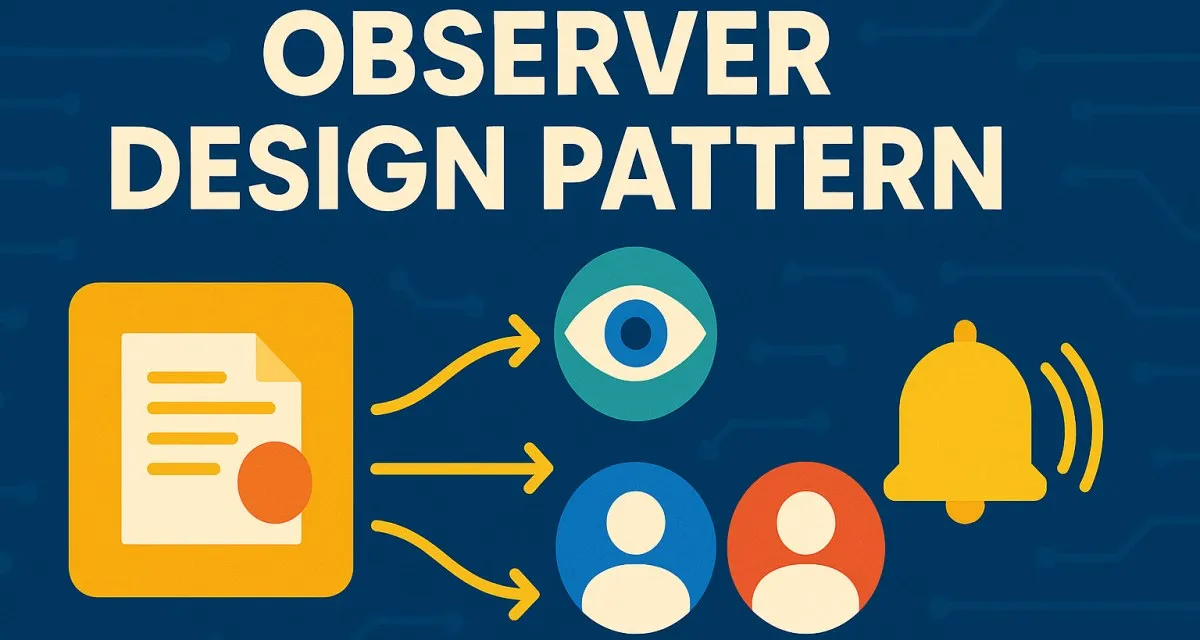
Observer Design Pattern Explained: A Deep Dive with C# (That Actually Makes Sense!)
- Sudhir mangla
- Behavioral design patterns
- 22 Apr, 2025
Introduction
Ever felt like you’re juggling way too many balls at once in your software application? Changes in one part of the codebase cascading into chaos elsewhere—sound familiar? If so, buckle up, friend, because you’re about to master a powerful design pattern that’ll simplify your life dramatically: the Observer Design Pattern.
In this article, we’ll break down the Observer pattern, using simple language and crystal-clear C# code examples. By the end, you’ll be ready to wield this pattern like a pro—and maybe even impress your boss or colleagues at your next meeting!
What is the Observer Pattern Anyway?
Think about your favorite streaming service. You subscribe once, and from that moment on, every new episode is automatically delivered to you. You don’t have to keep checking. You don’t have to repeatedly ask, “Hey, is there anything new yet?” Nope, the service lets YOU know when something new arrives.
That, my friend, is exactly what the Observer Pattern is all about!
The Observer Pattern is a behavioral design pattern that allows an object (called a Subject) to notify other objects (Observers) automatically whenever its state changes.
In simpler terms: the observer pattern makes sure everyone interested gets an instant notification whenever something exciting happens.
Principles Behind the Observer Pattern
The Observer pattern follows some core design principles:
- Open/Closed Principle: You can add new observers without changing existing code.
- Loose Coupling: Observers and subjects don’t need to know every detail about each other. They interact through clearly defined interfaces.
- Single Responsibility Principle: Subjects only handle state changes; Observers only handle reactions.
Pretty neat, right? It’s like a well-organized newsroom—one reporter (subject) reports breaking news, and all subscribers (observers) instantly get updates.
When Should You Use the Observer Pattern?
Great question! Use the Observer pattern when:
- A change in one object’s state requires updates to other objects.
- Your system is event-driven or involves real-time data updates.
- You want to create a publish-subscribe mechanism without tight coupling.
For example, think about applications like:
- Stock trading software: Stock price changes trigger multiple displays to update instantly.
- Social media notifications: New likes or comments trigger notifications for users.
Sound familiar? It should, because the Observer pattern is literally everywhere!
Key Components of the Observer Pattern
Let’s break down the roles clearly:
- Subject (Publisher): Maintains a list of observers and notifies them when its state changes.
- Observer (Subscriber): Receives notifications from the subject and updates itself accordingly.
- Concrete Subject: Implements the subject interface and tracks its own state.
- Concrete Observer: Implements the observer interface and defines how to react to state changes.
Implementing the Observer Pattern: Step-by-Step Guide in C#
Scenario:
Imagine we’re creating a simple weather station. Whenever the temperature changes, multiple displays (observers) need to update automatically.
Let’s get coding!
Detailed C# Example of Observer Pattern
Step 1: Defining the Observer Interface
public interface IObserver
{
void Update(float temperature);
}
Step 2: Creating Concrete Observers
Here’s where displays react to the temperature change:
public class WeatherDisplay : IObserver
{
private string _displayName;
public WeatherDisplay(string name)
{
_displayName = name;
}
public void Update(float temperature)
{
Console.WriteLine($"{_displayName} says: Temperature updated to {temperature}°C");
}
}
Simple enough, right?
Step 3: Defining the Subject Interface
The subject manages observers:
public interface ISubject
{
void RegisterObserver(IObserver observer);
void RemoveObserver(IObserver observer);
void NotifyObservers();
}
Step 4: Creating a Concrete Subject
This is the actual weather station that maintains and updates temperature:
public class WeatherStation : ISubject
{
private List<IObserver> _observers;
private float _temperature;
public WeatherStation()
{
_observers = new List<IObserver>();
}
public void RegisterObserver(IObserver observer)
{
_observers.Add(observer);
}
public void RemoveObserver(IObserver observer)
{
_observers.Remove(observer);
}
public void NotifyObservers()
{
foreach (var observer in _observers)
{
observer.Update(_temperature);
}
}
public void SetTemperature(float temperature)
{
Console.WriteLine($"WeatherStation: Setting temperature to {temperature}°C");
_temperature = temperature;
NotifyObservers();
}
}
Step 5: Connecting It All Together
Let’s write a quick test to see this in action:
class Program
{
static void Main(string[] args)
{
WeatherStation station = new WeatherStation();
WeatherDisplay phoneDisplay = new WeatherDisplay("Phone Display");
WeatherDisplay webDisplay = new WeatherDisplay("Web Display");
station.RegisterObserver(phoneDisplay);
station.RegisterObserver(webDisplay);
station.SetTemperature(25.0f);
station.SetTemperature(30.5f);
station.RemoveObserver(phoneDisplay);
station.SetTemperature(28.0f);
Console.ReadLine();
}
}
Step 6: Running the Example (Here’s What Happens)
Output:
WeatherStation: Setting temperature to 25°C
Phone Display says: Temperature updated to 25°C
Web Display says: Temperature updated to 25°C
WeatherStation: Setting temperature to 30.5°C
Phone Display says: Temperature updated to 30.5°C
Web Display says: Temperature updated to 30.5°C
WeatherStation: Setting temperature to 28°C
Web Display says: Temperature updated to 28°C
Notice how smoothly observers get notifications? That’s the Observer pattern in action—effortlessly keeping everyone informed!
Different Ways to Implement the Observer Pattern (with C# Examples)
Okay, you’ve got the basics down—awesome job! But guess what? There’s more than one way to tackle the Observer Pattern in C#. Let’s look at some alternative methods and see how they’re used.
1. Using Events and Delegates in C#
Events and delegates are built-in language features in C# that naturally support the Observer pattern. It’s like Microsoft saying, “Here, we’ve made it even easier!”
C# Example: Events and Delegates
Here’s our WeatherStation example using C# events and delegates:
public class WeatherStation
{
public delegate void TemperatureChangedHandler(float temperature);
public event TemperatureChangedHandler TemperatureChanged;
private float _temperature;
public void SetTemperature(float temperature)
{
Console.WriteLine($"WeatherStation: Temperature set to {temperature}°C");
_temperature = temperature;
TemperatureChanged?.Invoke(_temperature);
}
}
public class Display
{
private string _name;
public Display(string name, WeatherStation station)
{
_name = name;
station.TemperatureChanged += UpdateDisplay;
}
private void UpdateDisplay(float temperature)
{
Console.WriteLine($"{_name} Display: Current temperature is {temperature}°C");
}
}
// Usage
class Program
{
static void Main()
{
var station = new WeatherStation();
var phoneDisplay = new Display("Phone", station);
var webDisplay = new Display("Web", station);
station.SetTemperature(22.5f);
station.SetTemperature(25.0f);
}
}
Output:
WeatherStation: Temperature set to 22.5°C
Phone Display: Current temperature is 22.5°C
Web Display: Current temperature is 22.5°C
WeatherStation: Temperature set to 25°C
Phone Display: Current temperature is 25°C
Web Display: Current temperature is 25°C
Simple, clean, and built into the language itself—how cool is that?
2. Reactive Extensions (Rx.NET)
Another powerful implementation is the Reactive Extensions (Rx.NET). This approach is perfect for handling complex streams of events and data.
C# Example: Reactive Extensions
First, install System.Reactive
via NuGet. Now, let’s see a quick example:
using System;
using System.Reactive.Subjects;
public class WeatherStation
{
private Subject<float> _temperatureSubject = new Subject<float>();
public IObservable<float> TemperatureStream => _temperatureSubject;
public void SetTemperature(float temperature)
{
Console.WriteLine($"WeatherStation: Setting temperature to {temperature}°C");
_temperatureSubject.OnNext(temperature);
}
}
public class Display
{
private string _name;
public Display(string name, WeatherStation station)
{
_name = name;
station.TemperatureStream.Subscribe(Update);
}
private void Update(float temperature)
{
Console.WriteLine($"{_name} Display: Temperature updated to {temperature}°C");
}
}
// Usage
class Program
{
static void Main()
{
var station = new WeatherStation();
var mobileDisplay = new Display("Mobile", station);
var desktopDisplay = new Display("Desktop", station);
station.SetTemperature(18f);
station.SetTemperature(20f);
}
}
Using Rx.NET gives you superpowers when dealing with asynchronous or real-time data streams!
Use Cases: When the Observer Pattern Shines
Still wondering if the Observer pattern fits your use case? Let’s simplify it further:
- Real-Time Stock Market Applications: Stock prices change, and multiple observers update instantly.
- Social Media Notifications: Followers get instant notifications when something new happens.
- Sensor Networks and IoT Devices: Temperature, humidity, or motion sensor data updated immediately across multiple displays.
- Game Development: Updating multiple HUD elements or game components simultaneously based on user actions.
- E-commerce platforms: Instant inventory updates and real-time cart synchronization.
Anti-Patterns to Avoid with Observer (Don’t Do This!)
Avoid these common mistakes that can ruin your elegant Observer setup:
- God Subject (too many responsibilities): Don’t put unrelated logic into your subject. Keep it simple!
- Spamming Observers: Not every little change needs an observer notification—notify wisely!
- Direct Access to Subject’s state by Observers: Observers shouldn’t directly query or modify the subject. Maintain loose coupling!
- Assuming Notification Order: Observers shouldn’t rely on a notification order. It’s unpredictable and leads to bugs.
Advantages of the Observer Pattern
Here’s why developers love the Observer pattern:
- Loose Coupling: Observers and subjects barely know each other—easy to maintain and update independently.
- Dynamic Subscribers: Add or remove observers dynamically at runtime.
- Broadcast Communication: One-to-many communication handled effortlessly.
- Reusability & Extensibility: Adding new observers or subjects doesn’t break existing code (Open/Closed Principle in action!).
- Easier Testing: You can test subjects and observers independently.
Disadvantages of the Observer Pattern (No Pattern is Perfect!)
Sure, the Observer pattern is powerful—but beware of these trade-offs:
- Unexpected Updates: Observers may receive too many unnecessary updates if notifications aren’t carefully managed.
- Performance Overhead: Managing and notifying a large number of observers can introduce latency.
- Debugging Complexity: Difficult to trace bugs because updates are indirect and decoupled.
- Memory Leaks: Observers that forget to unsubscribe can stick around in memory longer than expected.
Anti-patterns to Avoid (Yes, Again!)
Wait, did I mention how important these pitfalls are? Because they really matter:
- Observer Leaks: Always unsubscribe observers when no longer needed.
- Chain Reactions: Avoid observers triggering additional notifications unintentionally.
- Hidden Dependencies: Keep dependencies explicit. Observers shouldn’t depend implicitly on internal subject states.
Conclusion: Observer Pattern—Your New Best Friend!
And that’s a wrap, folks! You’ve now mastered everything about the Observer Design Pattern. You’ve seen:
- What it is and how it simplifies event-driven software.
- Several ways to implement it in C#, including delegates, events, and Reactive Extensions.
- Clear use-cases, advantages, disadvantages, and pitfalls to avoid.
Now you can confidently build software that’s responsive, scalable, and easy to maintain. Next time your code has events, notifications, or updates—think Observer.
Remember, patterns aren’t just academic; they’re practical, powerful tools in your coding toolbox. So don’t just stop here—go put this knowledge to good use!