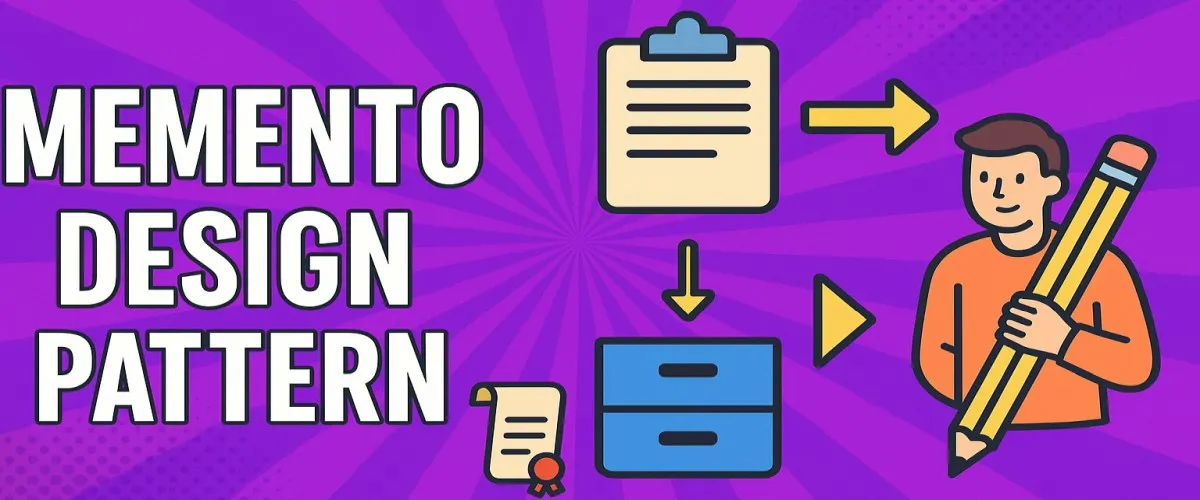
The Memento Design Pattern: Saving Your Objects' State (Without Losing Your Mind)
Ever found yourself wishing you had a “save” button in real life? Maybe you accidentally deleted a chunk of code, overwrote some critical data, or perhaps your latest code refactor went terribly wrong, and now you’re stuck trying to remember what worked before. What if I told you there’s a way to travel back in time—at least in your code? Welcome to the wonderful world of the Memento Design Pattern!
Imagine this: you’re playing a game, you reach a tricky level, and just before you make that risky jump, you quickly hit “save.” Why? Because if things don’t go as planned, you can reload and try again without losing progress. Wouldn’t it be amazing if you could do the same thing in your software architecture? Well, the good news is—you can, thanks to the Memento Pattern.
Ready to dive in? Let’s go!
What Exactly is the Memento Design Pattern?
The Memento Design Pattern is a behavioral design pattern that allows an object to save and restore its state without exposing its internal structure. Think of it like a snapshot or checkpoint for an object’s state, allowing you to revert to previous states without cluttering your object with extra code or breaking encapsulation.
Imagine your object is a character in a video game. You don’t want your character to remember every tiny detail of its history. You just want it to remember certain states—health points, position, inventory—so you can jump back if things go south.
The Memento does exactly that: it captures and externalizes an object’s state, letting you restore it later without revealing the object’s internals.
Principles Behind the Memento Pattern
Let’s break down why the Memento Pattern works so well:
1. Encapsulation
- The Memento pattern encapsulates the state within a separate object (the “memento”) without breaking the encapsulation of the original object.
- Think about it this way: your object is like a celebrity; its internal life (private state) should remain private. The Memento acts like a discreet assistant that knows exactly what the celebrity needs to remember, without leaking any juicy secrets to the press.
2. Single Responsibility Principle
- Each class in the Memento pattern has just one responsibility:
- Originator: Creates and restores the state.
- Memento: Stores the state.
- Caretaker: Keeps track of states.
- It’s like a movie set: the actor (Originator) does the acting, the cameraman (Memento) captures key scenes, and the director (Caretaker) keeps the footage organized.
3. Loose Coupling
- The pattern keeps components loosely coupled by ensuring the Originator doesn’t depend directly on the Caretaker or Memento’s details.
- Think of loose coupling like your smartphone and charger—different devices, connected by a standard USB cable. You can replace either easily without affecting the other.
When Should You Use the Memento Pattern?
Great question! Just because you can use a pattern doesn’t mean you always should. So, when is the Memento pattern really handy?
1. Undo/Redo Operations
- The classic use case: editors, drawing applications, and even IDEs, where users can undo and redo actions.
- Ever pressed Ctrl+Z? You’ve just used the memento pattern—sort of!
2. Stateful Objects
- Objects whose state can change frequently and unpredictably, and where you might need to restore previous states.
- For instance, tracking the state of an online form or configuration settings.
3. Snapshots and Checkpoints
- When your application requires the ability to save states at checkpoints, like in complex simulations or games.
- You know, that quick-save before the final boss fight—it’s essential!
4. Complex State Restoration
- If restoring the state of an object involves complex logic or resources, the Memento simplifies the process.
Key Components of the Memento Pattern
Every great recipe has key ingredients. Here are ours:
- Originator: The object whose state is captured.
- Memento: The object that holds a snapshot of the state.
- Caretaker: Manages the mementos but doesn’t modify or inspect their contents.
Detailed Implementation with C# Example
Okay, let’s dive into a complete, detailed C# example. We’ll implement a scenario where we have a Document editor that allows saving and restoring content states.
Scenario:
You’re creating a simple text editor application where users can write content, save versions, and revert to previous states.
Components:
- Document (Originator): Text content.
- DocumentMemento (Memento): Stores content snapshots.
- HistoryManager (Caretaker): Manages saved snapshots.
Step-by-Step C# Implementation:
Step 1: Create the Originator (Document
)
// Originator: Holds state and can create mementos
public class Document
{
private string _content;
public void Write(string content)
{
_content += content;
}
public string Content
{
get { return _content; }
}
// Saves the current state to a memento
public DocumentMemento Save()
{
return new DocumentMemento(_content);
}
// Restores state from a memento
public void Restore(DocumentMemento memento)
{
_content = memento.Content;
}
}
Step 2: Create the Memento (DocumentMemento
)
// Memento: Captures and stores the state
public class DocumentMemento
{
public string Content { get; }
public DocumentMemento(string content)
{
Content = content;
}
}
Step 3: Create the Caretaker (HistoryManager
)
// Caretaker: Manages saved mementos
public class HistoryManager
{
private Stack<DocumentMemento> _history = new Stack<DocumentMemento>();
public void Save(Document doc)
{
_history.Push(doc.Save());
}
public void Undo(Document doc)
{
if (_history.Any())
doc.Restore(_history.Pop());
}
}
Putting It All Together (Demo Program):
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
Document doc = new Document();
HistoryManager history = new HistoryManager();
doc.Write("Hello World! ");
history.Save(doc); // Save state 1
Console.WriteLine("Current content: " + doc.Content);
doc.Write("Let's learn Memento pattern. ");
history.Save(doc); // Save state 2
Console.WriteLine("Current content: " + doc.Content);
doc.Write("Oops, wrong sentence!");
Console.WriteLine("Current content: " + doc.Content);
// Undo last write
history.Undo(doc);
Console.WriteLine("After undo: " + doc.Content);
// Undo again
history.Undo(doc);
Console.WriteLine("After second undo: " + doc.Content);
}
}
Output:
Current content: Hello World!
Current content: Hello World! Let's learn Memento pattern.
Current content: Hello World! Let's learn Memento pattern. Oops, wrong sentence!
After undo: Hello World! Let's learn Memento pattern.
After second undo: Hello World!
Cool, right? You’ve just implemented a fully functional Memento Pattern in C#!
Different Ways to Implement the Memento Pattern (with C# Examples)
There’s never just one way to bake a cake, right? Similarly, the Memento pattern has different flavors too! Here are some ways you can spice things up:
1. Internal Memento (Using Nested Classes)
Sometimes you might not want others to see your secret recipe. A nested class ensures that only the originator knows about the memento’s contents. Think of it as a personal diary—no one else gets to peek!
Example in C#:
public class GameState
{
private string _level;
private int _score;
public void SetState(string level, int score)
{
_level = level;
_score = score;
}
public void Display()
{
Console.WriteLine($"Level: {_level}, Score: {_score}");
}
public Memento Save()
{
return new Memento(_level, _score);
}
public void Restore(Memento memento)
{
_level = memento.Level;
_score = memento.Score;
}
// Internal nested class
public class Memento
{
internal string Level { get; }
internal int Score { get; }
internal Memento(string level, int score)
{
Level = level;
Score = score;
}
}
}
Usage:
var game = new GameState();
game.SetState("Forest", 200);
var snapshot = game.Save();
game.SetState("Desert", 400);
game.Display(); // Desert, 400
game.Restore(snapshot);
game.Display(); // Forest, 200
2. Serialization-Based Memento
Serialization is a great way to capture complex states effortlessly. Imagine it like taking a screenshot rather than manually noting every pixel.
Example in C#:
using System.Text.Json;
public class SerializableState
{
public string Content { get; set; }
public string Save()
{
return JsonSerializer.Serialize(this);
}
public static SerializableState Restore(string serialized)
{
return JsonSerializer.Deserialize<SerializableState>(serialized);
}
}
Usage:
var state = new SerializableState { Content = "Hello, World!" };
string savedState = state.Save();
state.Content = "New Content";
Console.WriteLine(state.Content); // New Content
state = SerializableState.Restore(savedState);
Console.WriteLine(state.Content); // Hello, World!
Common Use Cases for the Memento Pattern
Wondering when exactly you’d call the memento for help? Here are some typical scenarios:
1. Text Editors & Graphics Applications
- Undo/Redo functionality in word processors or graphics software.
2. Games
- Save points, checkpoints, or quick saves to revert to earlier game states.
3. Complex Calculations & Simulations
- Storing calculation results or checkpoints in scientific software or simulations.
4. State Management in GUIs
- Remembering UI states or configurations users might wish to restore.
Anti-Patterns to Avoid When Using the Memento Pattern
Ever seen someone put sugar in pasta? Yeah, that’s what happens when you misuse patterns. Let’s dodge those mistakes!
Exposing Internal State Directly
- Memento should never expose the internal state publicly. That’s like showing your diary to strangers—just don’t!
Caretaker Manipulating Memento Content
- The Caretaker’s job is to store, not to modify. Don’t give it permission to edit the snapshots directly!
Overusing Snapshots
- Capturing a memento every single time something minor changes can be overkill—like taking selfies every second. Be strategic!
Advantages of Using the Memento Pattern
Here’s why you’ll love this pattern even more:
State Preservation without Breaking Encapsulation
- You can save states safely, without leaking your internal secrets.
Simplifies Undo Operations
- Implementing undo-redo becomes as easy as pie, keeping your users (and developers!) happy.
Reduced Complexity
- Managing historical states is organized, clean, and maintainable.
Clear Separation of Concerns
- Every component sticks to its responsibility—leading to clean, modular code.
Disadvantages of the Memento Pattern
Wait—there are downsides too? Yep, every rose has its thorn:
️ High Memory Usage
- Storing too many snapshots can eat memory quickly. Remember to prune your garden regularly.
️ Performance Overhead
- Creating and restoring snapshots might become expensive, especially with complex states.
️ Potential for Complexity in Management
- Caretaker complexity can grow quickly if managing lots of states. Be cautious!
More Anti-Patterns to Avoid (Bonus Tips!)
Did we say anti-patterns twice? Absolutely! It’s that important:
Multiple Originators with One Memento
- A single memento should represent one object’s state. Mixing different states in a single memento is like mixing pizza and ice cream in the same dish—awkward!
Using Memento for Every Small State Change
- Reserve mementos for meaningful state changes. Don’t spam your caretaker!
Conclusion: Should You Embrace the Memento Pattern?
By now, you’re probably thinking, “So, should I use this pattern or not?” Here’s your quick takeaway:
Use the Memento pattern if:
- Your application needs reliable undo/redo functionality.
- You require snapshots for critical checkpoints.
- You value encapsulation and clean architecture.
Avoid it if:
- Your application state is trivial.
- You have severe performance or memory constraints.
- The added complexity doesn’t justify the benefit.
Think of the Memento pattern as your personal time machine: great to have around, but use it wisely. Remember, design patterns aren’t a magic wand—they’re tools to help solve common problems. The Memento pattern is fantastic for managing object states efficiently, cleanly, and safely.
So, next time your code misbehaves, smile confidently, hit that undo button, and relax—your friendly memento has got your back!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →