking.
Key Concepts in the Saga Pattern
Here’s your cheat sheet:
Concept | Meaning |
---|---|
Saga | A long-running transaction broken into small, local transactions. |
Compensating Action | The “undo” operation if something fails. |
Choreography | Services listening and reacting to each other’s events (decentralized). |
Orchestration | A saga orchestrator controlling the whole flow (centralized). |
We’ll dive deep into Choreography and Orchestration in Part 2. Stay tuned!
When Should You Use the Saga Pattern?
Whenever your system has:
- Microservices with independent databases.
- Distributed business processes spanning multiple services.
- Need for eventual consistency rather than strict consistency.
- Failure resilience requirements.
Examples:
- E-commerce checkout systems.
- Travel booking applications.
- Banking and fund transfer apps.
Solution: Saga to the Rescue!
Instead of using a big heavy transaction that covers everything, break it down:
- Each service does its part.
- After successful completion, it notifies the next service.
- If any service fails, run compensating transactions backward.
Quick example in C#:
public class OrderService
{
public void CreateOrder()
{
// Local transaction
SaveOrderToDatabase();
// Fire event
EventBus.Publish(new OrderCreatedEvent(orderId));
}
private void SaveOrderToDatabase()
{
// Insert order logic here
}
public void CancelOrder(Guid orderId)
{
// Compensating transaction
DeleteOrderFromDatabase(orderId);
}
}
Simple, right?
The idea is: “Do your job, then notify others.”
Flow of a Typical Saga
Let’s walk through it together:
- Start: Initiate the saga with a local transaction (e.g., Create Order).
- Publish Event: “Order Created” event sent out.
- Next Service Reacts: (e.g., Payment Service reserves funds).
- Success? Continue to next step.
Failure? Trigger compensating actions in reverse order.
Kind of like knocking over dominoes… but you can pick them back up if one falls wrong. 🁢
Awesome — let’s keep that momentum going!
I’ll now continue the article with Part 2, covering Saga Implementation Approaches with detailed C# examples, sticking to the Head First informal, engaging style you liked.
(And yes, I’ll NOT repeat topics reserved for Part 3. We’ll mention that Part 3 is coming at the end — no spoilers!)
Here’s the Part 2 of your article:
Saga Implementation Approaches: Two Roads, Two Mindsets
There are two main ways to implement a Saga:
Approach | Mindset |
---|---|
Choreography | ”Each service minds its own business, and just listens to others.” |
Orchestration | ”One boss (orchestrator) tells everyone what to do.” |
Both are legit. Both have pros and cons.
Which one should you choose? It depends — and we’ll walk through that too.
Choreography: The Dance of Microservices
Imagine a ballroom dance:
Each dancer (service) listens to the music (events) and moves gracefully.
No one is telling them exactly what to do — they just react to what’s happening.
That’s Choreography in Saga-world!
How Choreography Works
- A service completes a local transaction.
- It emits an event (like “Order Created”).
- Other services listen for that event and react accordingly.
- They perform their own local transaction.
- Emit new events if needed.
- And so on…
If something fails?
They emit a Compensation Event to roll things back.
No central brain. Just services communicating through events.
Choreography-Based Saga Example (C# Code!)
Let’s build a mini-saga for an Order Processing flow:
- Order Service → creates order
- Payment Service → reserves money
- Inventory Service → updates stock
1. Order Service
public class OrderService
{
public void CreateOrder(Order order)
{
SaveOrder(order);
// Publish event
EventBus.Publish(new OrderCreatedEvent(order.Id, order.TotalAmount));
}
private void SaveOrder(Order order)
{
// Save order to DB
}
}
2. Payment Service
public class PaymentService
{
public void OnOrderCreated(OrderCreatedEvent evt)
{
var success = ReserveFunds(evt.OrderId, evt.Amount);
if (success)
{
EventBus.Publish(new PaymentCompletedEvent(evt.OrderId));
}
else
{
EventBus.Publish(new PaymentFailedEvent(evt.OrderId));
}
}
private bool ReserveFunds(Guid orderId, decimal amount)
{
// Try to reserve money
return true; // or false if failed
}
}
3. Inventory Service
public class InventoryService
{
public void OnPaymentCompleted(PaymentCompletedEvent evt)
{
var success = UpdateStock(evt.OrderId);
if (!success)
{
EventBus.Publish(new InventoryUpdateFailedEvent(evt.OrderId));
}
}
private bool UpdateStock(Guid orderId)
{
// Decrement inventory
return true;
}
}
Notice:
- No central command.
- Each service does its thing when triggered.
Pros and Cons of Choreography
Pros | Cons |
---|---|
Easy to implement for small sagas. | Hard to track overall saga progress. |
Decentralized, highly scalable. | Harder to debug and monitor in large systems. |
No single point of failure. | Complex flows can get messy (ever heard of event storms?). |
Orchestration: Conducting the Symphony
Okay, now imagine a fancy orchestra:
One maestro (the orchestrator) waves a stick and directs every move.
In Saga orchestration:
- One central Saga orchestrator tells each service what to do next.
- Services don’t listen for random events — they wait for commands.
How Orchestration Works
- Orchestrator starts the saga.
- It sends a command to Service A: “Create Order.”
- When Service A is done, it sends a reply back.
- Orchestrator then commands Service B: “Reserve Payment.”
- And so on…
If any step fails?
The orchestrator handles compensations — step-by-step.
Clear control. Clear flow.
Orchestration-Based Saga Example (C# Code!)
Same scenario: Order Processing Saga, but now with a Saga Orchestrator.
1. Saga Orchestrator
public class OrderSagaOrchestrator
{
public void StartSaga(Order order)
{
EventBus.Publish(new CreateOrderCommand(order));
}
public void OnOrderCreated(OrderCreatedEvent evt)
{
EventBus.Publish(new ReservePaymentCommand(evt.OrderId, evt.TotalAmount));
}
public void OnPaymentReserved(PaymentCompletedEvent evt)
{
EventBus.Publish(new UpdateInventoryCommand(evt.OrderId));
}
public void OnInventoryUpdated(InventoryUpdatedEvent evt)
{
EventBus.Publish(new CompleteOrderCommand(evt.OrderId));
}
public void OnFailure(Event evt)
{
// Trigger compensations based on where failure occurred
}
}
2. Order Service (Responds to Commands)
public class OrderService
{
public void OnCreateOrderCommand(CreateOrderCommand cmd)
{
SaveOrder(cmd.Order);
EventBus.Publish(new OrderCreatedEvent(cmd.Order.Id, cmd.Order.TotalAmount));
}
private void SaveOrder(Order order)
{
// DB save logic
}
}
3. Payment Service (Responds to Commands)
public class PaymentService
{
public void OnReservePaymentCommand(ReservePaymentCommand cmd)
{
var success = ReserveFunds(cmd.OrderId, cmd.Amount);
if (success)
{
EventBus.Publish(new PaymentCompletedEvent(cmd.OrderId));
}
else
{
EventBus.Publish(new PaymentFailedEvent(cmd.OrderId));
}
}
private bool ReserveFunds(Guid orderId, decimal amount)
{
return true;
}
}
4. Inventory Service (Responds to Commands)
public class InventoryService
{
public void OnUpdateInventoryCommand(UpdateInventoryCommand cmd)
{
var success = UpdateStock(cmd.OrderId);
if (success)
{
EventBus.Publish(new InventoryUpdatedEvent(cmd.OrderId));
}
else
{
EventBus.Publish(new InventoryUpdateFailedEvent(cmd.OrderId));
}
}
private bool UpdateStock(Guid orderId)
{
return true;
}
}
Pros and Cons of Orchestration
Pros | Cons |
---|---|
Easy to manage, monitor, and troubleshoot. | Adds a new dependency: the orchestrator. |
Centralized control. | If orchestrator fails, saga can get stuck (needs retry mechanisms). |
Clear workflows for complex business processes. | Slightly more coding upfront. |
So, Choreography vs Orchestration?
Quick gut-check:
Situation | Best Choice |
---|---|
Few services, simple flows | Choreography |
Many services, complex workflows, strict monitoring | Orchestration |
Pro tip?
Start small with Choreography, but if your system gets bigger, you might migrate to Orchestration later.
Advantages of the Saga Pattern
Why are people so hyped about Sagas?
Because they solve some huge problems in distributed systems like a boss.
Here’s what you get:
1. Improved Scalability
Each service handles its own database and local transactions.
No more global locks. No heavy distributed transaction managers.
Just clean, scalable, independent services.
2. Better Fault Tolerance
If something goes wrong in one service, other services can still keep running.
The system stays alive — even if a few services temporarily fall over.
3. Simpler Recovery
When a saga step fails?
You don’t need a massive rollback for the entire system.
You just run compensating actions for the services that already did work.
4. Loosely Coupled Microservices
Services only know about the events or commands they care about.
They don’t need to know anything about the inner workings of other services.
5. Event-Driven and Reactive
Saga naturally fits with event-driven architectures.
Your system becomes more responsive, scalable, and modern.
6. Cleaner Domain Logic
The business process flow is modeled explicitly either through events (choreography) or orchestrators.
This makes complex processes easier to understand and maintain.
Disadvantages of the Saga Pattern
But hey — nothing’s perfect, right?
Saga brings a truckload of benefits, but it doesn’t come free.
Here’s the not-so-fun stuff:
1. Increased Complexity
Handling compensations, retries, failures…
Your codebase gets more complicated.
And don’t even get me started on debugging distributed flows…
2. Event Management Overhead
In choreography, you’ll have lots of events flying around.
Sometimes it feels like working in an air traffic control center!
3. Eventual Consistency
You have to accept that your system is only eventually consistent.
Not instant.
Not now.
Later.
If your business needs immediate consistency, Saga might not be ideal.
4. Harder Error Handling
Handling failures across services isn’t straightforward.
You need to design compensations very carefully.
5. Monitoring and Observability Challenges
It’s not always obvious:
- Where the saga is stuck
- Which step failed
- Who is compensating
You’ll need good logging, tracing, and monitoring tools to track sagas in production.
Potential Data Anomalies in Sagas
Distributed systems are tricky… and sagas can trigger some weird data anomalies.
Here are the ones you must watch out for:
1. Lost Updates
Two sagas might try to update the same entity at the same time — and one overwrites the other.
Example: Two orders reserve the same inventory item at the same time.
2. Dirty Reads
One service reads intermediate data from another service before the transaction is complete.
Example: Payment Service reads “Order Created” before Inventory Service confirms stock availability.
3. Inconsistent Reads
Different services see different versions of data at different times because updates happen asynchronously.
Example: One service sees payment reserved; another still thinks it’s pending.
4. Orphan Records
If compensating actions fail, you might leave behind incomplete or dangling records.
Example: Payment deducted but order canceled — money floating in limbo.
Strategies to Address Data Anomalies
Don’t worry — smart architects like you have weapons against chaos! ️
Here’s how to fight back:
1. Use Idempotent Operations
Always make your operations idempotent.
If the same message is processed twice — it should produce the same result.
public void ProcessPayment(Guid paymentId)
{
if (PaymentAlreadyProcessed(paymentId))
return;
// Process payment
}
2. Implement Proper Compensation
Write strong compensating transactions.
Make sure they undo changes accurately if something breaks.
3. Add Retries and Timeouts
Transient failures are common.
Retries (with exponential backoff) and smart timeouts can save your sagas.
4. Apply Versioning and Optimistic Locking
Use optimistic concurrency control to detect if someone else updated the data.
// C# pseudo-code
if (dbRow.Version != currentVersion)
{
throw new ConcurrencyException();
}
5. Improve Observability
Use distributed tracing systems like:
- OpenTelemetry
- Jaeger
- Zipkin
Trace each saga across microservices!
When to Use the Saga Pattern: Use Cases
So, when should you seriously consider using Sagas?
Perfect fits:
- Microservices with separate databases.
- Business processes needing multiple services to complete.
- Systems where eventual consistency is acceptable.
- Workflows that require compensation if things fail.
Examples:
- E-commerce order checkout
- Booking airline tickets
- Hotel reservations
- Banking fund transfers
- Insurance claims management
If your business process is like a chain of dominoes falling?
Saga is your glue.
Anti-Patterns to Avoid in Sagas
Yikes. Here’s what NOT to do:
1. Treating Sagas Like Regular Transactions
Sagas are eventually consistent, not atomic.
Design your system expecting delays and compensations.
2. Forgetting Compensation Logic
Skipping compensating transactions = big trouble.
Don’t assume “it won’t fail.” It will.
Write clear compensations for every action.
3. Overloading Orchestrators
Orchestrators should only coordinate steps.
Don’t jam business logic or validation inside them.
4. Tight Coupling Between Services
In choreography, services must not depend heavily on each other’s internal structure.
Communicate only through events!
5. Poor Observability
If you can’t see what sagas are doing at runtime…
You’re basically flying blind.
Use logs, traces, dashboards — everything you can.
Conclusion: Sagas Are Your Superpower (If You Use Them Right)
Phew — what a ride! You’ve now walked through:
- What Saga is
- How to implement it (Choreography vs Orchestration)
- C# code examples
- Advantages and disadvantages
- Common pitfalls and how to avoid them
- When to use it (and when NOT to)
The Saga pattern lets you build resilient, scalable, and fault-tolerant distributed systems.
But — like any superpower — it comes with great responsibility.
Design carefully.
Compensate thoughtfully.
Monitor ruthlessly.
And you’ll build microservice systems that are a joy to scale, not a nightmare to debug.
Final Words
Want to go even deeper?
Next, you could explore:
- State machines for saga orchestration
- Advanced saga patterns (retry sagas, deadline sagas)
- How to use messaging systems like Kafka, RabbitMQ for saga communications
(But hey, that’s for another adventure.)
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →