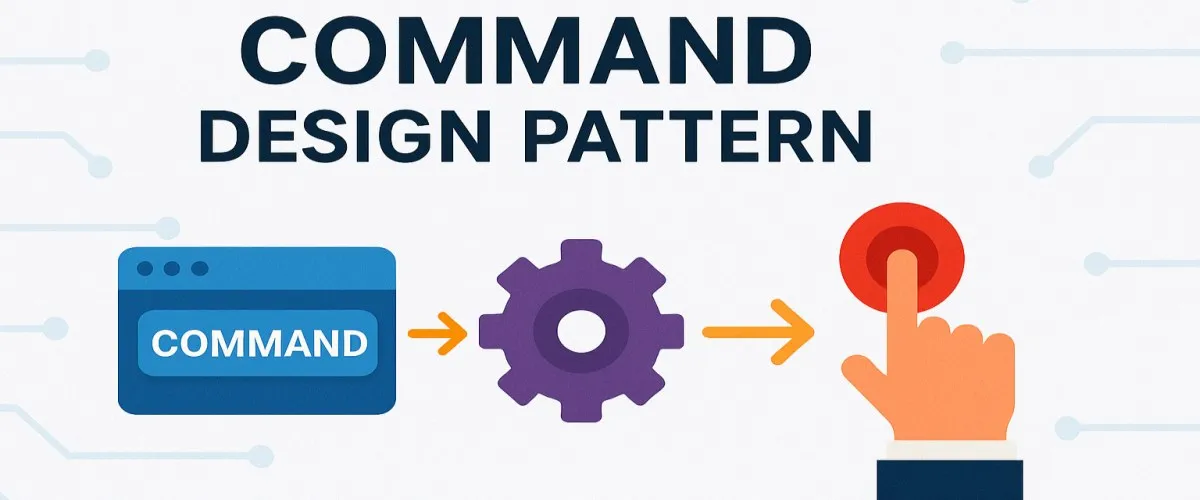
Mastering the Command Design Pattern in C#: A Fun and Practical Guide for Software Architects
- Sudhir mangla
- Behavioral design patterns
- 17 Apr, 2025
📚 Introduction
Hey there, software architect! Have you ever felt like you’re constantly juggling flaming torches when managing requests in a large application? You’re adding commands here, removing commands there, trying desperately to keep everything organized—but it’s messy, right? Well, guess what? There’s a superhero pattern designed precisely for this chaos: the Command Pattern.
Today, we’ll demystify the Command Design Pattern with C# examples that even your coffee machine could follow (if it spoke code!). Buckle up—you’re about to become an expert!
🚀 What Exactly is the Command Pattern?
Let’s start from the basics—What exactly is this Command Design Pattern thingy?
The Command Pattern is a behavioral design pattern that turns a request or action into a standalone object. These standalone objects encapsulate all information required to execute or even undo actions. Imagine if your TV remote didn’t directly change channels, but instead, each button press created a small “command” that could be executed, logged, or even undone later. That’s exactly what the Command Pattern does.
Think of commands as mini-robots carrying out your orders. They encapsulate what you want to do, how you want to do it, and even how to reverse it later.
đź“– Principles of the Command Pattern
Let’s quickly decode the foundational principles behind this pattern:
-
Encapsulation of Requests:
Wrap requests into command objects, separating them from the code that executes them. -
Parameterization of Clients with Commands:
Commands can be dynamically changed, meaning clients aren’t hardcoded to specific actions. It’s like switching batteries rather than wiring new circuits every time you need a change. -
Support for Undo/Redo Operations:
Because commands store state, you can easily reverse actions, making your app feel like magic! -
Decoupling Senders from Receivers:
The sender (like your UI or controller) doesn’t directly depend on who’s executing the command. It just says, “Hey, here’s what I want done. Figure it out.”
⏳ When Should You Use the Command Pattern?
The Command Pattern shines when you’re dealing with situations like:
- Undoable Operations: Need a reliable “undo” button? Command Pattern’s got your back.
- Transactional Systems: Commands can encapsulate transactions, making your database operations safer.
- Scheduling Tasks: Think task queues or scheduled jobs, where you don’t execute immediately.
- Macro Operations: Want to bundle several tasks into one command? Easy!
- Event-driven UIs: Button clicks, menu items—basically any user-triggered action.
If any of these sound familiar, congrats—you’ve found the perfect pattern!
đź”§ Key Components of the Command Pattern
Before diving into code, let’s understand the key players involved:
- Command Interface: Defines the method (
Execute()
) that concrete commands must implement. - Concrete Command: Implements the Command interface; knows about the receiver and invokes a method on it.
- Invoker: Calls commands without knowing their details—think of it as your TV remote control.
- Receiver: Knows how to perform the actual operations.
- Client: Creates command objects, assigns receivers, and hands commands to invokers.
Here’s a simpler analogy:
Invoker = Waiter
Command = Order ticket
Receiver = Chef
The waiter doesn’t know how to cook but hands your order to the chef who executes the cooking. Makes sense?
đź’» Implementation with Detailed C# Example
Let’s roll up our sleeves and dive into a practical, complete example in C# using a common scenario: a Text Editor that supports undo functionality.
🎯 Scenario:
You’re building a text editor. Users should be able to type, delete text, and undo operations easily. Sounds familiar, huh?
🎨 Step-by-step Implementation:
Step 1: Define the Command Interface
public interface ICommand
{
void Execute();
void Unexecute();
}
Step 2: Create the Receiver (TextEditor)
The receiver performs the actual text operations:
public class TextEditor
{
public string Text { get; private set; } = "";
public void AddText(string text)
{
Text += text;
Console.WriteLine($"Text after adding: {Text}");
}
public void DeleteText(int length)
{
if (length > Text.Length) length = Text.Length;
Text = Text.Substring(0, Text.Length - length);
Console.WriteLine($"Text after deleting: {Text}");
}
}
Step 3: Concrete Commands
Let’s create concrete commands for adding and deleting text:
// AddText Command
public class AddTextCommand : ICommand
{
private readonly TextEditor _editor;
private readonly string _textToAdd;
public AddTextCommand(TextEditor editor, string textToAdd)
{
_editor = editor;
_textToAdd = textToAdd;
}
public void Execute()
{
_editor.AddText(_textToAdd);
}
public void Unexecute()
{
_editor.DeleteText(_textToAdd.Length);
}
}
// DeleteText Command
public class DeleteTextCommand : ICommand
{
private readonly TextEditor _editor;
private readonly int _lengthToDelete;
private string _deletedText;
public DeleteTextCommand(TextEditor editor, int lengthToDelete)
{
_editor = editor;
_lengthToDelete = lengthToDelete;
}
public void Execute()
{
_deletedText = _editor.Text.Substring(_editor.Text.Length - _lengthToDelete, _lengthToDelete);
_editor.DeleteText(_lengthToDelete);
}
public void Unexecute()
{
_editor.AddText(_deletedText);
}
}
Step 4: Create the Invoker (CommandManager)
This is your remote control that executes commands and keeps history for undo operations:
public class CommandManager
{
private readonly Stack<ICommand> _history = new Stack<ICommand>();
public void ExecuteCommand(ICommand command)
{
command.Execute();
_history.Push(command);
}
public void Undo()
{
if (_history.Any())
{
ICommand command = _history.Pop();
command.Unexecute();
}
}
}
Step 5: Putting It All Together (Client Code)
Here’s your client code running everything smoothly:
class Program
{
static void Main()
{
var editor = new TextEditor();
var manager = new CommandManager();
// Add some text
manager.ExecuteCommand(new AddTextCommand(editor, "Hello "));
manager.ExecuteCommand(new AddTextCommand(editor, "World!"));
// Delete some text
manager.ExecuteCommand(new DeleteTextCommand(editor, 6));
// Undo last delete operation
manager.Undo();
// Undo adding "World!"
manager.Undo();
Console.WriteLine($"Final Text: {editor.Text}");
}
}
🚀 Run the code and you’ll see:
Text after adding: Hello
Text after adding: Hello World!
Text after deleting: Hello
Text after adding: Hello World!
Text after deleting: Hello
Final Text: Hello
Congrats! You’ve now successfully implemented the Command Pattern complete with undo functionality!
🎯 Takeaways (TL;DR):
- Commands encapsulate operations as objects.
- Invokers call commands without needing details about execution.
- Receivers perform actual operations.
- Undoable actions, macro commands, and transaction handling are easy.
- Your architecture stays clean, maintainable, and extensible.
🌟 Different Ways to Implement the Command Pattern (With C# Examples)
Great job getting through the basics! Now, let’s kick things up a notch and see some cool variations on implementing the Command Pattern. Think of these as spices—same dish, different flavors!
📌 Method 1: Generic Command (Reusable Command Class)
If your commands are similar enough, you can use a single, reusable generic command:
public class GenericCommand : ICommand
{
private readonly Action _executeAction;
private readonly Action _unexecuteAction;
public GenericCommand(Action executeAction, Action unexecuteAction)
{
_executeAction = executeAction;
_unexecuteAction = unexecuteAction;
}
public void Execute() => _executeAction();
public void Unexecute() => _unexecuteAction();
}
Client code looks neat and tidy:
var editor = new TextEditor();
var manager = new CommandManager();
manager.ExecuteCommand(new GenericCommand(
() => editor.AddText("Hello, Generic World!"),
() => editor.DeleteText("Hello, Generic World!".Length)));
manager.Undo();
📌 Method 2: Command with Lambda Expressions
Want brevity and beauty? Lambdas have your back:
var editor = new TextEditor();
var manager = new CommandManager();
manager.ExecuteCommand(new GenericCommand(
executeAction: () => editor.AddText("Lambda Rocks!"),
unexecuteAction: () => editor.DeleteText("Lambda Rocks!".Length)));
📌 Method 3: Command Factory Pattern (Dynamic Command Creation)
If your app grows complex, consider using factories to simplify command creation:
public class CommandFactory
{
public static ICommand CreateAddTextCommand(TextEditor editor, string text)
{
return new AddTextCommand(editor, text);
}
public static ICommand CreateDeleteTextCommand(TextEditor editor, int length)
{
return new DeleteTextCommand(editor, length);
}
}
// Usage:
manager.ExecuteCommand(CommandFactory.CreateAddTextCommand(editor, "Factory Created!"));
Think of factories as vending machines—they dispense commands ready-to-go.
🔸 Command Queue for Batch Execution
Commands can be queued for later batch execution. Imagine a “macro” or “batch” command like this:
public class BatchCommand : ICommand
{
private readonly List<ICommand> _commands = new List<ICommand>();
public void AddCommand(ICommand command) => _commands.Add(command);
public void Execute()
{
foreach (var cmd in _commands)
cmd.Execute();
}
public void Unexecute()
{
foreach (var cmd in Enumerable.Reverse(_commands))
cmd.Unexecute();
}
}
This approach lets you bundle several commands into one neat package. Think “playlist,” but for commands!
🎯 Real-World Use Cases of the Command Pattern
Time for some practical examples where this superhero truly shines:
-
Undo/Redo in Editors:
Text editors (think Visual Studio) heavily rely on the Command Pattern for undo operations. -
Transaction Management:
Financial software and database operations often encapsulate transactions into commands for rollback capabilities. -
Task Scheduling and Queuing:
Batch-processing systems or cloud jobs use commands to schedule, delay, and track tasks. -
Game Development:
Games frequently use commands for player actions—pause, move, attack, or undo moves. -
Remote Procedure Calls (RPC):
Web apps and APIs use commands to encapsulate and serialize actions across network boundaries.
đźš« Anti-Patterns to Avoid with the Command Pattern
Let’s talk traps—common mistakes you want to dodge:
Now, before you dive too deeply, here are a few potholes you definitely want to steer clear of:
-
Overloading Commands with Responsibilities:
- Keep commands simple—don’t make them manage database connections, validation, and business logic all at once. Keep it lean and mean.
-
Ignoring Undo/Redo Consistency:
- If you offer undo, always make sure it truly reverses the action. Half-undoing operations leads to chaos (and unhappy users).
-
Creating Commands for Every Single Method:
- If every method becomes a command, you’ll drown in tiny classes. Identify operations where encapsulation and undoability matter.
-
Coupling Invoker and Receiver Tightly:
- Commands are supposed to decouple invokers and receivers. Tight coupling defeats the whole purpose.
🎖️ Advantages of the Command Pattern
The Command Pattern brings some pretty compelling benefits:
-
Decoupling & Flexibility:
- Changes to commands won’t ripple through your app.
-
Support for Undo/Redo:
- Built-in support for undo operations makes your app feel intuitive and forgiving.
-
Simplified Extensibility:
- Adding new commands is as easy as making a sandwich—no structural upheaval required.
-
Improved Maintainability:
- Clean separation of concerns means easy maintenance and debugging.
⚠️ Disadvantages of the Command Pattern
Every superhero has a weakness. Command Pattern isn’t exempt:
-
Increased Complexity:
- Initially, creating all those extra classes might feel cumbersome, especially in small projects.
-
Too Many Small Classes:
- Sometimes commands become tiny “one-liners,” leading to class bloat if not managed wisely.
-
Difficulty in Debugging:
- Debugging can get tricky with indirect calls and stacked commands.
đźš« Anti-patterns (Important enough to Repeat!)
Yup, you guessed it—these are important enough to stress again (because mistakes here hurt a lot):
-
Over-complicating Simple Actions:
- Don’t commandify trivial methods. Use commands when you need real encapsulation, undo capability, or delayed execution.
-
State Management Neglect:
- Ignoring or improperly managing command state ruins undo functionality. Keep track of states diligently.
🏅 Conclusion: Command Your Code Like a Pro!
Wow—you’ve come a long way! You’re now officially armed with enough knowledge to master and leverage the Command Design Pattern effectively in C#.
The Command Pattern is like a trusty Swiss Army knife in your architectural toolbox. Whether you’re creating flexible UIs, batch operations, undoable transactions, or even building a game, commands will make your life smoother.
Remember, patterns aren’t rigid rules—they’re helpful guidelines. Your job as a software architect isn’t just to implement patterns blindly; it’s to know when and how to use them strategically. The Command Pattern is no exception.
So, what are you waiting for? Go command your architecture, boost your application’s maintainability, and impress your colleagues with your newfound architectural prowess!