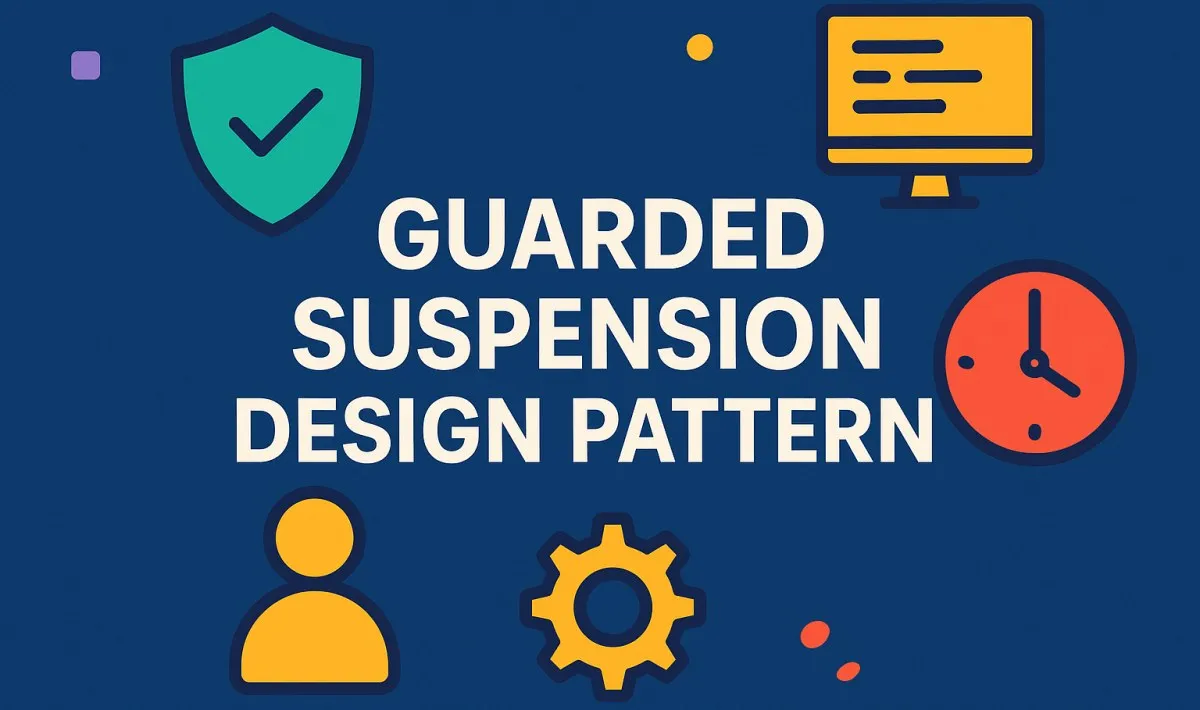
Guarded Suspension Design Pattern: A Deep Dive for Software Architects
Introduction to the Pattern
Definition and Core Concept
The Guarded Suspension design pattern is a concurrency control mechanism that ensures a thread waits for a specific condition to become true before proceeding with an operation. This pattern is particularly useful in scenarios where operations depend on certain preconditions, such as the availability of resources or the readiness of data. By suspending the execution of a thread until the required condition is met, the pattern helps in maintaining data consistency and preventing race conditions.
Historical Context and Origin
The concept of Guarded Suspension emerged from the need to manage concurrent operations in multi-threaded environments. It was popularized by Doug Lea in his seminal work, “Concurrent Programming in Java,” where he detailed various concurrency patterns to handle complex synchronization issues. The pattern has since been adopted across various programming languages and platforms, including C#, to address similar concurrency challenges.
Position Within Behavioral Design Patterns
Guarded Suspension falls under the category of behavioral design patterns, which are concerned with the interaction and responsibility of objects. Specifically, it is a concurrency pattern that deals with the coordination and communication between threads, ensuring that operations are executed in a controlled and predictable manner.
Core Principles
At its core, the Guarded Suspension pattern operates on the following principles:
-
Guard Condition: A boolean expression that determines whether a thread can proceed with its operation. If the condition is false, the thread must wait.
-
Suspension Mechanism: When the guard condition is not met, the thread suspends its execution, typically by waiting on a monitor or condition variable.
-
Notification Mechanism: Once the guard condition becomes true, another thread notifies the waiting thread(s) to resume execution.
These principles ensure that threads only proceed when it’s safe to do so, thereby maintaining the integrity of shared resources.
Key Components
Implementing the Guarded Suspension pattern involves several key components:
-
Lock Object: A synchronization primitive (e.g.,
lock
in C#) that ensures mutual exclusion when accessing shared resources. -
Condition Variable: An object that allows threads to wait for certain conditions to become true. In C#, this can be implemented using
Monitor.Wait
andMonitor.Pulse
. -
Guard Condition: A method or expression that evaluates whether the precondition for proceeding is met.
-
Wait and Notify Mechanisms: Methods that allow threads to wait for a condition (
Wait
) and to notify waiting threads when the condition changes (Pulse
orPulseAll
).
When to Use
Appropriate Scenarios
The Guarded Suspension pattern is particularly useful in scenarios where operations depend on certain conditions being met. Examples include:
-
Producer-Consumer Queues: Where consumers must wait for producers to add items to the queue before processing.
-
Resource Pools: Where threads must wait for resources (e.g., database connections) to become available.
-
Event Handling Systems: Where threads wait for specific events or signals before proceeding.
Business Cases
In business applications, the pattern is applicable in:
-
Order Processing Systems: Where orders must wait for inventory to be restocked before fulfillment.
-
Booking Systems: Where reservations must wait for cancellations or availability before confirmation.
-
Workflow Management: Where tasks must wait for prerequisites to be completed before execution.
Technical Contexts Where This Pattern Shines
From a technical standpoint, the Guarded Suspension pattern is advantageous in:
-
Multi-threaded Applications: To prevent race conditions and ensure thread-safe operations.
-
Asynchronous Programming: To manage dependencies between asynchronous tasks.
-
Real-time Systems: To coordinate timing-sensitive operations.
Implementation Approaches (with Detailed C# Examples)
In C#, the Guarded Suspension pattern can be implemented using the Monitor
class, which provides mechanisms for synchronizing access to objects.
Example: Producer-Consumer Queue
Let’s consider a simple producer-consumer scenario where the consumer waits for items to be available in the queue.
using System;
using System.Collections.Generic;
using System.Threading;
public class GuardedQueue<T>
{
private readonly Queue<T> _queue = new Queue<T>();
private readonly object _lock = new object();
public void Enqueue(T item)
{
lock (_lock)
{
_queue.Enqueue(item);
Monitor.Pulse(_lock); // Notify waiting threads
}
}
public T Dequeue()
{
lock (_lock)
{
while (_queue.Count == 0)
{
Monitor.Wait(_lock); // Wait until an item is available
}
return _queue.Dequeue();
}
}
}
In this example, the Dequeue
method waits for the queue to have items before proceeding, ensuring that consumers only process available data.
Example: Resource Pool
Consider a scenario where threads need to acquire a limited resource, such as a database connection.
using System;
using System.Collections.Generic;
using System.Threading;
public class ResourcePool
{
private readonly Queue<string> _resources = new Queue<string>();
private readonly object _lock = new object();
public ResourcePool(IEnumerable<string> initialResources)
{
foreach (var resource in initialResources)
{
_resources.Enqueue(resource);
}
}
public string AcquireResource()
{
lock (_lock)
{
while (_resources.Count == 0)
{
Monitor.Wait(_lock); // Wait until a resource is available
}
return _resources.Dequeue();
}
}
public void ReleaseResource(string resource)
{
lock (_lock)
{
_resources.Enqueue(resource);
Monitor.Pulse(_lock); // Notify waiting threads
}
}
}
Here, threads attempting to acquire a resource will wait if none are available, and will be notified when a resource is released back into the pool.
Example: Event Waiter
In situations where a thread must wait for a specific event to occur, the pattern can be applied as follows:
using System;
using System.Threading;
public class EventWaiter
{
private bool _eventOccurred = false;
private readonly object _lock = new object();
public void WaitForEvent()
{
lock (_lock)
{
while (!_eventOccurred)
{
Monitor.Wait(_lock); // Wait for the event
}
_eventOccurred = false; // Reset for future use
}
}
public void SignalEvent()
{
lock (_lock)
{
_eventOccurred = true;
Monitor.PulseAll(_lock); // Notify all waiting threads
}
}
}
This implementation allows threads to wait for a signal indicating that a particular event has occurred.
Different Ways to Implement Guarded Suspension (Using Modern C# Features)
The classic implementation using Monitor.Wait
and Monitor.Pulse
works well, but modern C# offers more expressive and robust ways to handle concurrency, making your code cleaner and often more resilient. Let’s walk through some contemporary approaches using newer features in the .NET ecosystem.
1. Using SemaphoreSlim
SemaphoreSlim
provides a lightweight alternative to Monitor
for signaling. It’s especially useful when the guarded condition is simply about availability.
public class SemaphoreGuardedQueue<T>
{
private readonly Queue<T> _queue = new();
private readonly SemaphoreSlim _semaphore = new(0);
public void Enqueue(T item)
{
lock (_queue)
{
_queue.Enqueue(item);
}
_semaphore.Release();
}
public async Task<T> DequeueAsync()
{
await _semaphore.WaitAsync();
lock (_queue)
{
return _queue.Dequeue();
}
}
}
This version is ideal when you want to incorporate async/await
for non-blocking operations. It keeps your threads responsive, especially in UI or web apps.
2. Using Channel<T>
from System.Threading.Channels
Introduced in .NET Core, Channel<T>
is designed for producer-consumer scenarios and internally uses guarded suspension patterns.
public class ChannelQueue<T>
{
private readonly Channel<T> _channel = Channel.CreateUnbounded<T>();
public async Task EnqueueAsync(T item)
{
await _channel.Writer.WriteAsync(item);
}
public async Task<T> DequeueAsync()
{
return await _channel.Reader.ReadAsync();
}
}
If you don’t want to reinvent the wheel, Channel<T>
is the safest and most performant way to implement a guarded suspension pipeline today.
3. Using AsyncManualResetEvent
(Custom Implementation)
For custom synchronization requirements, a resettable async event is handy.
public class AsyncManualResetEvent
{
private volatile TaskCompletionSource<bool> _tcs = new(TaskCreationOptions.RunContinuationsAsynchronously);
public Task WaitAsync() => _tcs.Task;
public void Set()
{
var tcs = _tcs;
Task.Factory.StartNew(() => tcs.TrySetResult(true),
CancellationToken.None, TaskCreationOptions.PreferFairness, TaskScheduler.Default);
}
public void Reset()
{
if (_tcs.Task.IsCompleted)
{
_tcs = new TaskCompletionSource<bool>(TaskCreationOptions.RunContinuationsAsynchronously);
}
}
}
This approach gives you more control over the lifecycle of a “waitable” condition and works well for complex workflows.
Real-World Use Cases
Theory is great, but what really drives value is how you apply a pattern in the real world. So, where does Guarded Suspension show up in production systems?
1. Message Brokers and Event Buses
Imagine a microservices architecture where services produce and consume messages. Consumers often wait for specific types of events to arrive before acting. Guarded Suspension prevents premature execution until the expected event lands.
2. Job Scheduling and Task Queues
In systems like background workers, jobs can’t start unless certain conditions (e.g., dependencies, priority level, resource availability) are satisfied. Rather than busy-waiting or polling, threads can suspend until everything is ready.
3. Inventory and Booking Systems
Let’s say you’re booking tickets. If the desired seat isn’t available, the system can pause processing and resume once cancellations free up space. This is where the guard condition revolves around availability.
4. Concurrent Data Aggregators
In analytics or ETL systems, downstream operations may wait for upstream data feeds. The suspension ensures downstream computations only kick in when there’s something valid to work with.
Common Anti-patterns and Pitfalls
Even the best patterns can backfire if misused. Here are some common traps to avoid when implementing Guarded Suspension.
1. Spurious Wakeups
Threads can wake up even if the condition hasn’t changed. Always re-check the condition in a loop after waking.
lock (_lock)
{
while (!condition)
Monitor.Wait(_lock);
}
Failing to use a loop around Monitor.Wait
is a recipe for subtle bugs.
2. Missing Notification
If you forget to call Monitor.Pulse
or Semaphore.Release
, waiting threads could be stuck forever. Always ensure your modifying code includes a corresponding notify step.
3. Deadlocks from Nested Locks
Avoid taking other locks inside the guarded block. This can easily lead to deadlocks if not managed carefully.
4. Overengineering
Sometimes developers use Guarded Suspension when a simple blocking collection or a well-designed queue would suffice. Don’t force it if the problem doesn’t warrant the complexity.
Advantages and Benefits
So why even bother with Guarded Suspension when there are so many other concurrency models? Here’s what makes it valuable.
1. Predictable Thread Coordination
Threads proceed only when they should. This helps eliminate race conditions and unintended side effects.
2. Improved System Stability
Avoids unnecessary resource contention and failure scenarios by waiting until the system is ready.
3. Cleaner Resource Utilization
Rather than spinning and wasting CPU, threads sleep when they have nothing to do.
4. Increased Modularity
The pattern separates condition-checking logic from the business operation itself. This makes components easier to test and reason about.
Disadvantages and Limitations
No pattern is flawless. Understanding the downsides is key to knowing when to look elsewhere.
1. Complexity in Debugging
Threads that “just wait” without clear logging or diagnostics can be hard to trace, especially under heavy load.
2. Performance Bottlenecks
Improper use can serialize access to shared resources, degrading performance instead of improving it.
3. Harder to Reason About in Async Contexts
While possible, combining guarded suspension with async/await
requires careful handling of task completion and continuation logic.
4. Thread Starvation Risk
If certain conditions are met too infrequently, some threads may be waiting longer than needed unless managed correctly.
Testing Pattern Implementations
Concurrency testing is challenging but essential. Here are some practical ways to validate your Guarded Suspension implementation.
**1. Use Countdown Events and Latches
Use CountdownEvent
or similar primitives to synchronize threads during tests.
var countdown = new CountdownEvent(2);
Useful when you want to coordinate multiple threads reaching a specific state.
2. Leverage Task Timeouts
Wrap waits in timeouts to detect hangs or deadlocks.
var task = Task.Run(() => guardedQueue.DequeueAsync());
if (!task.Wait(TimeSpan.FromSeconds(5)))
{
throw new TimeoutException("Thread did not resume in time");
}
3. Simulate Load with Multiple Producers and Consumers
Stress-test the pattern with real thread pools and random delays to flush out edge cases.
4. Use Logging to Track State Transitions
Logging every wait, pulse, and condition change helps diagnose bugs quickly.
Conclusion and Best Practices
Guarded Suspension isn’t just a theoretical construct—it’s a practical solution to real-world synchronization problems. It allows threads to pause patiently until their time comes, making systems more reliable and efficient.
Best Practices Recap:
- Always check guard conditions inside a loop.
- Don’t forget to notify waiting threads when state changes.
- Favor built-in abstractions (
Channel<T>
,BlockingCollection
) unless you need fine-grained control. - Keep guarded regions as small and fast as possible.
- Test under stress. Concurrency bugs often hide in scale.
If you’re working in a system where timing and readiness matter, Guarded Suspension is a pattern you should have on speed dial. It’s not always the first tool to reach for, but in the right context, it brings order to the chaos of concurrent execution.
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →