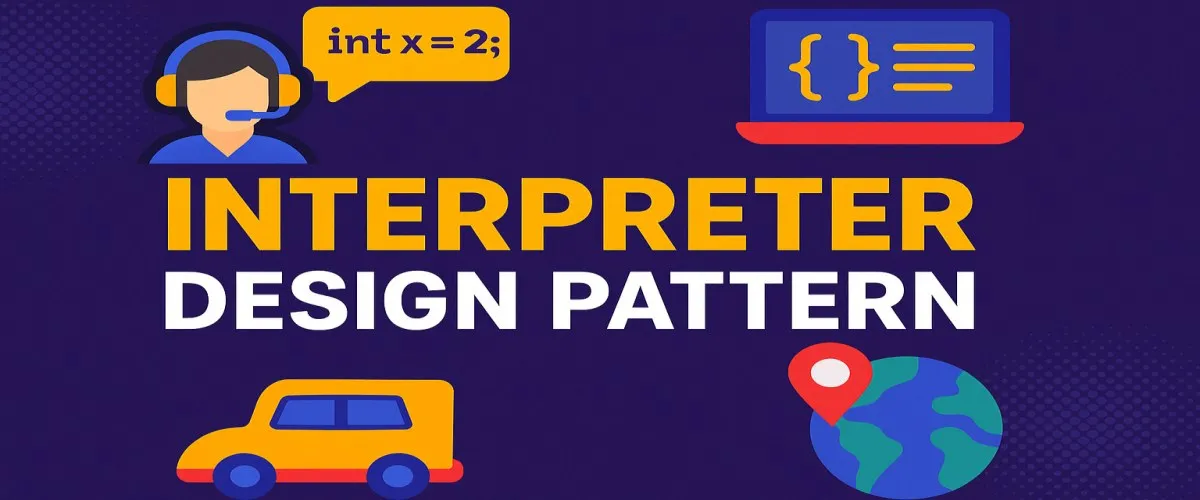
Interpreter Design Pattern Explained: A Deep Dive for C# Developers (With Real-World Examples)
Ever felt like explaining things to a machine is just too tough? Ever wished you could give instructions in a more human-readable way without getting tangled up in complex code logic? Well, my friend, you’re in luck—because that’s exactly where the Interpreter design pattern steps in!
This article dives deeply into the Interpreter pattern, especially for you brilliant software architects working with Microsoft technologies and C#. We’ll demystify this pattern step by step, sprinkle analogies throughout, and give you real, practical C# examples you can apply right now.
So buckle up, grab your coffee, and let’s unravel the magic!
What Exactly Is the Interpreter Design Pattern?
Imagine this: You’re traveling abroad and find yourself in a busy market. Everyone speaks a language you don’t understand. You have two choices: either learn their language from scratch or hire an interpreter who can translate it into your language. Easy choice, right?
In the software world, things aren’t much different. Sometimes, programs or apps need to understand and execute instructions written in a specific “language.” That language might be a simple query, a set of custom commands, or even a configuration instruction.
That’s where the Interpreter pattern comes in.
In simple words, the Interpreter pattern provides a way to evaluate grammar or expressions. It defines a representation of a grammar, allowing us to create interpreters that understand a language or expressions defined by our application.
Principles Behind the Interpreter Pattern
There are a few key principles that drive the Interpreter pattern. Let’s break these down, one at a time:
Principle 1: Separation of Concerns
Interpreter separates interpretation logic from the grammar rules. Imagine grammar rules as your cooking recipes, and interpretation logic as your cooking utensils. Recipes (grammar) stay clean and easy to manage, while utensils (interpretation) get the work done.
Principle 2: Extensibility
It’s super easy to extend the grammar. Want to add more expressions or commands? No problem! You don’t need to touch existing interpreters—just create new ones.
Principle 3: Clarity and Simplicity
The pattern aims for simplicity in expressing complex rules. Each interpreter has only one task—evaluate one type of expression. Easy peasy, lemon squeezy!
When Should You Use the Interpreter Pattern?
Alright, here’s the kicker. Interpreter isn’t for every scenario. So, when exactly should you whip out the Interpreter pattern?
- When your app needs a custom, mini-language to handle specific tasks.
- When your grammar rules are relatively simple or well-defined.
- When you have repetitive instructions or rules that can be clearly structured.
- When you need to dynamically evaluate expressions or commands at runtime.
Caution!
Avoid Interpreter if your language grammar is super complex (like, seriously complicated). You don’t want to end up tangled in interpreter spaghetti, right?
Key Components of the Interpreter Pattern
Every design pattern has its “cast of characters.” Here’s who you’ll meet in Interpreter:
1. Abstract Expression
This defines the common interface for all concrete expressions. It’s like the blueprint everyone else follows.
2. Terminal Expression
Represents the leaf nodes—actual words or symbols in your grammar. Think of these as the individual LEGO bricks. They don’t need further interpretation.
3. Non-terminal Expression
These expressions are built from terminal expressions. Think of non-terminal expressions as LEGO structures made from those bricks.
4. Context
Holds the data that needs to be interpreted. It’s your source code, query, or instruction set.
5. Client
The component that sets up the context and kicks off the interpretation process. This is you—or your code—using the interpreter.
Deep-Dive Implementation: Interpreter Pattern in C# (Very Detailed!)
Alright, enough theory! Let’s get our hands dirty with a solid, realistic example in C#.
Scenario:
Imagine you’re building a simple calculator app that interprets mathematical expressions like "5 + 3 - 2"
and returns the result. Let’s make our own simple math interpreter.
Step 1: Define the Abstract Expression
Here’s our abstract expression:
public interface IExpression
{
int Interpret();
}
Simple, right?
Step 2: Terminal Expression (Numbers)
Each number is a terminal expression:
public class NumberExpression : IExpression
{
private int _number;
public NumberExpression(int number)
{
_number = number;
}
public int Interpret()
{
return _number;
}
}
Terminal expressions are our basic building blocks—no further breakdown required.
Step 3: Non-terminal Expressions (Addition, Subtraction)
Let’s now build non-terminal expressions:
Addition Expression:
public class AddExpression : IExpression
{
private IExpression _leftExpression, _rightExpression;
public AddExpression(IExpression left, IExpression right)
{
_leftExpression = left;
_rightExpression = right;
}
public int Interpret()
{
return _leftExpression.Interpret() + _rightExpression.Interpret();
}
}
Subtraction Expression:
public class SubtractExpression : IExpression
{
private IExpression _leftExpression, _rightExpression;
public SubtractExpression(IExpression left, IExpression right)
{
_leftExpression = left;
_rightExpression = right;
}
public int Interpret()
{
return _leftExpression.Interpret() - _rightExpression.Interpret();
}
}
Step 4: Context (Parser)
We need a context or parser that understands our simple expressions:
public class ExpressionParser
{
public IExpression Parse(string input)
{
string[] tokens = input.Split(' ');
Stack<IExpression> stack = new Stack<IExpression>();
stack.Push(new NumberExpression(int.Parse(tokens[0])));
for (int i = 1; i < tokens.Length; i += 2)
{
string @operator = tokens[i];
int number = int.Parse(tokens[i + 1]);
IExpression rightExpression = new NumberExpression(number);
IExpression leftExpression = stack.Pop();
switch (@operator)
{
case "+":
stack.Push(new AddExpression(leftExpression, rightExpression));
break;
case "-":
stack.Push(new SubtractExpression(leftExpression, rightExpression));
break;
default:
throw new InvalidOperationException($"Unknown operator: {@operator}");
}
}
return stack.Pop();
}
}
This parser takes a string like "5 + 3 - 2"
and constructs an interpreter tree.
Step 5: Client (How to Use Your Interpreter)
class Program
{
static void Main(string[] args)
{
string expression = "5 + 3 - 2";
ExpressionParser parser = new ExpressionParser();
IExpression expr = parser.Parse(expression);
int result = expr.Interpret();
Console.WriteLine($"Result of '{expression}' is: {result}");
// Output: Result of '5 + 3 - 2' is: 6
}
}
Run this, and you’ll have a functioning interpreter!
Different Ways to Implement the Interpreter Pattern (with C# Examples)
So far, we’ve seen a straightforward, simple way to implement the Interpreter pattern. But did you know there’s more than one way to cook this particular dish? Yup! There are several different flavors you can choose from based on your requirements and context. Let’s check out the alternatives:
1. Classical Approach (Already Covered)
We’ve already done this—using individual classes to represent expressions. Simple, clean, and effective for small grammars. But, what about something a bit different?
2. Implementing Interpreter with Expression Trees (Advanced & Powerful!)
When your grammar becomes complex or performance-critical, C# expression trees can be a lifesaver. Think of them like the Ferrari of interpreters—fast, powerful, but requiring skilled hands to handle correctly.
Here’s a quick (yet solid!) example using Expression Trees in C#:
using System;
using System.Linq.Expressions;
class ExpressionTreeInterpreter
{
public Func<int> ParseAndCompile(string input)
{
var tokens = input.Split(' ');
Expression expr = Expression.Constant(int.Parse(tokens[0]));
for (int i = 1; i < tokens.Length; i += 2)
{
string op = tokens[i];
int number = int.Parse(tokens[i + 1]);
var right = Expression.Constant(number);
switch (op)
{
case "+":
expr = Expression.Add(expr, right);
break;
case "-":
expr = Expression.Subtract(expr, right);
break;
default:
throw new InvalidOperationException($"Operator {op} not supported");
}
}
return Expression.Lambda<Func<int>>(expr).Compile();
}
}
// Client Usage:
class Program
{
static void Main()
{
var interpreter = new ExpressionTreeInterpreter();
var compiledExpr = interpreter.ParseAndCompile("10 + 20 - 5");
Console.WriteLine($"Result is: {compiledExpr()}");
// Output: Result is: 25
}
}
Cool, right? Expression Trees compile expressions into executable code, making interpretation blazing-fast!
Real-world Use Cases for the Interpreter Pattern
You might be thinking, “Okay, cool! But when am I actually gonna use this?” Good question! Here are some practical examples:
1. Query Languages (SQL-like)
Ever wanted a tiny SQL-like language embedded in your app? Interpreter pattern is perfect!
2. Rule Engines
Business rules, validation logic, workflows—they all become easier to maintain when defined as interpreted rules.
3. Mini-calculators and Expression Evaluators
Think financial calculators, tax computations, or game logic expressions—Interpreter’s got your back!
4. Domain-Specific Languages (DSLs)
When your app has domain-specific commands, the Interpreter pattern makes it easy to introduce flexibility without recompiling your whole app.
Anti-patterns to Avoid: Interpreter Pattern Pitfalls
Whoa! Hold your horses! Before you jump into the deep end, let’s highlight some common pitfalls—anti-patterns to steer clear of:
1. Mega Interpreter Anti-pattern (a.k.a. “The God Interpreter”)
If your interpreter tries to do everything in a single class—run! It’ll quickly spiral into a maintenance nightmare. Keep your interpreters small, focused, and simple.
2. Over-Interpreting (or Interpreter Fever)
Just because you know how to implement Interpreter, doesn’t mean every little instruction needs one. Sometimes simpler solutions like switch-cases or tables will suffice.
3. Unnecessary Complexity
Don’t implement complex grammars in Interpreter. If your language grammar is gigantic, consider using tools like ANTLR or Roslyn instead.
Advantages of the Interpreter Pattern
Interpreter is not just another fancy pattern—it brings real, tangible advantages to your project. Let’s review:
Easy to Change & Extend
Want to add a new expression or rule? Simply add another interpreter without modifying existing code. Win-win!
Improves Readability
Each expression type is encapsulated clearly, enhancing readability and maintainability.
Dynamic Evaluation
Interpreters naturally support dynamic instructions, making your code super-flexible.
Great for Small to Medium Complexity
Perfectly fits scenarios with clearly defined and manageable rules.
Disadvantages of the Interpreter Pattern
But, alas, nothing in life (or software) is perfect. Let’s see the downsides clearly:
Performance Overhead
Since interpreter involves multiple classes and method calls, it can introduce some overhead. For ultra-performance-critical scenarios, carefully consider alternatives.
Complexity Grows Quickly
Simple grammars? Great! Complex grammar? Brace yourself—complexity increases dramatically, making the interpreter tough to manage.
Maintenance Challenges
If not carefully managed, lots of interpreter classes can become tough to navigate or debug.
Anti-patterns (Again!) Worth Emphasizing
Just to make sure you really avoid these nasty pitfalls, let’s quickly recap the key anti-patterns again:
- Mega Interpreter: Keep your interpreters specialized.
- Interpreter Fever: Don’t interpret unnecessarily. Keep it practical.
- Overcomplication: Stay away from overly complex grammars—seek alternatives if things get messy.
Quick Recap:
- What? Interpreter defines a language’s grammar and evaluates expressions.
- Principles: Separation of concerns, extensibility, simplicity.
- When? Simple grammars, repetitive tasks, mini-languages.
- Components: Abstract, Terminal, Non-terminal expressions, Context, Client.
- Real-world Use: Mini calculators, query builders, rule engines, DSLs.
Conclusion: Should You Use Interpreter?
So, here we are at the end of our Interpreter journey. You’ve learned the “what,” “how,” “why,” and even “why not” of the Interpreter pattern. You’ve got the C# examples to back you up, and you’re now ready to wield this powerful tool wisely.
Think of Interpreter as a specialized kitchen tool—super useful for certain recipes, not needed for others. When your application genuinely benefits from a structured, maintainable, and extendable interpretation mechanism, the Interpreter pattern is your best friend.
Final Thoughts:
- Keep your grammar simple.
- Use Interpreter only when it genuinely fits your scenario.
- Always balance simplicity with flexibility and performance.
Remember, patterns aren’t about following a rigid rulebook—they’re about making your life easier. So grab this Interpreter pattern, add it to your toolbox, and pull it out whenever you face those pesky “mini-language” challenges!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →