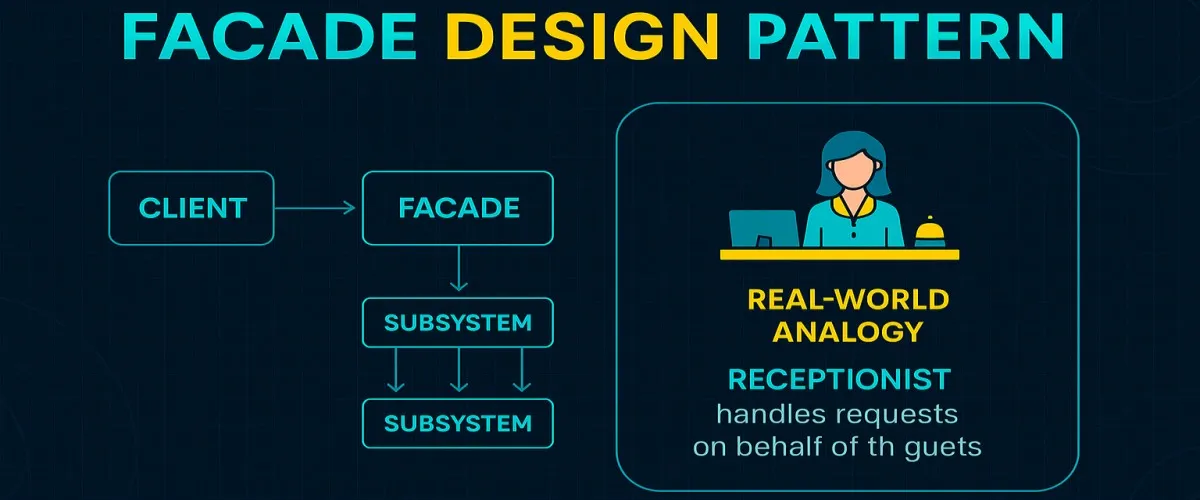
Understanding the Facade Design Pattern: Simplify Your C# Applications!
Hey there, fellow architect! Ever felt like your application’s structure is growing into a sprawling mess, with components interacting all over the place? You know what I mean—that moment when adding a simple feature turns into an episode of detective work. Yeah, it’s frustrating. But what if I told you there’s a design pattern specifically designed to keep complexity in check?
Enter the Facade Pattern—your application’s friendly receptionist, making complex systems feel simple and welcoming.
In this ultimate guide, we’ll dive deep into the Facade Pattern with practical C# examples. You’ll understand exactly when and how to implement it. Ready? Let’s simplify!
What is the Facade Design Pattern?
Imagine you’re ordering food at a restaurant. You don’t walk straight into the kitchen and start shouting your order to the chefs, do you? Of course not! Instead, you talk to the waiter. The waiter then coordinates your request with the chefs, the bartender, and the cashier. That waiter is your Facade.
The Facade Design Pattern provides a simplified interface to a complex subsystem. It hides the details, makes the subsystem easier to use, and keeps clients from worrying about what’s happening behind the scenes.
Here’s what the Facade looks like in a nutshell:
Client --> Facade --> Complex Subsystem
Easy, right? Let’s dig deeper.
Core Principles of the Facade Pattern
Before implementing it, let’s ensure you fully grasp its principles:
- Simplification: Reduce complexity by providing a single, simplified interface.
- Abstraction: Hide intricate subsystem details from your clients.
- Loose coupling: Decouple the client from the subsystem, making your application more maintainable and scalable.
- Single responsibility: Facades are responsible only for simplifying interactions, not business logic or heavy processing.
When Should You Use the Facade Pattern?
Wondering when to unleash the Facade’s power? Here are a few scenarios:
- Complex subsystems: Your application integrates multiple complicated systems, APIs, or legacy codebases. You want simpler interactions.
- Layer separation: You need clear boundaries between the business logic, UI, and data layers.
- Integration and APIs: Providing a straightforward API for clients to interact with a complex service.
- Refactoring and legacy code: When gradually simplifying a messy codebase (yes, we’ve all inherited those!).
Still uncertain? Just ask yourself this: “Could my team understand and manage the complexity faster with a Facade?” If the answer is yes—Facade it is!
Key Components of the Facade Pattern
Let’s meet the stars of this pattern:
- Facade Class: The simplified interface clients interact with.
- Subsystem Classes: The complex, individual components behind the scenes.
- Client: Uses the Facade rather than the subsystem directly.
Simple Diagram:
[Client] ---calls---> [Facade] ---coordinates---> [Subsystem A, B, C...]
Now, let’s get practical!
Detailed Implementation in C# (Step-by-Step Example)
Ready to code? Let’s build a real-world scenario: Booking a holiday package. Booking involves multiple complicated systems—flights, hotels, cars, payment gateways—let’s simplify it with a Facade.
Step 1: Defining Subsystems
Let’s first define our subsystems (Flights, Hotels, Car Rentals):
// Flight Booking subsystem
public class FlightBooking {
public void BookFlight(string origin, string destination) {
Console.WriteLine($"Booking flight from {origin} to {destination}");
}
}
// Hotel Booking subsystem
public class HotelBooking {
public void BookHotel(string location, int nights) {
Console.WriteLine($"Booking hotel in {location} for {nights} nights");
}
}
// Car Rental subsystem
public class CarRental {
public void RentCar(string location, int days) {
Console.WriteLine($"Renting car at {location} for {days} days");
}
}
Step 2: Creating the Facade Class
Now, let’s build the simplified Facade class:
public class HolidayFacade {
private readonly FlightBooking _flight;
private readonly HotelBooking _hotel;
private readonly CarRental _car;
public HolidayFacade() {
_flight = new FlightBooking();
_hotel = new HotelBooking();
_car = new CarRental();
}
public void BookHoliday(string origin, string destination, int nights) {
Console.WriteLine("Starting holiday booking...");
_flight.BookFlight(origin, destination);
_hotel.BookHotel(destination, nights);
_car.RentCar(destination, nights);
Console.WriteLine("Holiday booked successfully!");
}
}
Step 3: Using the Facade (Client)
Now, your client code becomes beautifully simple:
class Program {
static void Main() {
var holidayFacade = new HolidayFacade();
holidayFacade.BookHoliday("New York", "Paris", 5);
}
}
Output:
Starting holiday booking...
Booking flight from New York to Paris
Booking hotel in Paris for 5 nights
Renting car at Paris for 5 days
Holiday booked successfully!
See that simplicity? Pure magic!
Facade vs. Similar Patterns (Adapter, Decorator, Mediator)
Confused by similar patterns? Let’s clear it up:
- Facade vs. Adapter: Adapter changes the interface of one class into another that clients expect, while Facade simplifies multiple subsystem interactions without changing interfaces.
- Facade vs. Decorator: Decorator adds or modifies behavior dynamically, while Facade simplifies interactions without changing behaviors.
- Facade vs. Mediator: Mediator reduces chaotic interactions among multiple objects, while Facade primarily provides a simplified external interface.
Different Ways to Implement the Facade Pattern (With C# Examples)
Alright, let’s spice things up! There’s no one-size-fits-all Facade—you can implement it in several different ways based on your needs. Here are a few tasty variations, complete with easy-to-understand C# examples:
1. Simple Facade (Standard Implementation)
This is the most common scenario, exactly like we’ve just implemented above.
Example (quick recap):
public class SimpleFacade
{
private SubsystemA _subsystemA = new SubsystemA();
private SubsystemB _subsystemB = new SubsystemB();
public void DoSomething()
{
_subsystemA.OperationA();
_subsystemB.OperationB();
}
}
Simple, effective, and easy. It’s the pizza Margherita of Facades.
2. Parameterized Facade (Flexible Facade)
Sometimes your facade needs a bit of flexibility—like customizing toppings on your pizza.
Example:
public class FlexibleFacade
{
public void Execute(bool includeSubsystemB)
{
var subsystemA = new SubsystemA();
subsystemA.OperationA();
if (includeSubsystemB)
{
var subsystemB = new SubsystemB();
subsystemB.OperationB();
}
}
}
Here, the client gets more control without worrying about details.
3. Static Facade
If you want global, quick access without object instantiation, the Static Facade comes to the rescue!
Example:
public static class StaticFacade
{
private static readonly SubsystemA subsystemA = new SubsystemA();
private static readonly SubsystemB subsystemB = new SubsystemB();
public static void Execute()
{
subsystemA.OperationA();
subsystemB.OperationB();
}
}
Use it wisely! Static Facades are handy but beware of hidden state or dependencies lurking behind.
Real-World Use Cases of the Facade Pattern
Ever wonder where else you could use a Facade pattern in the real world? Let’s see some everyday examples:
1. E-commerce Checkouts:
Think Amazon or Shopify. Clicking “Buy Now” initiates multiple processes—payment, inventory update, shipping, notification emails—all seamlessly hidden behind a facade.
2. Media Player APIs:
Ever used video libraries or audio players? You’re interacting with a facade that handles codecs, streams, buffers, and audio/video synchronization.
3. Cloud Services:
Using cloud SDKs like Azure Storage SDK or AWS SDK? Each one is a facade simplifying a complex network of API calls, storage management, and authentication.
4. Complex ERP Systems:
Enterprise systems often expose simplified facades for daily tasks like payroll, scheduling, and HR operations, hiding vast complexity.
Anti-patterns to Avoid (Don’t Go Down This Road!)
The Facade pattern is awesome, but misuse can quickly turn it into a nightmare. Let’s dodge these anti-pattern bullets:
1. The “God Facade”:
- Problem: One facade tries to handle everything, becoming enormous and hard to maintain.
- Example (BAD!):
public class GodFacade
{
public void DoEverything()
{
// Flights, hotels, payments, notifications, inventory management...
}
}
- Solution: Divide into specialized facades—keep responsibilities clear and focused.
2. Leaky Facade:
- Problem: Exposing subsystem internals or requiring subsystem knowledge.
- Example (BAD!):
public class LeakyFacade
{
public SubsystemA GetSubsystemA() { return new SubsystemA(); }
}
- Solution: Ensure facades hide complexity completely. Clients should remain unaware of subsystem details.
3. Over-abstracting:
- Problem: Too many layers of facades complicate rather than simplify.
- Solution: Keep it straightforward. Only abstract when genuinely beneficial.
Anti-patterns to Avoid (Quick Reinforcement)
Yes, it’s important enough to say it again!
- God Facade (too broad)
- Leaky Facade (not encapsulating enough)
- Over-abstraction (layers upon layers)
Avoid these, and your Facade implementation stays clean and efficient.
Advantages of Using the Facade Pattern
Let’s briefly celebrate the great things about the facade pattern:
- Reduced Complexity: A simplified interface makes life easy.
- Improved Readability: Clearly outlines high-level operations.
- Better Maintainability: Decouples subsystems, making future changes painless.
- Quicker Integration: Facades help integrate external or legacy systems smoothly.
- Testing Simplicity: Easier to write tests against facades rather than complicated subsystems.
Disadvantages of Using the Facade Pattern
But nothing’s perfect, right? Let’s quickly touch upon some downsides:
- Risk of Oversimplification: Sometimes important details get lost or hidden.
- “Black Box” Syndrome: Developers may ignore subsystem details entirely, causing issues later.
- Performance Concerns: If not designed carefully, facades might unintentionally introduce overhead or performance bottlenecks.
Conclusion – Wrapping It Up!
Whew! You’ve journeyed through the world of the Facade Design Pattern. You now have the superpower to tame complex subsystems and messy integrations using clear, friendly, and effective facades.
Let’s quickly recap your newfound powers:
- You know exactly when and why to use the Facade.
- You’ve got different implementation styles under your belt.
- You’re aware of use cases that perfectly suit the Facade.
- You’re mindful of potential pitfalls—especially those nasty anti-patterns.
- And finally, you’re equipped to weigh the advantages and disadvantages carefully.
So next time complexity knocks on your door, you’ll confidently reply:
“Not today, chaos—I’ve got a Facade.”
Happy architecting, my friend, and remember—keep your code simple, friendly, and always understandable!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →