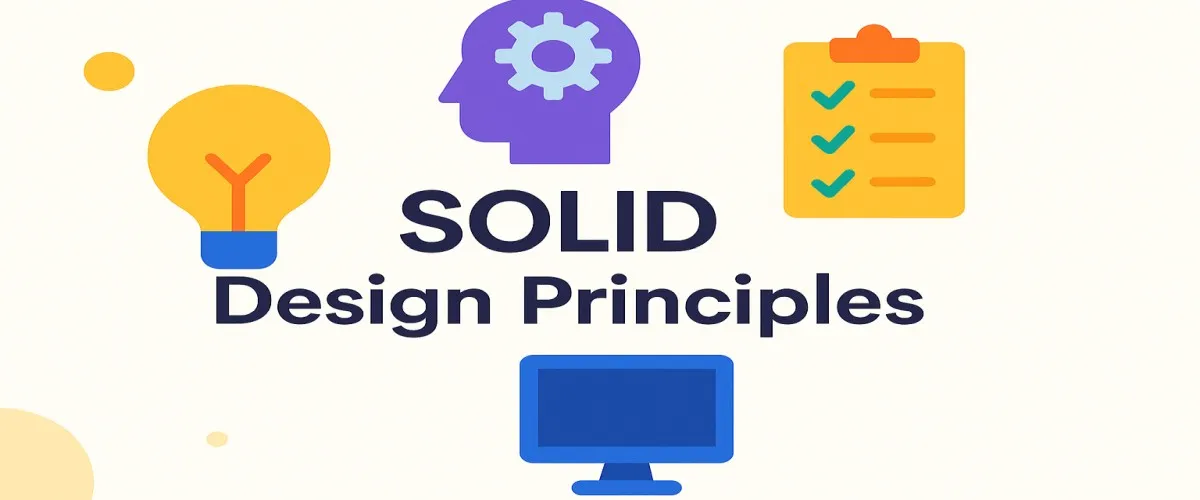
SOLID Design Principles: A Beginner’s Guide to Clean Software Architecture
- Sudhir mangla
- Design Principles , Clean Code
- 15 Mar, 2025
Introduction: What’s the Deal with SOLID, Anyway?
Have you ever found yourself swimming through layers of tangled code, desperately trying to patch up your application, only to see it fall apart the moment you add something new? You write one little feature, and suddenly, your tidy software turns into a messy monster that’s impossible to manage. Welcome to the frustrating—but common—world of spaghetti code.
Luckily, there’s a superhero team in the software architecture universe known as SOLID principles. Popularized by software legend Robert C. Martin, affectionately known as Uncle Bob, SOLID provides guidelines to keep your code neat, flexible, and reliable. Think of it like a team of friendly experts advising you on how to structure your applications, helping you avoid the headaches of complicated, brittle software.
In this detailed guide, we’ll explore SOLID principles step-by-step with clear explanations, vivid analogies, and practical C# and .NET examples. You’ll walk away with skills that’ll make your teammates admire your code and your future self breathe a sigh of relief.
Let’s dive in!
So, What Exactly Are SOLID Principles?
SOLID stands for five design principles that form the foundation of object-oriented programming (OOP). Each principle tackles common issues developers face, guiding you toward maintainable, reusable code:
- S: Single Responsibility Principle
- O: Open-Closed Principle
- L: Liskov Substitution Principle
- I: Interface Segregation Principle
- D: Dependency Inversion Principle
If you follow these principles, you’ll write software that’s easy to understand, test, and extend, making you an architect teammates love collaborating with.
Principle 1: Single Responsibility Principle (SRP)
“A class should have only one reason to change.”
Why Should You Care About SRP?
Imagine trying to fix a broken television that’s also your fridge, toaster, and washing machine—all in one appliance. Sounds ridiculous, right? But that’s exactly what many software classes look like when violating SRP. When your code tries to handle too many jobs, it becomes fragile and hard to maintain.
What Happens Without SRP?
Check out this typical scenario, with a class that tries to handle everything:
public class UserManager
{
public void RegisterUser(User user)
{
ValidateUser(user);
SaveToDatabase(user);
SendWelcomeEmail(user);
}
private void ValidateUser(User user) { /* validation logic */ }
private void SaveToDatabase(User user) { /* database logic */ }
private void SendWelcomeEmail(User user) { /* email logic */ }
}
Do you see the trouble?
- Change database logic? Modify this class.
- Update email templates? Modify this class again.
Multiple reasons to change means trouble!
Applying SRP Correctly
Here’s a better way to structure it, making your life easier:
// Responsible for user validation only
public class UserValidator
{
public bool IsValid(User user) { /* validation logic */ return true; }
}
// Responsible for database interactions only
public class UserRepository
{
public void Save(User user) { /* database logic */ }
}
// Responsible only for sending emails
public class EmailService
{
public void SendWelcomeEmail(User user) { /* email logic */ }
}
// Coordinates the user registration process
public class UserManager
{
private readonly UserValidator _validator = new();
private readonly UserRepository _repository = new();
private readonly EmailService _emailService = new();
public void RegisterUser(User user)
{
if (_validator.IsValid(user))
{
_repository.Save(user);
_emailService.SendWelcomeEmail(user);
}
}
}
Now, if you need to modify email logic, your validation logic stays safe and untouched. Each class has exactly one reason to change—mission accomplished!
Principle 2: Open-Closed Principle (OCP)
“Software entities should be open for extension but closed for modification.”
Why OCP Matters?
Consider LEGO bricks. You don’t need to break existing bricks to build new designs; you simply add more. Similarly, great software architecture lets you add features without changing existing code.
What Happens Without OCP?
Imagine dealing with this nightmare every time you add new payment methods:
public class PaymentProcessor
{
public void ProcessPayment(string paymentType)
{
if (paymentType == "CreditCard") { /* process credit card */ }
else if (paymentType == "PayPal") { /* PayPal logic */ }
// Imagine adding more types—total chaos!
}
}
Applying OCP Correctly
Let’s use abstraction to simplify extending functionality without altering code:
public interface IPaymentProcessor
{
void ProcessPayment(decimal amount);
}
public class CreditCardPaymentProcessor : IPaymentProcessor
{
public void ProcessPayment(decimal amount) { /* credit card logic */ }
}
public class PayPalPaymentProcessor : IPaymentProcessor
{
public void ProcessPayment(decimal amount) { /* PayPal logic */ }
}
public class PaymentService
{
public void ExecutePayment(IPaymentProcessor processor, decimal amount)
{
processor.ProcessPayment(amount);
}
}
Adding a new payment type is now effortless. Your code stays closed to modification and open to extension!
Principle 3: Liskov Substitution Principle (LSP)
“Subclasses should be substitutable for their base classes without affecting correctness.”
Why Does LSP Matter?
Think batteries—if your flashlight accepts AA batteries, any AA battery should work. Similarly, subclasses should seamlessly replace their parent classes without causing unexpected behaviors.
The Problem Without LSP
Consider a confusing relationship between Rectangle
and Square
:
public class Rectangle
{
public virtual int Width { get; set; }
public virtual int Height { get; set; }
}
public class Square : Rectangle
{
public override int Width
{
set { base.Width = base.Height = value; }
}
public override int Height
{
set { base.Height = base.Width = value; }
}
}
// Surprise behavior when substituting Square for Rectangle!
Rectangle rect = new Square();
rect.Width = 5;
rect.Height = 10;
Console.WriteLine(rect.Width * rect.Height); // Outputs 100 instead of 50!
Applying LSP Correctly
A cleaner approach using clear abstractions:
public abstract class Shape
{
public abstract int GetArea();
}
public class Rectangle : Shape
{
public int Width { get; set; }
public int Height { get; set; }
public override int GetArea() => Width * Height;
}
public class Square : Shape
{
public int Side { get; set; }
public override int GetArea() => SideLength * SideLength;
}
No surprises here! Each derived class behaves correctly, honoring LSP.
Principle 4: Interface Segregation Principle (ISP)
“Clients should not be forced to depend on methods they do not use.”
Why Should You Care About ISP?
Would you order the entire menu at a restaurant just to eat a burger? Exactly. Likewise, don’t burden classes with unnecessary methods.
What Happens Without ISP?
Here’s a bulky interface causing headaches:
public interface IPrinter
{
void Print();
void Fax();
void Scan();
}
What if your printer doesn’t scan or fax? You’re stuck implementing useless methods.
Applying ISP Correctly
Simplify by segregating interfaces:
public interface IPrinter { void Print(); }
public interface IFax { void Fax(); }
public interface IScanner { void Scan(); }
public class SimplePrinter : IPrinter
{
public void Print() { /* printing logic */ }
}
public class AdvancedPrinter : IPrinter, IFax, IScanner
{
public void Print() { /* printing logic */ }
public void Fax() { /* fax logic */ }
public void Scan() { /* scanning logic */ }
}
Clients now implement only what’s necessary. No waste!
Principle 5: Dependency Inversion Principle (DIP)
“High-level modules should not depend on low-level modules; both should depend on abstractions.”
Why DIP Matters?
You don’t plug devices directly into the power plant—you use a universal socket. Similarly, your classes shouldn’t directly depend on concrete implementations but on abstract interfaces.
Applying DIP Correctly
Introduce abstractions:
public interface ILogger
{
void Log(string message);
}
public class FileLogger : ILogger
{
public void Log(string message) { /* file logging */ }
}
public class UserService
{
private readonly ILogger _logger;
public UserService(ILogger logger) => _logger = logger;
public void CreateUser(User user)
{
// Create user logic
_logger.Log("User created successfully!");
}
}
Now your classes are flexible and easy to swap!
Conclusion
SOLID isn’t just theory—it’s your ticket to writing code that’s maintainable, readable, and enjoyable. Follow Uncle Bob’s timeless wisdom, and you’ll build software your team will love, reducing stress and increasing productivity. Happy coding!