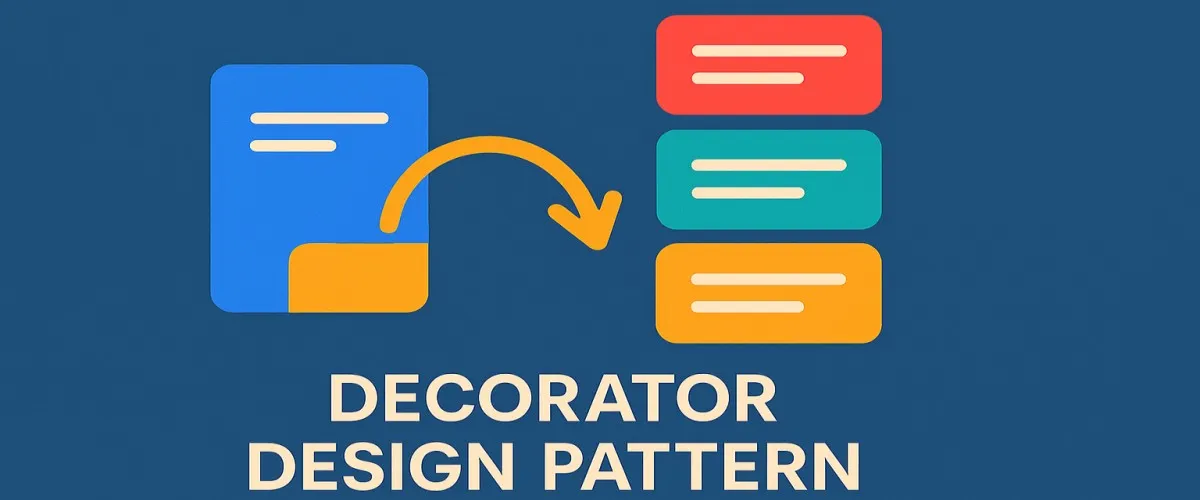
Decorator Design Pattern in C# Explained: Real-World Examples & Best Practices
Ever feel like you’re building something amazing, but adding a tiny new feature means rewriting the entire structure of your code? Yep, we’ve all been there. It’s like trying to put sprinkles on your ice cream after you’ve already poured hot fudge—it’s messy, complicated, and usually ends in tears. But don’t despair! Enter the Decorator Pattern, your new superhero sidekick, ready to save your architecture from complexity and clutter.
In this guide, we’re diving deep (I mean really deep!) into what the Decorator Pattern is, how it works, and—most importantly—how you can leverage it to make your C# code cleaner, more flexible, and easier to maintain.
What is the Decorator Pattern Anyway?
Let’s start simple. Imagine you’re ordering a pizza. You start with a basic Margherita—dough, sauce, cheese. But what if you suddenly crave pepperoni? Or mushrooms? Or extra cheese? You don’t throw out the pizza and start again, right? You add toppings!
That’s exactly how the Decorator Pattern works in software engineering. It’s a structural design pattern that allows you to dynamically add new behaviors or responsibilities to objects without changing their existing code.
Sounds cool, right? Now, let’s get into the nitty-gritty.
Core Principles of the Decorator Pattern
There are three key principles at play here:
1. Open/Closed Principle
“Software entities should be open for extension, but closed for modification.”
Decorator allows you to add functionality without changing the existing classes, aligning perfectly with this principle.
2. Composition Over Inheritance
Decorator emphasizes adding functionality through composition rather than inheritance. Instead of building complex hierarchies, you just decorate your object with more features.
3. Single Responsibility Principle
Each decorator handles exactly one new responsibility or behavior. This ensures your classes remain focused, lean, and maintainable.
When to Use the Decorator Pattern
You might be wondering: when is it the perfect time to use decorators?
- When you want to add responsibilities to objects dynamically at runtime.
- When using inheritance leads to complex, bloated class hierarchies.
- When extending functionality via inheritance could break existing implementations.
- When you want flexible and reusable object behaviors.
Key Components Explained
Here are the crucial components you’ll meet when implementing decorators:
Component | Description |
---|---|
Component Interface | Defines the methods that concrete components and decorators must implement. |
Concrete Component | The basic object that will receive additional responsibilities dynamically. |
Decorator | Maintains a reference to a Component object and conforms to the Component interface, delegating requests to it. |
Concrete Decorator | Adds responsibilities or behaviors to the Component. |
Decorator Pattern in Action: A Real-World Example in C#
Imagine you’re developing a text editor in C#. Initially, it just allows basic text entry, but your users want advanced features—bold text, italic text, spell-checking. Rather than rewriting your base class every time, decorators allow you to add these features dynamically.
Detailed Step-by-Step C# Implementation
Let’s get our hands dirty with some real code! Here’s a fully explained, step-by-step C# example implementing the Decorator Pattern.
Step 1: Define the Component Interface
public interface IText
{
string GetText();
}
Step 2: Implement the Concrete Component
public class PlainText : IText
{
private string _text;
public PlainText(string text)
{
_text = text;
}
public string GetText()
{
return _text;
}
}
Step 3: Define the Abstract Decorator Class
public abstract class TextDecorator : IText
{
protected IText _innerText;
protected TextDecorator(IText text)
{
_innerText = text;
}
public virtual string GetText()
{
return _innerText.GetText();
}
}
Step 4: Implement Concrete Decorators
Bold Decorator:
public class BoldDecorator : TextDecorator
{
public BoldDecorator(IText text) : base(text) { }
public override string GetText()
{
return "<b>" + base.GetText() + "</b>";
}
}
Italic Decorator:
public class ItalicDecorator : TextDecorator
{
public ItalicDecorator(IText text) : base(text) { }
public override string GetText()
{
return "<i>" + base.GetText() + "</i>";
}
}
Spell Check Decorator:
public class SpellCheckDecorator : TextDecorator
{
public SpellCheckDecorator(IText text) : base(text) { }
public override string GetText()
{
string correctedText = SpellCheck(base.GetText());
return correctedText;
}
private string SpellCheck(string text)
{
// Simulate spell checking
return text.Replace("teh", "the");
}
}
Step 5: Use It All Together!
class Program
{
static void Main(string[] args)
{
IText simpleText = new PlainText("Decorators are teh best!");
IText decoratedText = new SpellCheckDecorator(
new BoldDecorator(
new ItalicDecorator(simpleText)));
Console.WriteLine(decoratedText.GetText());
// Output: <b><i>Decorators are the best!</i></b>
}
}
Boom! You’ve dynamically added features—without modifying your base class. Isn’t that neat?
Decorator vs. Inheritance: Battle of the Patterns
Decorator:
- Dynamically adds functionality at runtime.
- Avoids deep inheritance hierarchies.
- Highly flexible and composable.
Inheritance:
- Less flexible—changes are compile-time.
- Leads to complex, rigid class structures.
- Simpler for straightforward scenarios.
Different Ways to Implement the Decorator Pattern in C#
Now that you’ve got the basics down, let’s explore a few different ways to implement decorators in C#. The flexibility of this pattern means there are plenty of options. Let’s look at three of the most common methods:
1. Using Abstract Classes (Traditional Approach)
This is the classic implementation—what we already covered briefly. It relies on abstract classes and inheritance. This method provides clarity and keeps your decorators neatly organized.
C# Example (Quick recap):
// Interface
public interface INotifier
{
void Send(string message);
}
// Concrete Component
public class EmailNotifier : INotifier
{
public void Send(string message)
{
Console.WriteLine($"Email sent: {message}");
}
}
// Abstract Decorator
public abstract class NotifierDecorator : INotifier
{
protected INotifier _notifier;
protected NotifierDecorator(INotifier notifier)
{
_notifier = notifier;
}
public virtual void Send(string message)
{
_notifier.Send(message);
}
}
// Concrete Decorator
public class SMSNotifier : NotifierDecorator
{
public SMSNotifier(INotifier notifier) : base(notifier) { }
public override void Send(string message)
{
base.Send(message);
Console.WriteLine($"SMS sent: {message}");
}
}
2. Using Interfaces Only (Pure Composition)
Want to stay away from abstract classes entirely? You can! Use pure interfaces to build your decorators—super simple, ultra-flexible.
C# Example:
public interface ILogger
{
void Log(string message);
}
public class ConsoleLogger : ILogger
{
public void Log(string message)
{
Console.WriteLine($"Log: {message}");
}
}
public class TimestampLogger : ILogger
{
private readonly ILogger _logger;
public TimestampLogger(ILogger logger)
{
_logger = logger;
}
public void Log(string message)
{
string timestampedMessage = $"[{DateTime.Now}] {message}";
_logger.Log(timestampedMessage);
}
}
3. Using Delegates and Functional Decorators (Modern Approach)
Feeling adventurous? Functional decorators using delegates can make your decorators concise and powerful. Here’s how:
C# Example:
public delegate void NotificationHandler(string message);
public class Notifier
{
private readonly NotificationHandler _handler;
public Notifier(NotificationHandler handler)
{
_handler = handler;
}
public void Notify(string message)
{
_handler(message);
}
}
// Functional decorators
NotificationHandler emailHandler = msg => Console.WriteLine($"Email: {msg}");
NotificationHandler smsHandler = msg => Console.WriteLine($"SMS: {msg}");
// Composition
var combinedNotifier = new Notifier(emailHandler + smsHandler);
combinedNotifier.Notify("You got mail!");
See? Multiple paths to decorating your objects. Choose your favorite approach based on your project’s needs, complexity, and coding style.
Real-world Use Cases for the Decorator Pattern
Wondering when you’d actually use decorators in real life? Here are some common scenarios:
1. UI Enhancements:
- Dynamically adding tooltips, borders, shadows, and behaviors to UI elements.
2. Data Streams:
- Wrapping data streams with buffering, encryption, or compression features (think:
System.IO.Stream
in .NET).
3. Logging & Monitoring:
- Enhancing your methods with logging, performance metrics, or security checks without cluttering your business logic.
4. Game Development:
- Adding dynamic abilities, buffs, or power-ups to game characters on the fly.
5. E-commerce Applications:
- Adding dynamic features like promotional discounts, shipping options, or taxes to shopping carts without modifying your core logic.
Advantages of the Decorator Pattern
Let’s recap the juicy benefits:
-
Dynamic Extension:
Add or remove behaviors at runtime without messing up your existing code. -
Promotes Composition over Inheritance:
Avoid deep, rigid inheritance trees, making your code easier to maintain and extend. -
Flexible and Scalable:
Combine and mix decorators freely for endless possibilities. -
SOLID-friendly:
Supports the Single Responsibility Principle and the Open/Closed Principle effortlessly. -
Easy to Test:
Decorators simplify unit testing by clearly separating concerns.
Disadvantages of the Decorator Pattern
Every superhero has weaknesses, right? Here’s where decorators might cause headaches:
-
Complexity with Excessive Nesting:
Too many decorators stacked together can become confusing and hard to debug. -
Runtime Overhead:
Each decorator introduces another layer of indirection, potentially impacting performance slightly. -
Initialization Complexity:
The more decorators, the harder your objects become to set up and maintain clearly. -
Learning Curve:
Developers unfamiliar with decorators might struggle initially, potentially slowing down onboarding.
Conclusion: Decorators—Your New Coding Superpower!
The Decorator Design Pattern in C# is like having a powerful Swiss Army knife in your toolbox. Need to quickly add a feature without rewriting your whole app? Decorators have your back. Want clean, maintainable code that’s fun to work with? Yep, decorators again!
Throughout this guide, we explored what decorators are, their principles, when and how to implement them in various ways (abstract classes, interfaces, delegates—take your pick!), their real-world uses, and weighed their pros and cons.
Yes, decorators add complexity—but it’s manageable complexity, the kind that brings clarity, maintainability, and extensibility to your code. Used wisely, decorators help you create software that’s not only powerful but also a joy to maintain.
So why not give decorators a spin? Start small, experiment, and soon you’ll wonder how you ever coded without them. Happy decorating!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →