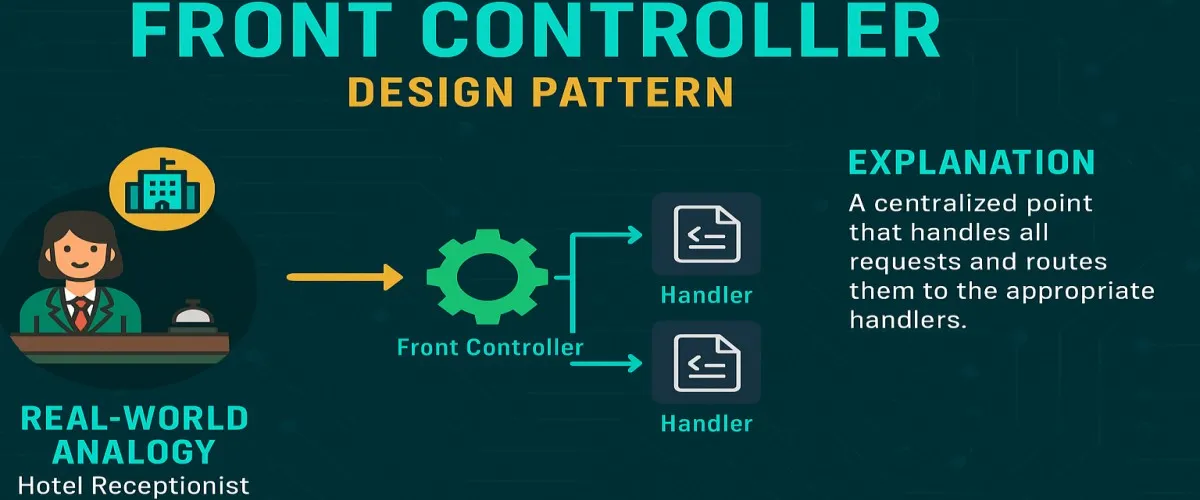
Front Controller Design Pattern in C#: The Ultimate Guide
Ever had to change something small in your app, only to realize you’ve got to hunt through dozens of different pages just to tweak a single line of logic? Yep, we’ve all been there. If this sounds like your daily struggle, it’s time you met your new best friend: the Front Controller design pattern.
In this article, you’ll get the full scoop on the Front Controller pattern—what it is, why it’s a big deal, and exactly how to implement it using good old C#. By the end, you’ll understand this pattern so deeply you’ll wonder how you ever managed without it.
1. What Exactly Is the Front Controller Pattern?
Ever visited a hotel where all requests—from room service to laundry—go through the front desk? That’s exactly how a Front Controller pattern works. It’s a single entry point for handling all user requests in your application.
Instead of scattering your request-handling logic all over your app, you funnel it through a single object, known as the Front Controller. This bad boy then delegates the request to the appropriate handler or controller.
Imagine this scenario:
- Without Front Controller: Your web requests go straight to individual pages or controllers scattered all over your application. It’s like guests directly barging into different rooms—chaotic and tough to manage!
- With Front Controller: Every request first meets our Front Controller at the “front desk.” The controller figures out exactly where to send the request next. It’s clean, efficient, and easy to maintain.
2. Principles Behind the Front Controller
The Front Controller is guided by some simple yet powerful principles:
- Centralized Control: All requests pass through one place, making managing and updating your app a breeze.
- Separation of Concerns: Request handling logic is separated from your business logic. Imagine each department doing its specific job—keeping things neat and organized.
- Single Entry Point: There’s only one entry point into the app. No more random entry points to chase down.
3. When Should You Use It?
Still not sure if you really need a Front Controller? Ask yourself these questions:
- Are you building a large application with lots of controllers?
- Is maintaining and scaling your app a priority?
- Do you want better control over request handling?
If you nodded at these questions, it’s time to meet the Front Controller pattern.
4. Key Components of a Front Controller
Let’s quickly break down the players involved:
- Front Controller: The single entry point for all incoming requests.
- Dispatcher: Responsible for routing the requests to specific controllers or handlers.
- View/Handler Controllers: These are the guys who actually handle the request and prepare the response.
- Views: Responsible for displaying data or results to users.
Think of the Front Controller like your receptionist, the Dispatcher as the concierge who points guests to the right department, and handlers as specialists who handle specific tasks.
5. Implementing a Front Controller in C#: Step-by-Step Guide
Let’s dive straight into action with an easy-to-follow yet detailed C# example.
Scenario:
You’re building a basic ASP.NET Core web application to manage requests centrally.
Step 1: Create the Front Controller
Here’s a simplified Front Controller in C#:
// FrontController.cs
public class FrontController
{
private Dispatcher dispatcher;
public FrontController()
{
dispatcher = new Dispatcher();
}
public void HandleRequest(string requestUrl)
{
Console.WriteLine($"Front Controller received request: {requestUrl}");
dispatcher.Dispatch(requestUrl);
}
}
Step 2: The Dispatcher
// Dispatcher.cs
public class Dispatcher
{
private HomeController homeController;
private AccountController accountController;
public Dispatcher()
{
homeController = new HomeController();
accountController = new AccountController();
}
public void Dispatch(string requestUrl)
{
if (requestUrl.Equals("/home"))
homeController.Handle();
else if (requestUrl.Equals("/account"))
accountController.Handle();
else
Console.WriteLine("404: Page not found!");
}
}
Step 3: The Controllers
Let’s create simple handlers:
// HomeController.cs
public class HomeController
{
public void Handle()
{
Console.WriteLine("Home Controller: Handling home request...");
}
}
// AccountController.cs
public class AccountController
{
public void Handle()
{
Console.WriteLine("Account Controller: Handling account request...");
}
}
Step 4: Testing the Setup
Let’s test our simple implementation:
// Program.cs
class Program
{
static void Main(string[] args)
{
FrontController frontController = new FrontController();
frontController.HandleRequest("/home"); // Output: Home Controller handling...
frontController.HandleRequest("/account"); // Output: Account Controller handling...
frontController.HandleRequest("/invalid"); // Output: 404 Page not found!
}
}
Congrats! You just implemented your first Front Controller in C#.
6. Real-Life Example: Building a Web Application in ASP.NET Core
In ASP.NET Core, the Front Controller pattern is already implemented for you (sweet!). Here’s how:
- Startup.cs handles routing setup.
- Middleware serves as your dispatcher.
- Controllers and Actions are your handlers.
Quick ASP.NET Core example:
// Startup.cs
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
The built-in routing middleware acts as your dispatcher, sending requests to controllers and actions.
7. Best Practices & Tips
- Keep your Front Controller lean—avoid business logic.
- Implement robust error handling in your dispatcher.
- Utilize dependency injection (DI) to simplify managing controllers.
- Regularly test your controller logic separately from the Front Controller.
8. Different Ways to Implement the Front Controller Pattern (With C# Examples)
There’s no one-size-fits-all when it comes to design patterns—especially in the world of Microsoft technologies. Let’s look at different ways you can implement the Front Controller pattern in C# to fit your project’s unique needs.
Method 1: Custom HTTP Handler (ASP.NET Framework)
Back in the classic ASP.NET days, custom HTTP handlers were a popular way to implement a Front Controller:
Example Implementation:
// FrontControllerHandler.cs
public class FrontControllerHandler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
var requestUrl = context.Request.Url.AbsolutePath;
switch (requestUrl)
{
case "/home":
new HomeController().Handle(context);
break;
case "/about":
new AboutController().Handle(context);
break;
default:
context.Response.StatusCode = 404;
context.Response.Write("Page Not Found");
break;
}
}
public bool IsReusable => false;
}
// Controller Example
public class HomeController
{
public void Handle(HttpContext context)
{
context.Response.Write("Welcome to Home!");
}
}
When to use?
- Legacy ASP.NET apps needing a centralized request processor.
Method 2: Middleware (ASP.NET Core Recommended)
In ASP.NET Core, Middleware simplifies the implementation:
Example Implementation:
// FrontControllerMiddleware.cs
public class FrontControllerMiddleware
{
private readonly RequestDelegate _next;
public FrontControllerMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
var path = context.Request.Path.Value;
if (path == "/home")
{
await context.Response.WriteAsync("This is the Home page.");
}
else if (path == "/about")
{
await context.Response.WriteAsync("This is the About page.");
}
else
{
await _next(context);
}
}
}
// Register Middleware in Startup.cs
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseMiddleware<FrontControllerMiddleware>();
app.Run(async context =>
{
await context.Response.WriteAsync("Default Response from App");
});
}
When to use?
- Modern applications, especially those built with ASP.NET Core.
9. Real-World Use Cases for Front Controller Pattern
Wondering if Front Controller fits your situation? Check these scenarios:
- Enterprise-level web applications:
- Centralized security, logging, and routing.
- API Gateways:
- Single entry point for multiple microservices.
- E-commerce Applications:
- Centralized control of routing and session management.
- CMS and Blogging Platforms:
- Simplified content routing and user management.
Basically, whenever central control and maintainability are critical, Front Controller is your go-to pattern.
10. Anti-patterns to Avoid with Front Controller
While Front Controller is awesome, there are ways you might accidentally misuse it:
Overloaded Front Controller:
Don’t let your Front Controller do everything—routing, handling, validation, etc. This breaks Separation of Concerns.
Avoid:
// Avoid this!
if (path == "/login") { ValidateUser(); Authenticate(); Log(); SendEmail(); }
Tight Coupling with Controllers:
Don’t hard-code every possible route directly inside your Front Controller.
Avoid:
// Avoid hard-coded routes
if (requestUrl == "/admin/settings/updatepassword") { /* Complex logic here */ }
Better Solution:
Use a Dispatcher or Routing middleware.
Mega Dispatcher Syndrome:
Your dispatcher shouldn’t be overloaded with business logic.
Ignoring Middleware or Filters:
Not leveraging built-in middleware (ASP.NET Core) or action filters (ASP.NET MVC) can lead to unnecessary complexity.
11. Advantages of the Front Controller Pattern
Here’s why architects love the Front Controller:
- Single Point of Control: Easy to manage, debug, and enhance your system.
- Security: Centralized security checks reduce vulnerabilities.
- Reusability: Common functionality like logging, error handling, or authentication can be reused across controllers.
- Simplified Maintenance: Changes in request processing logic affect just one place.
- Reduced Duplication: Centralizing common logic reduces redundancy.
12. Disadvantages of the Front Controller Pattern
But wait—there’s no free lunch in software design. Watch out for these:
- Single Point of Failure: If the Front Controller breaks, the entire application could fail.
- Complexity Overhead: Can become complicated if implemented incorrectly, leading to a confusing system.
- Performance Risks: Mismanagement of the dispatcher could introduce latency or bottlenecks.
**13. Conclusion: Wrapping it All Up **
So here we are—after our journey through the world of the Front Controller pattern in C#. Hopefully, by now, you’re seeing why this pattern is such a staple for serious architects working in the Microsoft ecosystem.
Remember these key points:
- Keep it simple: Use Front Controller to centralize handling but delegate specialized logic to handlers.
- Balance: Avoid making the Front Controller a jack-of-all-trades.
- Choose wisely: Middleware for ASP.NET Core, HTTP Handlers for legacy ASP.NET, or custom solutions depending on your project’s needs.
The Front Controller pattern is like having the ultimate receptionist—someone who always knows exactly where each guest (request) needs to go, keeps the chaos under control, and makes your architecture clean, efficient, and maintainable.
So go ahead—give your next Microsoft-based project the solid foundation it deserves!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →