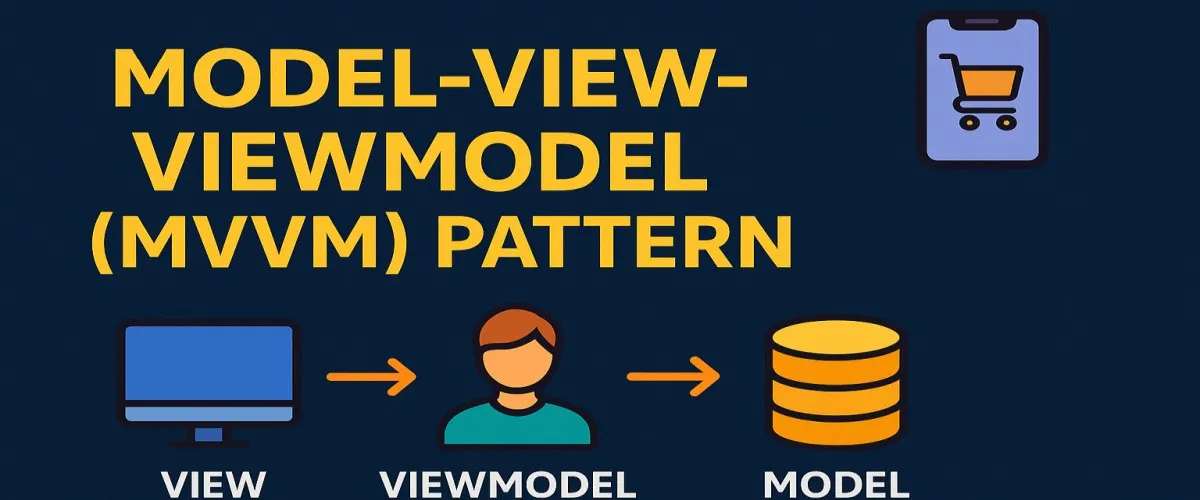
Dive into the Model-View-ViewModel (MVVM) Pattern: Your Ultimate Guide in C#
- Sudhir mangla
- Design Patterns
- 14 Apr, 2025
Hey there, software architects and developers! Ever felt like your application’s codebase is slowly turning into spaghetti, making it harder to maintain and scale? Relax—you’re not alone, and thankfully, there’s a solution! Enter the MVVM (Model-View-ViewModel) pattern.
In this article, we’ll dive deep into the MVVM pattern, understand its core principles, explore exactly when to use it (and when not to), highlight its key components, and wrap it all up with a detailed, practical implementation using C# and Microsoft technologies. By the end, you’ll be a bona fide MVVM ninja, ready to refactor your applications with ease.
So, buckle up, and let’s jump right in!
What Exactly is MVVM?
MVVM stands for Model-View-ViewModel, a design pattern created specifically to simplify the creation and maintenance of user interfaces, especially in platforms like WPF, Xamarin, MAUI, or UWP. Originally developed by Microsoft engineers to support WPF and Silverlight, MVVM emphasizes separating responsibilities, making your applications easier to develop, test, and maintain.
Imagine building software as being similar to constructing a house. You wouldn’t mix plumbing and electrical wiring, right? Similarly, in MVVM, your UI logic, business logic, and data handling are kept in clearly defined layers. It’s all about separation of concerns, making each part manageable and clear.
Core Principles of MVVM (Let’s Keep it Simple!)
MVVM is based around three fundamental principles:
1. Separation of Concerns
Your UI (View) doesn’t interact directly with your data model. Instead, you have a dedicated intermediary (ViewModel) that translates the model data for the View. Think of the ViewModel as a translator, making sure your model and UI speak the same language.
2. Data Binding
Data Binding is MVVM’s secret sauce. It’s the automatic synchronization between your UI (View) and your data (ViewModel). Change your data, and voila, the UI updates automatically—like magic! (Well, more like smart glue than magic.)
3. Testability
With MVVM, your logic stays out of your UI code. This makes it straightforward to write automated tests for your ViewModel without dealing with messy UI elements. Goodbye manual UI tests, hello reliable unit tests!
When to Use MVVM (and When Not to)
Alright, MVVM sounds awesome, but should you always use it? Not necessarily. Let’s see when MVVM shines and when you might want something simpler:
✅ Use MVVM when:
- You’re working with UI-intensive applications (especially WPF, Xamarin, UWP, or MAUI).
- You need clear, maintainable, and scalable code.
- You plan to use automated unit testing.
- You’re working in teams where responsibilities must be clearly separated.
❌ Avoid MVVM when:
- Your application is extremely simple and short-lived.
- You’re building non-UI applications, like backend services.
- You’re in a hurry to build a quick prototype (but even then, beware of technical debt!).
Key Components of MVVM: Meet Your New Best Friends
The MVVM pattern revolves around three core players:
- Model
- View
- ViewModel
🧩 1. The Model
Your Model represents the business data and logic of your app. It’s unaware of any UI specifics. Think of it as your raw, real-world data, completely independent of how it’s displayed.
🖼️ 2. The View
The View is simply the UI component. In MVVM, the View is lightweight and “dumb.” It’s responsible only for presenting data and capturing user interactions. It doesn’t do any heavy lifting or decision-making.
🚦 3. The ViewModel
The ViewModel bridges the gap between the View and the Model. It transforms data from the Model into a format the View can easily present and interact with. This is your application’s brain—handling commands, interaction logic, state management, and notifications to the UI.
Getting Our Hands Dirty: A Deep-Dive MVVM Implementation in C#
Now, let’s shift gears from theory to practice. We’ll create a practical, step-by-step implementation using C# and WPF (the classic MVVM playground).
🛠️ Project Setup:
First, fire up Visual Studio and create a new WPF project named MVVMExample
.
📁 Project Structure:
Let’s structure our project neatly into these folders:
MVVMExample
│
├── Models
├── ViewModels
├── Views
└── Commands
✅ Step-by-step Implementation:
Let’s create an MVVM-driven app—a simple “Task List Application.”
Step 1: Define the Model
Create TaskItem.cs
in Models
:
// Models/TaskItem.cs
namespace MVVMExample.Models
{
public class TaskItem
{
public string Title { get; set; }
public bool IsCompleted { get; set; }
}
}
Step 2: Define the ViewModel with INotifyPropertyChanged
Your ViewModel needs to implement INotifyPropertyChanged
to tell the View when data changes:
// ViewModels/TaskViewModel.cs
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Runtime.CompilerServices;
using MVVMExample.Models;
namespace MVVMExample.ViewModels
{
public class TaskViewModel : INotifyPropertyChanged
{
private string _newTaskTitle;
public ObservableCollection<TaskItem> Tasks { get; set; }
public string NewTaskTitle
{
get => _newTaskTitle;
set
{
_newTaskTitle = value;
OnPropertyChanged();
}
}
public RelayCommand AddTaskCommand { get; }
public TaskViewModel()
{
Tasks = new ObservableCollection<TaskItem>();
AddTaskCommand = new RelayCommand(AddTask);
}
private void AddTask(object parameter)
{
if (!string.IsNullOrWhiteSpace(NewTaskTitle))
{
Tasks.Add(new TaskItem { Title = NewTaskTitle, IsCompleted = false });
NewTaskTitle = string.Empty;
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
Step 3: Create a RelayCommand Class
A simple implementation of ICommand for MVVM:
// Commands/RelayCommand.cs
using System;
using System.Windows.Input;
namespace MVVMExample.Commands
{
public class RelayCommand : ICommand
{
private readonly Action<object> execute;
private readonly Func<object, bool> canExecute;
public RelayCommand(Action<object> execute, Func<object, bool> canExecute = null)
{
this.execute = execute;
this.canExecute = canExecute;
}
public bool CanExecute(object parameter) => canExecute == null || canExecute(parameter);
public void Execute(object parameter) => execute(parameter);
public event EventHandler CanExecuteChanged
{
add => CommandManager.RequerySuggested += value;
remove => CommandManager.RequerySuggested -= value;
}
}
}
Step 4: Implement the View
Finally, update your main window XAML (MainWindow.xaml
) to bind to your ViewModel:
<Window x:Class="MVVMExample.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MVVM Task List" Height="350" Width="400">
<Grid Margin="10">
<StackPanel>
<TextBox Text="{Binding NewTaskTitle, UpdateSourceTrigger=PropertyChanged}"
Width="300" Margin="0,0,0,10"/>
<Button Content="Add Task" Command="{Binding AddTaskCommand}" Width="100"/>
<ListBox ItemsSource="{Binding Tasks}" Margin="0,10,0,0">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<CheckBox IsChecked="{Binding IsCompleted}" Margin="0,0,10,0"/>
<TextBlock Text="{Binding Title}"/>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</StackPanel>
</Grid>
</Window>
Don’t forget to set the DataContext in MainWindow.xaml.cs
:
// MainWindow.xaml.cs
public MainWindow()
{
InitializeComponent();
DataContext = new TaskViewModel();
}
Different Ways to Implement MVVM (With C# Examples)
Alright, so you’ve learned the basics of MVVM. But did you know there’s more than one way to implement MVVM in C#? Let’s explore two popular approaches: using a MVVM framework and implementing MVVM manually.
1. Implementing MVVM Manually (Vanilla MVVM)
Sometimes, simplicity is best. With this method, you control every line of your MVVM implementation—perfect for learning the ropes or building smaller apps.
Let’s check out a quick example of manual MVVM:
ViewModel without Frameworks:
using System.ComponentModel;
using System.Runtime.CompilerServices;
namespace SimpleMVVM.ViewModels
{
public class SimpleViewModel : INotifyPropertyChanged
{
private string _message;
public string Message
{
get => _message;
set
{
_message = value;
OnPropertyChanged();
}
}
public SimpleViewModel()
{
Message = "Hello, MVVM!";
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string name = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(name));
}
}
}
Here, you directly implement INotifyPropertyChanged
. Simple and straightforward!
View (XAML):
<TextBlock Text="{Binding Message}" FontSize="24"/>
That’s it—no external frameworks, just pure MVVM magic. Great for small projects!
2. Implementing MVVM Using MVVM Frameworks
When your project grows, frameworks like MVVM Light, Prism, or Caliburn.Micro become invaluable. They handle repetitive tasks, simplify command handling, navigation, and more. Let’s see how MVVM Light Toolkit simplifies things:
Installation:
Use NuGet to install MVVM Light:
Install-Package MvvmLightLibs
MVVM with MVVM Light:
using GalaSoft.MvvmLight;
namespace FrameworkMVVM.ViewModels
{
public class FrameworkViewModel : ViewModelBase
{
private string _message;
public string Message
{
get => _message;
set => Set(ref _message, value);
}
public FrameworkViewModel()
{
Message = "MVVM with MVVM Light!";
}
}
}
ViewModelBase
automatically implements INotifyPropertyChanged
and simplifies your life significantly!
Common MVVM Use Cases (Where MVVM Shines Bright!)
MVVM isn’t just fancy architecture; it solves real-world problems! Here’s when MVVM really shines:
-
Enterprise Apps: If your application demands maintainability, scalability, and testability, MVVM is your superhero.
-
Cross-Platform Mobile Apps (Xamarin or MAUI): MVVM simplifies UI logic sharing across multiple platforms, saving you tons of development time.
-
Complex User Interfaces: Rich interactions and frequent UI updates? MVVM’s data-binding mechanism helps keep complexity manageable.
-
Test-Driven Development (TDD): If you love unit tests (and you should!), MVVM allows you to test logic without complicated UI setups.
MVVM Anti-Patterns: Avoid These Pitfalls!
MVVM is great, but beware these common anti-patterns. If you spot these, run:
🚫 Overstuffed ViewModels
If your ViewModel does too much (like database operations or heavy logic), it’s no longer just a translator—it becomes bloated.
Solution: Keep ViewModels lean. Move heavy logic into services or models.
🚫 View Knows the Model
When your View directly references the Model, MVVM breaks down.
Solution: Always interact with models through ViewModels. No cheating!
🚫 Ignoring Commands
Avoid triggering actions from event handlers directly in the View’s code-behind.
Solution: Use ICommand
implementations (RelayCommand) to delegate tasks to the ViewModel.
Advantages of MVVM: Why You’ll Love It
MVVM isn’t just another buzzword—it truly makes your coding life easier. Check out these perks:
-
✅ Clean Separation of Concerns
UI, logic, and data handling are distinct, organized layers. No more tangled code! -
✅ Enhanced Testability
ViewModels are easy to unit test. Goodbye manual UI tests, hello quick unit tests. -
✅ Code Reusability and Maintainability
Reusable ViewModels across different views or platforms save time and effort. -
✅ Simplified UI Development (Data Binding)
Automatic updates between ViewModel and View simplify UI logic tremendously. -
✅ Better Scalability
Growing apps stay organized, manageable, and maintainable.
Disadvantages of MVVM: Not a Silver Bullet!
But wait—MVVM isn’t perfect. Consider these challenges before diving in headfirst:
-
❌ Initial Learning Curve
Beginners might struggle at first due to abstract concepts. -
❌ Debugging Complexity
Data binding and automatic updates sometimes obscure the flow, complicating debugging. -
❌ Potential Overengineering
For tiny, simple apps, MVVM may feel like bringing a sledgehammer to crack a nut. -
❌ Performance Concerns
Heavy reliance on data binding might impact performance slightly in very complex UI scenarios.
MVVM Anti-Patterns (Bonus Reminder): Seriously, Avoid These!
(Yes, worth mentioning again because they’re common!)
- 🚫 Code-behind Mania: Excessive code in View’s code-behind breaks MVVM principles.
- 🚫 Model in View: Never bind directly to Models from your View.
- 🚫 ViewModel Knows the View: Your ViewModel shouldn’t reference any View directly.
- 🚫 Heavy Logic in ViewModels: ViewModel is a presenter—not the logic master!
Conclusion: Is MVVM Right for You?
Phew! You made it through a comprehensive MVVM journey. By now, you’re probably wondering: “Should I use MVVM?”
Here’s the short answer:
- Yes, if you value maintainable, testable, scalable UI applications.
- Maybe not, if your project is extremely small, quick-and-dirty, or non-UI driven.
Ultimately, MVVM helps you write cleaner, clearer, and easier-to-maintain code—especially when working within Microsoft ecosystems like WPF, Xamarin, or MAUI.
So go ahead, start refactoring your spaghetti code, apply MVVM principles, and thank yourself later. Your future self (and your teammates!) will appreciate your effort!
Now, go conquer your next MVVM-driven project!