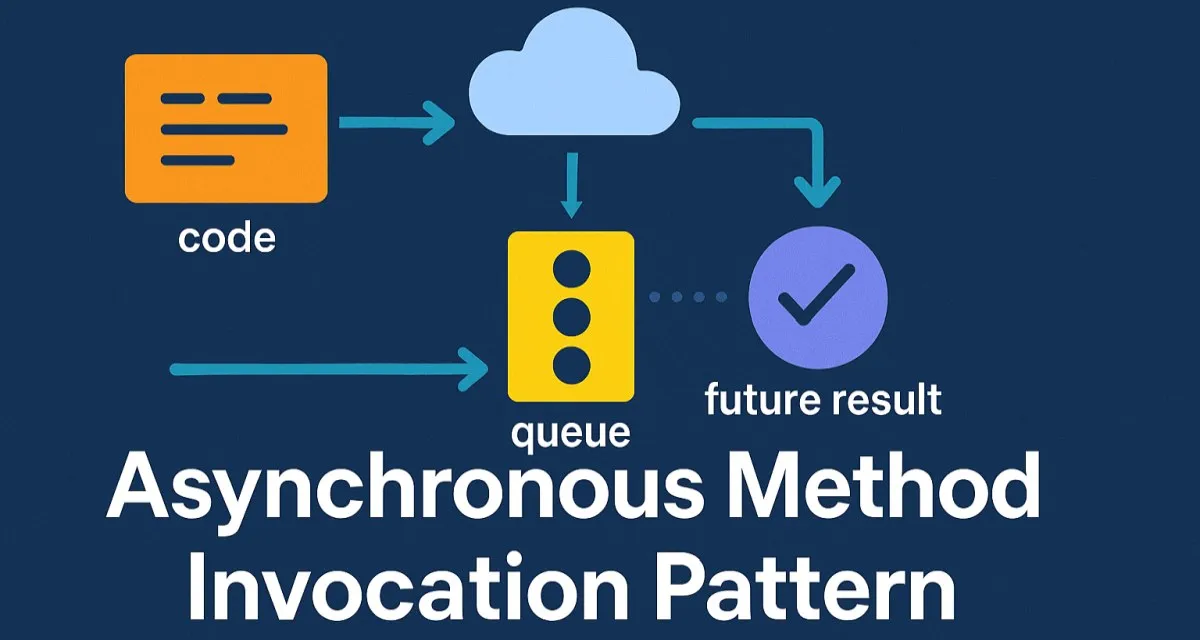
Asynchronous Method Invocation Design Pattern: A Comprehensive Guide for Software Architects
Introduction
Imagine you’re at a restaurant. You place your order, and instead of waiting idly at the counter, you return to your table, engage in conversation, or check your phone. When your meal is ready, the server brings it to you. This is akin to how the Asynchronous Method Invocation (AMI) design pattern operates in software development.
In traditional synchronous programming, a method call waits (or blocks) until it completes before moving on to the next task. This can lead to inefficiencies, especially when dealing with long-running operations like file I/O, network requests, or database queries. The AMI pattern addresses this by allowing methods to execute asynchronously, enabling the application to remain responsive and efficient.
In this article, we’ll delve deep into the AMI design pattern, exploring its principles, appropriate use cases, key components, and detailed implementation in C#. Whether you’re a seasoned software architect or a developer aiming to enhance application performance, this guide will provide valuable insights.
What is the Asynchronous Method Invocation Design Pattern?
The Asynchronous Method Invocation (AMI) design pattern is a programming paradigm that allows a method to be invoked asynchronously, meaning the caller does not wait for the method to complete before proceeding. Instead, the method executes in the background, and the caller is notified upon its completion, either through callbacks, events, or polling mechanisms.
This pattern is particularly beneficial in scenarios where operations are time-consuming or resource-intensive, as it prevents the application from becoming unresponsive during these operations.
Principles of the AMI Design Pattern
Understanding the core principles of the AMI pattern is crucial for its effective implementation:
-
Non-Blocking Operations: The primary goal is to prevent the calling thread from being blocked while waiting for a method to complete. This ensures that the application remains responsive.
-
Separation of Concerns: The initiation of the method and the handling of its result are decoupled. This separation allows for better modularity and maintainability.
-
Concurrency Management: By executing methods asynchronously, the pattern leverages concurrent processing, making efficient use of system resources.
-
Callback Mechanisms: Upon completion of the asynchronous operation, the result is communicated back to the caller through callbacks, events, or other notification mechanisms.
-
Error Handling: Proper mechanisms are in place to handle exceptions or errors that may occur during the asynchronous operation.
When to Use the AMI Design Pattern
The AMI pattern is not a one-size-fits-all solution. It’s essential to assess whether it’s appropriate for your specific scenario. Here are situations where AMI is particularly beneficial:
-
Long-Running Operations: Tasks like file I/O, network communication, or complex computations that take significant time to complete.
-
User Interface Responsiveness: In GUI applications, to prevent the UI from freezing during lengthy operations.
-
Scalable Web Services: For handling multiple client requests concurrently without blocking threads.
-
Resource Optimization: To make efficient use of system resources by allowing other tasks to proceed while waiting for an operation to complete.
-
Improved Throughput: In server applications, to handle more requests by not tying up threads with blocking operations.
However, if the operation is quick and doesn’t significantly impact performance, introducing asynchronous complexity might not be justified.
Key Components of the AMI Design Pattern
Implementing the AMI pattern involves several key components:
-
Asynchronous Method: The method that initiates the operation and returns immediately, allowing the caller to continue execution.
-
Callback or Event Handler: A mechanism through which the result of the asynchronous operation is communicated back to the caller.
-
Future or Promise: An object representing the eventual result of the asynchronous operation. It allows the caller to retrieve the result once it’s available.
-
Executor or Scheduler: Manages the execution of asynchronous tasks, often by utilizing thread pools or task queues.
-
Error Handling Mechanism: Ensures that any exceptions or errors during the asynchronous operation are appropriately handled and communicated.
Implementing the AMI Design Pattern in C#
C# provides robust support for asynchronous programming, primarily through the async
and await
keywords introduced in .NET Framework 4.5. Let’s explore how to implement the AMI pattern in C# with detailed examples.
Example Scenario: Fetching Data from a Web API
Suppose we have a method that fetches data from a web API. This operation can be time-consuming due to network latency, making it a prime candidate for asynchronous execution.
Synchronous Implementation
public string FetchData()
{
using (var client = new HttpClient())
{
var response = client.GetStringAsync("https://api.example.com/data").Result;
return response;
}
}
In this synchronous version, the method blocks until the data is fetched, potentially causing the application to become unresponsive.
Asynchronous Implementation Using async/await
public async Task<string> FetchDataAsync()
{
using (var client = new HttpClient())
{
var response = await client.GetStringAsync("https://api.example.com/data");
return response;
}
}
Here, FetchDataAsync
initiates the data fetch operation and returns immediately. The await
keyword ensures that the method resumes execution once the data is available, without blocking the calling thread.
Using Callbacks
Alternatively, you can use callbacks to handle the result of the asynchronous operation:
public void FetchDataAsync(Action<string> callback)
{
Task.Run(() =>
{
using (var client = new HttpClient())
{
var response = client.GetStringAsync("https://api.example.com/data").Result;
callback(response);
}
});
}
In this approach, FetchDataAsync
accepts a callback function that is invoked once the data is fetched. This method provides flexibility, especially in scenarios where async/await
cannot be used.
Handling Exceptions
Proper error handling is crucial in asynchronous operations. Here’s how you can handle exceptions using async/await
:
public async Task<string> FetchDataAsync()
{
try
{
using (var client = new HttpClient())
{
var response = await client.GetStringAsync("https://api.example.com/data");
return response;
}
}
catch (HttpRequestException ex)
{
// Handle specific HTTP request exceptions
Console.WriteLine($"Request error: {ex.Message}");
return null;
}
catch (Exception ex)
{
// Handle other exceptions
Console.WriteLine($"An error occurred: {ex.Message}");
return null;
}
}
This implementation ensures that any exceptions during the asynchronous operation are caught and handled appropriately, preventing the application from crashing.
Using TaskCompletionSource for Custom Asynchronous Operations
In scenarios where you need more control over the asynchronous operation, TaskCompletionSource
can be utilized:
public Task<string> FetchDataWithTaskCompletionSource()
{
var tcs = new TaskCompletionSource<string>();
Task.Run(() =>
{
try
{
using (var client = new HttpClient())
{
var response = client.GetStringAsync("https://api.example.com/data").Result;
tcs.SetResult(response);
}
}
catch (Exception ex)
{
tcs.SetException(ex);
}
});
return tcs.Task;
}
This approach allows you to manually control the completion of the task, providing flexibility in complex scenarios.
Best Practices for Implementing the AMI Pattern
To effectively implement the AMI pattern in C#, consider the following best practices:
-
Use async/await: Leverage the
async
andawait
keywords for cleaner and more readable asynchronous code. -
Avoid Blocking Calls: Refrain from using
.Result
or.Wait()
on asynchronous methods, as they can lead to deadlocks. -
Handle Exceptions: Always implement proper error handling to catch and manage exceptions in asynchronous operations.
-
Use Cancellation Tokens: Incorporate
CancellationToken
to allow operations to be canceled gracefully. -
Limit Concurrency: Be mindful of the number of concurrent asynchronous operations to prevent resource exhaustion.
-
Test Thoroughly: Asynchronous code can introduce subtle bugs; ensure comprehensive testing, including edge cases and error scenarios.
Different Ways to Implement AMI in C#
C# gives us several flexible approaches to implement the Asynchronous Method Invocation pattern. Depending on the complexity of the task and the level of control you need, you can choose from a few different techniques. Let’s walk through the most common ones and explore how they differ.
1. async
/ await
with Task
This is the most idiomatic and widely used method in modern C#. It’s clean, readable, and integrates well with the .NET runtime.
public async Task<string> ReadFileAsync(string filePath)
{
return await File.ReadAllTextAsync(filePath);
}
The await
keyword tells the compiler to pause method execution until the awaited task completes, without blocking the calling thread.
2. Task Parallel Library (TPL)
The TPL gives more control over task scheduling and execution. You can use Task.Run()
for CPU-bound work.
public Task<int> ComputePrimeAsync(int number)
{
return Task.Run(() => FindNextPrime(number));
}
private int FindNextPrime(int number)
{
while (!IsPrime(++number)) {}
return number;
}
This method is good when you’re offloading computationally expensive work to a background thread.
3. Using Delegates (Classic .NET AMI Pattern)
Before async
/await
came along, AMI was often implemented using delegates with BeginInvoke
and EndInvoke
. This approach is now mostly legacy but helps to understand where the pattern originated.
public delegate int AddDelegate(int a, int b);
public void BeginAddOperation()
{
AddDelegate add = (x, y) => x + y;
add.BeginInvoke(10, 20, new AsyncCallback(AddCompleted), add);
}
private void AddCompleted(IAsyncResult result)
{
var del = (AddDelegate)((AsyncResult)result).AsyncDelegate;
int sum = del.EndInvoke(result);
Console.WriteLine($"Sum is {sum}");
}
This method is rarely used today but still appears in older codebases.
4. TaskCompletionSource (for bridging async behavior)
If you have a legacy or event-based API that doesn’t support async
/await
, you can use TaskCompletionSource
to wrap it.
public Task<string> GetDataWithCallbackAsync()
{
var tcs = new TaskCompletionSource<string>();
SimulateAsyncOperation(result =>
{
if (result != null)
tcs.SetResult(result);
else
tcs.SetException(new Exception("Failed to retrieve data"));
});
return tcs.Task;
}
private void SimulateAsyncOperation(Action<string> callback)
{
Task.Delay(1000).ContinueWith(_ => callback("Hello from async callback!"));
}
This is useful when integrating older libraries into modern async code.
Use Cases for the AMI Pattern
Asynchronous Method Invocation is ideal for certain types of problems. Let’s explore where it’s commonly applied.
1. Web and API Calls
Most real-world APIs involve I/O latency. Using AMI, the application can continue running while it waits for a response.
Example: Calling third-party services like payment gateways, geolocation APIs, or external databases.
public async Task<WeatherInfo> GetWeatherAsync(string city)
{
using var httpClient = new HttpClient();
var json = await httpClient.GetStringAsync($"https://api.weather.com/{city}");
return JsonConvert.DeserializeObject<WeatherInfo>(json);
}
2. File and Disk I/O
Reading or writing large files can block threads if done synchronously. Asynchronous file operations improve app responsiveness.
3. Background Processing
Need to send an email or generate a PDF after a transaction? Offload it to a background task.
public async Task SendEmailAsync(string to, string body)
{
await emailService.SendAsync(to, body);
}
4. UI Applications
In desktop or mobile apps, blocking the UI thread results in a poor user experience. Async programming keeps the interface snappy.
5. Microservices Communication
In distributed systems, services often call one another. AMI helps prevent thread exhaustion when making concurrent requests.
Anti-Patterns to Avoid
Even good tools can be misused. Let’s look at a few common mistakes when using AMI that you’ll want to avoid.
1. Blocking Async Code with .Result
or .Wait()
These force the asynchronous method to run synchronously and can cause deadlocks, especially in UI or ASP.NET contexts.
What to avoid:
var result = GetDataAsync().Result; // BAD: can deadlock
What to do instead:
var result = await GetDataAsync(); // GOOD
2. Fire-and-Forget Without Error Handling
Starting an async operation without tracking or catching exceptions leads to unpredictable behavior.
DoSomethingAsync(); // BAD if not awaited or tracked
If you need fire-and-forget, log exceptions or use background job processing frameworks like Hangfire.
3. Ignoring Cancellation Tokens
Ignoring cancellation can waste resources when an operation is no longer needed (like user navigated away from a page).
public async Task FetchWithCancellationAsync(CancellationToken token)
{
var data = await httpClient.GetStringAsync("https://example.com", token);
}
4. Overusing Async Where It’s Not Needed
Not every method needs to be async. Overuse can add unnecessary complexity.
Advantages of the AMI Pattern
The AMI pattern comes with significant benefits, especially in the right scenarios.
1. Improved Responsiveness
Your applications remain interactive even during long operations. Users aren’t left wondering if the system crashed.
2. Better Resource Utilization
Asynchronous operations free up threads to handle other requests. This is critical in web servers or services under heavy load.
3. Scalability
Apps that use AMI scale better, particularly when handling I/O-bound operations. You can support more users with the same infrastructure.
4. Separation of Concerns
Decoupling the method invocation from result processing leads to more modular and testable code.
Disadvantages of the AMI Pattern
Despite its benefits, AMI isn’t without trade-offs. It’s important to know the downsides so you can plan accordingly.
1. Increased Complexity
Async code is harder to debug and trace. Stack traces become less intuitive, and error propagation can be tricky.
2. Difficult Error Handling
Managing exceptions across asynchronous boundaries takes discipline. Missing even one try/catch
can cause silent failures.
3. Context Switching Overhead
Async introduces context switches between threads. While generally efficient, excessive context switching can hurt performance in CPU-bound workloads.
4. Compatibility Issues
Older libraries or frameworks may not support async patterns, requiring wrappers or alternative solutions.
Conclusion
The Asynchronous Method Invocation design pattern is a foundational concept for building modern, responsive, and scalable applications. Whether you’re building a real-time dashboard, a microservices API, or a mobile app, knowing how to execute operations without blocking the main thread is vital.
C# gives you rich tools—from async/await
to TaskCompletionSource
—to implement AMI effectively. But as with any design pattern, it should be applied thoughtfully. Understand your use case, weigh the trade-offs, and avoid common pitfalls.
By mastering AMI, you’ll write cleaner, faster, and more user-friendly software. And in the end, isn’t that what every architect aims for?
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →