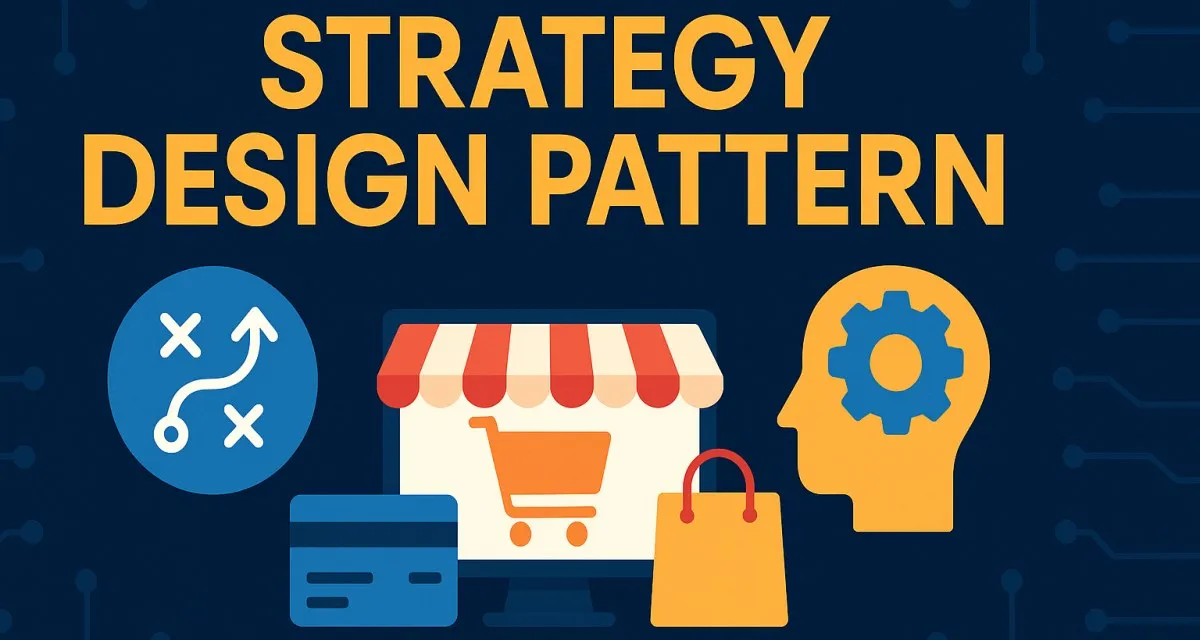
The Ultimate Guide to the Strategy Design Pattern in C#: How to Make Your Code Smarter, Cleaner, and Way More Flexible!
- Sudhir mangla
- Behavioral design patterns
- 24 Apr, 2025
Hey there, Software Architect! You’ve been knee-deep in Microsoft tech, wrestling with those nasty code monsters day in, day out. But what if I told you there’s a secret weapon that can help you beat those beasts? Yup, I’m talking about the Strategy Design Pattern. Ever heard of it?
If not, no worries! By the end of this guide, you’ll know the Strategy Pattern like the back of your keyboard. You’ll see how it can make your C# code cleaner, easier to manage, and more flexible—so you spend less time debugging and more time being awesome.
Ready to dive in? Let’s roll!
🧠 What Exactly Is the Strategy Design Pattern?
Imagine you’re going on a trip. You could get there by car, bus, or airplane, right? Each option is different, but they all have the same goal: get you from point A to point B.
That’s exactly how the Strategy Pattern works. It defines a family of algorithms, encapsulates each one, and makes them interchangeable at runtime. It lets the algorithm vary independently from clients that use it. In other words, your app doesn’t need to know exactly how things are getting done—it just knows what to do.
Let’s simplify that even more:
- Strategy Pattern = Interchangeable behaviors
- Think: plug-and-play. You just swap behaviors in and out—no complicated if-else chains cluttering your code!
📚 Core Principles of the Strategy Pattern
Okay, now let’s dig into some principles. These are crucial to mastering the pattern:
1. Encapsulate What Varies
This principle means you take the parts of your app that often change and isolate them from those that stay stable. It’s like organizing your desk: pens in one spot, notebooks in another. Easy to manage, right?
2. Favor Composition Over Inheritance
Instead of relying heavily on inheritance, we use composition—meaning objects can change behaviors at runtime by holding references to interfaces rather than inheriting them. Imagine building with Lego bricks—easy to add, remove, or rearrange.
3. Program to an Interface, Not an Implementation
Your code should depend on abstractions, not concrete implementations. Why? Because it makes your application more flexible. Think of it like using a universal adapter instead of a device-specific charger. You can plug in anything, anytime!
🎯 When Should You Use the Strategy Pattern?
Wondering when to whip out your new favorite pattern? Let’s make it easy:
- Multiple algorithms, same task: Got different ways to achieve the same goal? Strategy is your buddy.
- Reduce complexity: Tired of huge switch statements or long if-else chains cluttering your code? Time for Strategy!
- Dynamic behavior: Need to swap behaviors at runtime? Strategy pattern is exactly what you need.
A few classic examples:
- Payment systems (credit card, PayPal, etc.)
- Sorting algorithms (quick sort, merge sort, bubble sort)
- Compression techniques (ZIP, RAR, 7ZIP)
🧩 Key Components of the Strategy Pattern
Here’s a quick breakdown of what makes up the Strategy pattern:
Component | What it does | Real-life analogy |
---|---|---|
Context | Uses the strategy; the “consumer.” | Traveler |
Strategy (interface) | Defines the interface for all concrete strategies | Means of transportation |
Concrete Strategy | Implements the algorithm | Car, Bus, Plane |
Simple enough? Great!
💻 Deep Dive: Strategy Pattern Implementation in C# (With Detailed Example)
Alright, enough theory—let’s get our hands dirty with some C#. We’re gonna build a simple but real-world example: a payment processing system.
Scenario:
Imagine an online shop. Customers can pay using Credit Card, PayPal, or Crypto. Without a strategy pattern, you’d drown in if
statements. Let’s fix that!
⚙️ Step-by-Step Implementation with C#
Step 1: Define the Strategy Interface
// Payment Strategy Interface
public interface IPaymentStrategy
{
void Pay(decimal amount);
}
Step 2: Create Concrete Strategies
// Credit Card Payment Strategy
public class CreditCardPayment : IPaymentStrategy
{
private string _cardHolder;
private string _cardNumber;
private string _cvv;
private string _expiryDate;
public CreditCardPayment(string cardHolder, string cardNumber, string cvv, string expiryDate)
{
_cardHolder = cardHolder;
_cardNumber = cardNumber;
_cvv = cvv;
_expiryDate = expiryDate;
}
public void Pay(decimal amount)
{
Console.WriteLine($"Processing credit card payment for {amount:C}");
Console.WriteLine($"Card Holder: {_cardHolder}, Card Number: ****{_cardNumber.Substring(_cardNumber.Length - 4)}");
// Real payment logic here
}
}
// PayPal Payment Strategy
public class PayPalPayment : IPaymentStrategy
{
private string _email;
public PayPalPayment(string email)
{
_email = email;
}
public void Pay(decimal amount)
{
Console.WriteLine($"Processing PayPal payment for {amount:C} via {_email}");
// Real payment logic here
}
}
// Crypto Payment Strategy
public class CryptoPayment : IPaymentStrategy
{
private string _walletAddress;
public CryptoPayment(string walletAddress)
{
_walletAddress = walletAddress;
}
public void Pay(decimal amount)
{
Console.WriteLine($"Processing crypto payment for {amount:C} to wallet {_walletAddress}");
// Real payment logic here
}
}
Step 3: Define the Context Class
public class ShoppingCart
{
private IPaymentStrategy _paymentStrategy;
public void SetPaymentStrategy(IPaymentStrategy paymentStrategy)
{
_paymentStrategy = paymentStrategy;
}
public void Checkout(decimal amount)
{
if (_paymentStrategy == null)
{
throw new InvalidOperationException("Payment strategy not set.");
}
_paymentStrategy.Pay(amount);
}
}
🚀 Using Your Strategy (Example)
class Program
{
static void Main(string[] args)
{
var cart = new ShoppingCart();
// Paying with credit card
cart.SetPaymentStrategy(new CreditCardPayment("John Doe", "1234567890123456", "123", "12/27"));
cart.Checkout(99.99m);
// Switching to PayPal on the fly!
cart.SetPaymentStrategy(new PayPalPayment("john@example.com"));
cart.Checkout(149.99m);
// Switching to Crypto
cart.SetPaymentStrategy(new CryptoPayment("0xAB1234Ef567890..."));
cart.Checkout(299.99m);
}
}
Output:
Processing credit card payment for $99.99
Card Holder: John Doe, Card Number: ****3456
Processing PayPal payment for $149.99 via john@example.com
Processing crypto payment for $299.99 to wallet 0xAB1234Ef567890...
🚦 Different Ways to Implement the Strategy Pattern in C# (Yes, There’s More Than One!)
Now, let’s talk about different flavors of implementation. Just like ordering pizza—some prefer extra cheese, others love pepperoni—you have options with the Strategy Pattern too. Here are two popular alternatives:
🛠️ Method 1: Using Delegates & Lambda Expressions (Modern C# Way)
Want a simpler, cleaner way without creating extra classes? Delegates and lambda expressions are your ticket!
Example: Sorting Data
Here’s a fun example—sorting products by different criteria without complex code:
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
public class ProductSorter
{
private Func<List<Product>, List<Product>> _sortStrategy;
public void SetSortStrategy(Func<List<Product>, List<Product>> sortStrategy)
{
_sortStrategy = sortStrategy;
}
public List<Product> Sort(List<Product> products)
{
return _sortStrategy(products);
}
}
class Program
{
static void Main()
{
var products = new List<Product>
{
new Product { Name = "Laptop", Price = 1200 },
new Product { Name = "Tablet", Price = 600 },
new Product { Name = "Smartphone", Price = 800 }
};
var sorter = new ProductSorter();
// Sort by price ascending
sorter.SetSortStrategy(list => list.OrderBy(p => p.Price).ToList());
var sortedByPrice = sorter.Sort(products);
Console.WriteLine("Sorted by price (low to high):");
sortedByPrice.ForEach(p => Console.WriteLine($"{p.Name} - {p.Price:C}"));
// Sort by name descending
sorter.SetSortStrategy(list => list.OrderByDescending(p => p.Name).ToList());
var sortedByName = sorter.Sort(products);
Console.WriteLine("\nSorted by name (Z-A):");
sortedByName.ForEach(p => Console.WriteLine($"{p.Name} - {p.Price:C}"));
}
}
Why it rocks: Cleaner, simpler, no extra class clutter!
🛠️ Method 2: Dependency Injection (DI)
Got .NET Core? Awesome! DI helps implement Strategy super cleanly:
Example: Notification Service
public interface INotificationStrategy
{
void Notify(string message);
}
public class EmailNotification : INotificationStrategy
{
public void Notify(string message) => Console.WriteLine($"Email notification: {message}");
}
public class SmsNotification : INotificationStrategy
{
public void Notify(string message) => Console.WriteLine($"SMS notification: {message}");
}
public class NotificationService
{
private readonly INotificationStrategy _notificationStrategy;
public NotificationService(INotificationStrategy notificationStrategy)
{
_notificationStrategy = notificationStrategy;
}
public void Send(string message)
{
_notificationStrategy.Notify(message);
}
}
// Usage with DI
class Program
{
static void Main()
{
var service = new NotificationService(new EmailNotification());
service.Send("Your order is ready!");
service = new NotificationService(new SmsNotification());
service.Send("Your package is shipped!");
}
}
Why it rocks: Easy to test, super flexible, integrates perfectly into .NET Core apps!
📌 Common Use Cases (Where Strategy Pattern Shines!)
Let’s quickly recap where you’ll likely use Strategy pattern in real-world apps:
- Payment methods: Credit card, PayPal, Crypto
- Sorting: Dynamically changing sorting criteria
- Compression Algorithms: ZIP, RAR, 7ZIP, and more
- Validation: Different rules for user input based on context
- Logging: Changing logging methods (file, database, console)
- Notification: SMS, email, push notifications
❌ Strategy Pattern Anti-Patterns (Don’t Fall into These Traps!)
Avoid these common pitfalls:
- Too Many Strategies: Don’t create a strategy for absolutely everything! Ask yourself, “Do I really need dynamic behavior here?”
- Strategies With State: Strategies should ideally be stateless—don’t store states inside strategies.
- Strategy Explosion: Avoid having too many tiny classes. Simplify when possible using delegates or lambdas!
Pro Tip: Keep your strategies focused, lean, and straightforward. Less is more!
👍 Advantages of Strategy Pattern
Here’s why you’ll absolutely love it:
- Flexibility: Change behaviors at runtime—like swapping batteries!
- Clean Code: Eliminates nasty conditional clutter (
if
&switch
statements). - Maintainability: Easier to add or modify behaviors without breaking existing code.
- Open/Closed Principle (SOLID): Easily extend your application without modification.
- Testing & Mocking: Super easy to write tests, thanks to loose coupling!
👎 Disadvantages (Yes, There’s a Flip Side!)
But wait—there’s a catch:
- Complexity for Simple Tasks: Strategy adds complexity. Don’t use it where a simple
if
would suffice. - More Classes: You’ll end up with multiple classes/interfaces, potentially harder for newbies to understand.
- Runtime Decisions: More overhead when selecting strategies dynamically.
❌ Anti-Patterns to Avoid (Again—Because It’s Important!)
Because repetition reinforces memory:
- Don’t Overuse: Keep your strategies meaningful—don’t use Strategy just because you can.
- Stateful Strategies: Keep strategies stateless whenever possible.
- Strategy Classes Explosion: Limit strategies; prefer simpler implementations (lambdas, delegates).
📝 Quick Recap (TL;DR)
- What: Defines interchangeable algorithms.
- Principles: Encapsulate variations, composition over inheritance, program to interfaces.
- When to Use: Multiple ways of doing the same thing, simplify complexity, change behaviors at runtime.
- Components: Context, Strategy (interface), Concrete Strategies.
- Result: Cleaner, flexible, maintainable code.
🎯 Conclusion (Wrapping it All Up!)
You’ve made it! 🎉 You now have superpowers to make your codebase cleaner, simpler, and more adaptable. The Strategy Pattern in C# is like your coding Swiss Army knife—whether you’re handling payment gateways, sorting algorithms, or notification mechanisms, you can now confidently switch between behaviors without breaking a sweat.
Keep your code neat, your strategies lean, and remember—with great power comes great responsibility. Use your new pattern wisely!
Thanks for sticking with me. Now, go ahead—code smarter, not harder! 🥳