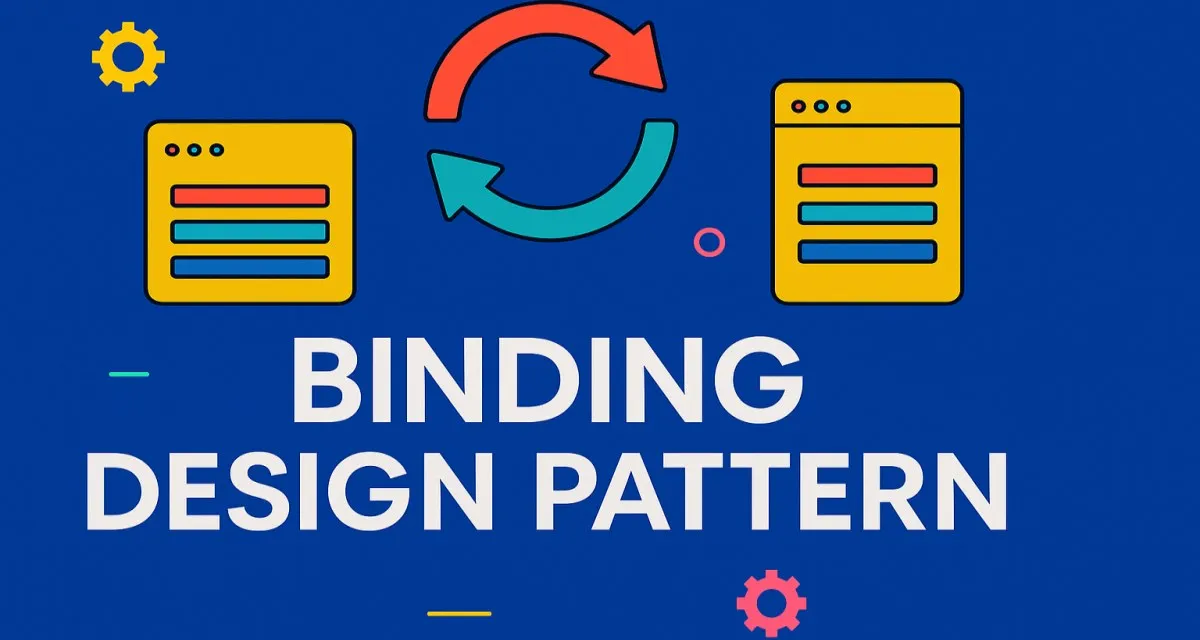
Mastering the Binding Design Pattern in C#: A Comprehensive Guide for Software Architects
Have you ever built software that feels tangled and rigid, where making changes resembles pulling at threads that unravel the entire garment? If you’re nodding right now, it’s time we introduce you to the Binding design pattern. Like a good playlist syncing effortlessly across your devices, the Binding pattern ensures your application’s data and UI stay in harmony, reflecting changes automatically and elegantly.
In this comprehensive guide, we’ll demystify the Binding design pattern. You’ll learn exactly what it is, how it evolved, when to use it, and—most importantly—how to implement it effectively in C#.
Introduction to the Binding Design Pattern
What Exactly Is the Binding Pattern?
The Binding pattern is a behavioral design pattern that creates a seamless connection between an application’s data (model) and its user interface (view). When data changes, your UI reacts immediately—no manual updates needed. Think of it as tying a knot between your data and your UI; tugging one end instantly pulls the other. Simple, right?
But why is this crucial?
Imagine building a music app. When your user skips to the next song, the UI needs to reflect the new track title instantly. Without Binding, you’d manually refresh the interface every time your data changes—like having to rewind your favorite cassette every time you switch songs. Painful.
Historical Context and Origin
The Binding pattern didn’t pop out of nowhere. It evolved from concepts introduced by earlier frameworks, particularly during the late ’90s and early 2000s, when desktop applications grew complex and dynamic. Frameworks like Microsoft’s WPF (Windows Presentation Foundation), introduced around 2006, popularized the Binding concept by making UI responsiveness easier and code more maintainable.
Today, this concept is foundational not only in desktop applications but also in web development frameworks like Angular, React, and Vue.js. Yet, in C# and the .NET ecosystem, Binding remains a cornerstone for creating responsive and maintainable applications.
Position Within Behavioral Design Patterns
Behavioral design patterns manage interactions and responsibilities among objects. The Binding pattern specifically fits here because it controls how data updates propagate across your application components. Unlike structural patterns (which define object composition) or creational patterns (which handle object creation), behavioral patterns orchestrate communication. Binding excels at precisely this—coordinating interactions between your data and UI seamlessly.
Core Principles of the Binding Design Pattern
To fully leverage Binding, let’s grasp its core principles first:
1. Automatic Synchronization
At its heart, Binding ensures automatic synchronization between a data source (model) and a target (view). Change one, and the other updates instantly.
2. Loose Coupling
The Binding pattern separates the UI from the data logic, reducing dependencies and making your code easier to manage and scale.
3. Observer Mechanism
Binding typically relies on an observer-like mechanism. The UI observes data changes and reacts automatically, promoting reactivity.
4. Reduced Boilerplate
By handling updates automatically, Binding drastically reduces repetitive, boilerplate code. Developers can thus focus more on core logic.
Key Components of the Binding Pattern
Understanding the Binding pattern involves breaking it down into three primary components:
1. Source
The source is the data or business logic (often your model or ViewModel in MVVM architecture). Changes here trigger updates.
2. Binding Mechanism
This intermediary connects source and target, listening for changes and propagating updates.
3. Target
Typically your UI elements or controls, which display or edit data.
Think of these components as a communication channel:
- Source: Your friend texting you song recommendations.
- Binding Mechanism: Your messaging app sending notifications.
- Target: Your phone’s notification display.
When Should You Use the Binding Pattern?
Not every situation calls for Binding. However, in scenarios where data constantly changes or needs immediate reflection in the UI, Binding becomes invaluable.
Appropriate Scenarios for Using Binding:
- Real-time Updates: Applications requiring instant UI refresh, like stock market apps, chat applications, or dashboards.
- Complex Forms and Data Entry: Applications where users enter complex data needing immediate validation and response.
- Interactive and Reactive Interfaces: Games or apps where immediate feedback enhances user experience.
Practical Business Cases:
- Financial Apps: Real-time portfolio updates as stock prices fluctuate.
- Healthcare Systems: Instant patient information updates across multiple views.
- E-commerce Platforms: Immediate updates to inventory or shopping carts.
Technical Contexts Where Binding Excels:
- MVVM Applications: WPF, Xamarin, and MAUI applications heavily rely on Binding to manage data/UI interaction effectively.
- Real-time Dashboards: SignalR-powered dashboards or real-time monitoring tools.
- Collaborative Tools: Systems like Trello, Slack, or Teams, where UI updates immediately reflect underlying data changes.
Implementation Approaches in C# (With Detailed Examples)
Let’s explore practical Binding implementations using C#. We’ll cover essential methods, including event-based Binding and Binding using the MVVM pattern.
Example 1: Simple Event-Based Binding
The simplest form of Binding is event-driven. Here’s how you can set it up:
Step-by-step C# example:
First, define a data model:
public class Song
{
private string _title;
public string Title
{
get => _title;
set
{
if (_title != value)
{
_title = value;
TitleChanged?.Invoke(this, EventArgs.Empty);
}
}
}
public event EventHandler TitleChanged;
}
Next, bind this model to your UI component:
public partial class MusicPlayer : Form
{
private Song currentSong;
public MusicPlayer()
{
InitializeComponent();
currentSong = new Song();
currentSong.TitleChanged += OnTitleChanged;
}
private void OnTitleChanged(object sender, EventArgs e)
{
titleLabel.Text = currentSong.Title;
}
}
Why it works:
- When the
Song
title changes, it triggersTitleChanged
. - Your UI (
MusicPlayer
) subscribes to that event, updating the UI automatically.
Example 2: Advanced MVVM-Based Binding (Recommended Approach)
MVVM (Model-View-ViewModel) provides a cleaner, decoupled architecture ideal for Binding.
Implementing MVVM Binding in WPF:
Step 1: Define ViewModel with INotifyPropertyChanged
public class SongViewModel : INotifyPropertyChanged
{
private string _title;
public string Title
{
get => _title;
set
{
if (_title != value)
{
_title = value;
OnPropertyChanged(nameof(Title));
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
Step 2: Bind ViewModel to View (XAML)
<Window x:Class="MusicApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Music App" Height="200" Width="300">
<StackPanel DataContext="{Binding SongViewModel}">
<TextBox Text="{Binding Title, UpdateSourceTrigger=PropertyChanged}" />
<TextBlock Text="{Binding Title}" FontSize="16"/>
</StackPanel>
</Window>
Step 3: Set DataContext in Code-Behind
public partial class MainWindow : Window
{
public SongViewModel SongViewModel { get; set; }
public MainWindow()
{
InitializeComponent();
SongViewModel = new SongViewModel { Title = "No song playing" };
DataContext = this;
}
}
Why this is powerful:
- Decouples UI from business logic.
- Any change in
SongViewModel.Title
updates UI elements bound to it automatically. - Cleaner architecture that’s maintainable and testable.
Different Ways to Implement Binding in Modern C#
As we’ve seen, the Binding pattern dramatically simplifies keeping your data and UI synchronized. But what about modern, feature-rich implementations using the latest C# and .NET frameworks? Let’s explore a few contemporary approaches.
1. Using the MVVM Toolkit with .NET MAUI or WPF (.NET 8+)
The CommunityToolkit.Mvvm has revolutionized MVVM-based Binding by eliminating repetitive boilerplate through attributes and code generators. Let’s see how easily you can implement Binding with this powerful toolkit.
Step 1: Install MVVM Toolkit
Use NuGet package manager:
Install-Package CommunityToolkit.Mvvm
Step 2: Create a ViewModel with ObservableProperty
Here’s your modern, succinct ViewModel:
using CommunityToolkit.Mvvm.ComponentModel;
public partial class SongViewModel : ObservableObject
{
[ObservableProperty]
private string title = "Nothing playing yet";
}
Why does this matter?
Notice something special? No more manual calls to OnPropertyChanged
. The [ObservableProperty]
attribute generates everything automatically, keeping your code neat and clean.
Step 3: Bind the ViewModel in your XAML (.NET MAUI Example)
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MusicApp.Views.MainPage">
<VerticalStackLayout BindingContext="{Binding SongViewModel}">
<Entry Text="{Binding Title}" Placeholder="Enter song title" />
<Label Text="{Binding Title}" FontSize="20"/>
</VerticalStackLayout>
</ContentPage>
Step 4: Set the ViewModel in your Code-behind
public partial class MainPage : ContentPage
{
public SongViewModel SongViewModel { get; }
public MainPage()
{
InitializeComponent();
SongViewModel = new SongViewModel();
BindingContext = this;
}
}
Why choose this method?
- Cleaner, less error-prone code
- Easier maintenance
- Ideal for large, scalable apps
Real World Use Cases of Binding
Still wondering if Binding is practical for real-world scenarios? Let’s explore some prominent cases:
1. Real-time Financial Trading Platforms
In financial apps, stock prices constantly fluctuate. Binding instantly reflects these changes, providing traders immediate insight.
2. Collaborative Editing Tools
Applications like Google Docs or Microsoft Teams utilize Binding extensively to synchronize text and cursor positions instantly among users.
3. Health Monitoring Systems
Vital health data like heart rate, blood pressure, or medication dosage require instant UI updates, something Binding accomplishes effortlessly.
Common Anti-patterns and Pitfalls
Binding seems simple, right? But developers can still fall into common traps:
1. Overuse of Binding (Binding Everything)
Binding isn’t magic; it’s a tool. Overusing Binding on trivial data or rarely changing values complicates maintenance unnecessarily. Ask yourself: Does this piece of data genuinely need real-time synchronization?
2. Ignoring Performance
Binding large collections directly to your UI without virtualization can lead to performance issues. Always use techniques like virtualization (ItemsControl
with virtualized panels in WPF or CollectionView in MAUI).
3. Mixing UI Logic in ViewModels
Your ViewModel should remain UI-independent. Avoid directly referencing UI elements or visual state. Keep the responsibilities separate to maintain clarity and testability.
Advantages and Benefits of the Binding Pattern
What do you get from implementing Binding effectively?
- Automatic UI Synchronization: Changes reflect instantly without manual updates.
- Reduced Boilerplate: Eliminates repetitive code, streamlining development.
- Maintainable Codebase: Easier debugging and updating due to clear separation of concerns.
- Improved Scalability: Makes expanding your application easier without breaking existing functionality.
- Enhanced User Experience: Instant updates make interfaces feel responsive and intuitive.
Disadvantages and Limitations of the Binding Pattern
But Binding isn’t flawless. Consider these trade-offs:
- Steeper Learning Curve: Initially challenging to understand, especially for beginners.
- Debugging Complexity: Identifying Binding issues or failures can be tricky.
- Potential Performance Penalties: Poorly designed Bindings, especially complex collections, can degrade UI performance.
- Overhead for Simple Apps: Small, static apps might not benefit significantly, making Binding unnecessary overhead.
Testing Pattern Implementations
Testing Binding implementations is critical for robust applications. But how do you effectively test Bindings?
1. Unit Testing ViewModels
Focus heavily on unit testing ViewModels. Because ViewModels hold your logic, they’re the most critical point to test.
[TestMethod]
public void ChangingSongTitleRaisesPropertyChanged()
{
var songViewModel = new SongViewModel();
var triggered = false;
songViewModel.PropertyChanged += (s, e) =>
{
if (e.PropertyName == nameof(songViewModel.Title))
triggered = true;
};
songViewModel.Title = "New Song";
Assert.IsTrue(triggered, "Title PropertyChanged event should have fired.");
}
2. Integration/UI Testing
Use integration tests to ensure your UI updates correctly:
- Automation tools: WinAppDriver, Selenium (for web), Appium (for mobile/MAUI).
- Ensure UI reflects changes immediately.
3. Performance Testing
Monitor your app’s performance to detect and resolve slow Bindings early using profiling tools like Visual Studio’s Diagnostics Tools.
Conclusion and Best Practices for Implementing Binding in C#
As we wrap up, let’s revisit key insights and best practices:
Best Practices to Remember:
- Keep ViewModels Clean: Logic, not UI details, should reside here.
- Leverage Modern Toolkits: The MVVM Toolkit significantly simplifies your implementation.
- Avoid Over-Binding: Only use Binding when it genuinely adds value.
- Focus on Maintainability: Ensure your Binding implementation remains clean, understandable, and maintainable over time.
- Proactively Test: Prioritize unit and integration tests to catch problems early.
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →