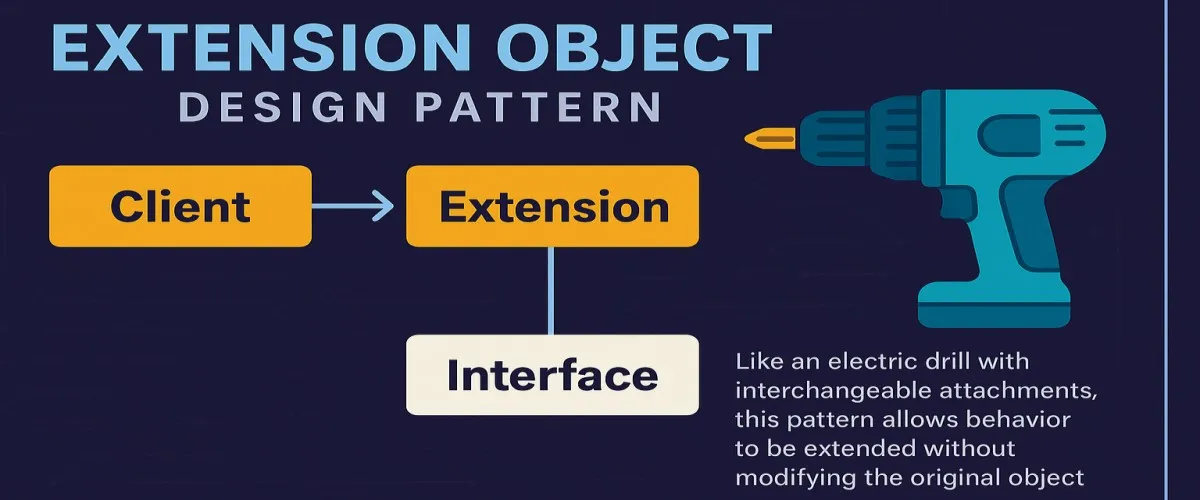
Mastering the Extension Object Design Pattern in C#: Expand Your Software Like a Pro!
- Sudhir mangla
- Design Patterns
- 02 Apr, 2025
Ever had that sinking feeling when your beautifully designed classes suddenly start looking like spaghetti code because of endless new requirements? Yeah, we’ve all been there. But fear not! There’s a superhero hiding in plain sight: the Extension Object Pattern.
Picture this: you’re playing with Lego bricks. You’ve built a perfect spaceship. Now someone hands you extra bricks saying, “Hey, add these cool lasers and antennas!” What do you do? You don’t tear down the spaceship and rebuild from scratch—no way! You simply snap those extensions on. That’s exactly what the Extension Object Pattern lets you do with your code.
In this article, we’ll dive deep into the Extension Object Pattern—breaking it down with clear explanations, vivid analogies, and tons of real-world C# examples to turn you into a design-pattern ninja.
Ready to level up your software architecture game? Let’s jump in!
🚀 What Exactly is the Extension Object Pattern?
Imagine you have a base class that works perfectly—clean, neat, and does exactly what it’s supposed to. But as the project evolves, someone inevitably asks, “Can we add just one more tiny feature?” And suddenly, your pristine class is bursting at the seams. That’s when the Extension Object Pattern saves your sanity.
In simple terms, the Extension Object Pattern allows you to add new functionality to existing classes without modifying their original code. Instead, you create separate objects—called extensions—that “plug-in” seamlessly to the original objects.
Metaphor time: Think of your base class as a smartphone and extensions as apps. You don’t alter the smartphone hardware every time you need a new feature, right? You simply install apps!
🎯 Core Principles of the Extension Object Pattern
Here’s the juicy part: the underlying principles that make this pattern so awesome.
Principle #1: Open-Closed Principle (OCP)
Your classes should be open for extension but closed for modification. Yep, exactly like a house that’s open for guests but closed for renovation every weekend.
Principle #2: Single Responsibility Principle (SRP)
Each class or extension should have one reason to change, just like you have one drawer for socks and another for shirts. Keep it tidy!
Principle #3: Separation of Concerns
Keep your original object separate from additional functionality—just like how your phone doesn’t become a toaster when you install a cooking app.
🕵️♂️ When Should You Use Extension Object?
Great question! Here are three major scenarios:
- Dynamic Feature Addition: When your system requires new features frequently without affecting existing ones.
- Third-Party Extensions: When you expect extensions from outside developers without letting them tamper with your core.
- Complex Conditional Logic: When your code begins drowning in endless
if-else
statements. (Yikes!)
🛠️ Key Components of the Extension Object Pattern
Before we jump into the deep end, let’s get familiar with the key players:
- Subject Interface: Defines basic operations that original objects should have.
- Concrete Subject: Implements the subject interface. This is your base object (your “smartphone”).
- Extension Interface: Defines additional behaviors or functionalities.
- Concrete Extension Object: Implements these behaviors and attaches them to the subject.
Now, let’s put theory into practice!
🔥 Hands-On Implementation in C#: A Super-Detailed Example!
Let’s say you’re developing an e-commerce application, and you have an Order
class. Everything’s going smoothly until your boss walks in saying, “We need to calculate taxes and offer special discounts dynamically, based on customer type.” Classic scenario, right?
If you cram all this logic into your Order
class, your code will soon resemble tangled headphones. Let’s prevent this mess by implementing the Extension Object Pattern instead.
Step 1: Define Your Subject Interface
public interface IOrder
{
decimal CalculateTotal();
}
Step 2: Create Concrete Subject (The Basic Order Class)
public class Order : IOrder
{
public List<OrderItem> Items { get; set; } = new List<OrderItem>();
public decimal CalculateTotal()
{
return Items.Sum(item => item.Price * item.Quantity);
}
}
public class OrderItem
{
public string Name { get; set; }
public decimal Price { get; set; }
public int Quantity { get; set; }
}
Step 3: Define Extension Interface
We’ll create an interface that every extension implements. Let’s call it IOrderExtension
.
public interface IOrderExtension
{
decimal CalculateExtensionTotal(decimal currentTotal);
}
Step 4: Create Concrete Extensions
Now, we’ll create different extensions for different functionalities.
a. Tax Calculation Extension
public class TaxExtension : IOrderExtension
{
private readonly decimal _taxRate;
public TaxExtension(decimal taxRate)
{
_taxRate = taxRate;
}
public decimal CalculateExtensionTotal(decimal currentTotal)
{
return currentTotal + (currentTotal * _taxRate);
}
}
b. Discount Extension
public class DiscountExtension : IOrderExtension
{
private readonly decimal _discount;
public DiscountExtension(decimal discount)
{
_discount = discount;
}
public decimal CalculateExtensionTotal(decimal currentTotal)
{
return currentTotal - _discount;
}
}
Step 5: Create the Extension-Enabled Order Wrapper
Let’s wrap our original Order
object so it can dynamically use extensions.
public class OrderWithExtensions : IOrder
{
private readonly IOrder _baseOrder;
private readonly List<IOrderExtension> _extensions = new List<IOrderExtension>();
public OrderWithExtensions(IOrder baseOrder)
{
_baseOrder = baseOrder;
}
public void AddExtension(IOrderExtension extension)
{
_extensions.Add(extension);
}
public decimal CalculateTotal()
{
decimal total = _baseOrder.CalculateTotal();
foreach(var extension in _extensions)
{
total = extension.CalculateExtensionTotal(total);
}
return total;
}
}
Step 6: Using Extensions in Action
Here’s how your awesome new system works:
class Program
{
static void Main(string[] args)
{
// Create base order
var order = new Order();
order.Items.Add(new OrderItem { Name = "Book", Price = 100, Quantity = 2 });
order.Items.Add(new OrderItem { Name = "Pen", Price = 5, Quantity = 10 });
// Wrap the order for extensions
var extendedOrder = new OrderWithExtensions(order);
// Dynamically add Tax & Discount extensions
extendedOrder.AddExtension(new TaxExtension(0.10m)); // 10% tax
extendedOrder.AddExtension(new DiscountExtension(15)); // $15 discount
// Calculate the final total
decimal finalTotal = extendedOrder.CalculateTotal();
Console.WriteLine($"Final Order Total: ${finalTotal}");
}
}
Output:
Final Order Total: $220
Boom! You’ve added dynamic functionality without altering your original Order
class. How cool is that?
🔍 Different Ways to Implement Extension Object Pattern (with C# Examples)
Did you know there’s more than one way to make tasty pancakes? Likewise, the Extension Object Pattern can also be implemented in various clever ways. Let’s explore some popular methods:
1. Using the Dictionary-based Extension Object Implementation
Think of this method like putting your items into clearly labeled drawers—simple, accessible, and practical!
C# Example:
public class ExtensibleObject
{
private readonly Dictionary<Type, object> _extensions = new Dictionary<Type, object>();
public void AddExtension<T>(T extension)
{
_extensions[typeof(T)] = extension;
}
public T GetExtension<T>()
{
return _extensions.TryGetValue(typeof(T), out var extension) ? (T)extension : default(T);
}
}
Usage:
var order = new ExtensibleObject();
order.AddExtension(new TaxExtension(0.15m));
order.AddExtension(new DiscountExtension(20));
var taxExtension = order.GetExtension<TaxExtension>();
Console.WriteLine($"Total after tax: {taxExtension.CalculateExtensionTotal(100)}");
2. Using Composition-based Implementation
Composition is like assembling modular furniture—each piece (extension) can be added or removed without affecting others.
C# Example:
public interface IComponent
{
void Execute();
}
public class CompositeObject : IComponent
{
private readonly List<IComponent> _extensions = new List<IComponent>();
public void AddComponent(IComponent component) => _extensions.Add(component);
public void Execute()
{
foreach (var component in _extensions)
component.Execute();
}
}
public class LoggingExtension : IComponent
{
public void Execute() => Console.WriteLine("Logging executed!");
}
Usage:
var composite = new CompositeObject();
composite.AddComponent(new LoggingExtension());
composite.Execute(); // Output: Logging executed!
🎬 Real-World Use Cases for the Extension Object Pattern
“Okay, sounds cool—but where do I really use this pattern?” Glad you asked! Here are some common scenarios:
1. Plugin Systems
Ever downloaded a plugin for your browser? Plugins use extension-like logic. Each plugin is a separate extension attached to a browser.
2. Games Development
Imagine a character in your game gaining new skills or abilities at runtime. You wouldn’t rewrite the character class; you’d extend its capabilities dynamically.
3. E-commerce & Financial Applications
Dynamic calculations of taxes, discounts, loyalty points, or fraud checks are perfect for the Extension Object Pattern.
🚨 Anti-patterns to Avoid (Don’t Fall into These Traps!)
Remember, with great power comes great responsibility. Let’s see some classic traps developers fall into:
🔻 Anti-pattern #1: Overusing Extensions
It might seem tempting to shove everything into an extension. But beware—too many extensions will turn your neat Lego set into a confusing pile of bricks. Keep it modular, but sensible.
🔻 Anti-pattern #2: Hardcoding Extensions
Extensions should be dynamic and configurable. If you’re creating extensions that can’t be easily added, removed, or replaced, you’re missing the point.
🔻 Anti-pattern #3: Ignoring Type Safety
Avoid generic “object” types or overly generic dictionaries without clear typing. Always prefer strongly typed extensions for clarity and safety.
🔻 Anti-pattern #4: Excessive Conditional Logic
If you find yourself constantly checking if an extension exists before using it (if-else
statements everywhere), rethink your design. Consider defaults or null-object patterns instead.
🔻 Anti-pattern #5: Cyclic Dependencies
Extensions should be independent modules. If your extensions rely heavily on each other, refactor to reduce coupling—otherwise, you risk spaghetti extensions.
📈 Advantages of the Extension Object Pattern
Wondering why you’d pick Extension Object over other patterns? Let’s quickly highlight its strengths:
- ✅ High Flexibility: Easily plug in and out functionality at runtime.
- ✅ Maintainability: Keeps your original classes clean, reducing the risk of introducing bugs when adding new features.
- ✅ Dynamic Behavior: Supports runtime configuration, ideal for plugin architectures.
- ✅ Compliant with SOLID Principles: Particularly the Open-Closed Principle (OCP), promoting solid architecture practices.
- ✅ Easy Collaboration: Allows external teams or third-party developers to safely extend your codebase without accessing the core implementation.
📉 Disadvantages (Things That May Bite You)
Let’s be fair and transparent. Every pattern has its flaws. Here’s where Extension Object can trip you up:
- ❌ Increased Complexity: The more extensions, the harder it might become to track behaviors at runtime.
- ❌ Performance Overhead: Slight performance hit due to the extra indirection in calling extensions, though typically negligible.
- ❌ Steep Learning Curve for New Developers: Developers unfamiliar with dynamic composition may initially struggle with understanding and using extensions effectively.
- ❌ Debugging Difficulty: More dynamic behavior can complicate debugging—it’s harder to trace exactly what extensions are executing at runtime.
🎯 Wrapping It All Up – A Quick Conclusion!
You’ve made it! By now, you’re a certified Extension Object Pattern ninja. You know:
- What the Extension Object Pattern is.
- Why it matters and when to use it.
- Multiple ways to implement it effectively.
- Real-world examples and practical use cases.
- Advantages and disadvantages to consider carefully.
- Critical anti-patterns that you’ll definitely avoid.
Using this pattern strategically is like having a Swiss Army knife in your coding toolkit—it empowers you to elegantly handle evolving requirements without compromising code quality. Just remember, like seasoning in a recipe, use it in moderation and with intention.
Now go ahead, apply this knowledge, and let your software architecture shine!