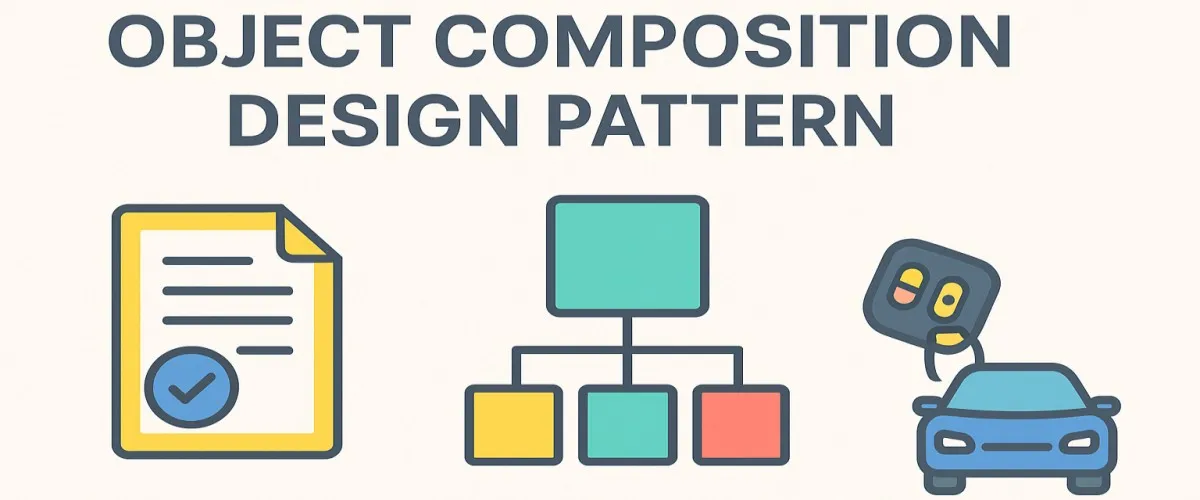
Mastering the Object Composition Design Pattern in C#: The Architect’s Guide
- Sudhir mangla
- Design Patterns
- 15 Apr, 2025
Ever wonder how Lego blocks effortlessly snap together to create fantastic structures? You simply combine small, reusable pieces to build something bigger, flexible, and maintainable. Guess what? That’s exactly what the Object Composition design pattern does for software architecture! If you’re a software architect deep into Microsoft Technologies (think C#, .NET, Azure), this guide is your new best friend.
Grab your coffee ☕, settle into your comfy chair 🪑, and get ready to level-up your architecture skills!
🔥 1. What Exactly is the Object Composition Pattern?
Object Composition is a design pattern that combines smaller, simpler objects into more complex structures. Instead of relying heavily on inheritance, it emphasizes assembling your classes from multiple parts (objects). Think of inheritance like baking a cake (fixed recipe, hard to modify) versus object composition like assembling a sandwich (flexible, easy to change ingredients).
🍕 Inheritance vs Composition (Quick Recap!)
- Inheritance: “Is-a” relationship. (e.g., a Car is-a Vehicle)
- Composition: “Has-a” relationship. (e.g., a Car has-an Engine, has-wheels, has-seats)
🤔 Why Composition?
Composition allows flexibility, making your systems easy to understand, extend, and maintain. It’s like building with Legos: just swap out bricks when you need changes without smashing everything apart!
🌟 2. Core Principles of Object Composition
Let’s get philosophical for a second. Understanding these key principles helps you become a better architect:
1. Favor Composition Over Inheritance
- Don’t inherit your problems! Composition keeps classes focused and reusable. When changes occur, you modify or replace a part without touching unrelated code.
2. Single Responsibility Principle (SRP)
- Each class should have only one reason to change. Composition helps by clearly separating responsibilities into dedicated classes.
3. Open-Closed Principle (OCP)
- Your classes should be open for extension but closed for modification. Composition enables adding new features without changing existing code—like plugging new accessories into your phone without opening it up!
4. Dependency Inversion Principle (DIP)
- Depend on abstractions, not concrete implementations. Composition leverages interfaces to decouple dependencies, increasing flexibility.
🚦 3. When Should You Use Object Composition?
Composition isn’t always the right tool, but it’s often the most effective. Use it when:
- You need flexibility and dynamic behavior.
- You prefer loosely-coupled components.
- You’re building complex systems from simpler reusable parts.
- You want to easily swap or extend functionality without heavy refactoring.
- Your codebase demands maintainability and scalability (who doesn’t?).
Example scenario:
Building an e-commerce site? YourShoppingCart
object can “have” payment methods, discounts, and items—all easily interchangeable via composition.
🛠️ 4. Key Components of Object Composition
Before diving into code, let’s break down essential parts of the pattern clearly:
✔️ Component (Abstract Interface)
Defines the abstraction or contract. It’s your blueprint—how components interact without worrying about specifics.
✔️ Leaf (Concrete Component)
Actual implementation classes that fulfill specific tasks.
✔️ Composite (Container Component)
Composed objects that aggregate multiple components to create complex structures.
⚡ 5. Deep Dive Implementation (with a REAL C# Example)
Let’s stop talking and start coding! We’ll build a realistic, detailed example to deeply understand how composition works using Microsoft’s favorite child—C#!
📚 Scenario: Building a Notification System
Imagine creating a notification system for a large enterprise app. Employees need alerts via email, SMS, or even push notifications, depending on their preferences.
🧩 Step 1: Define the Component Interface
public interface INotifier
{
void Send(string message);
}
This simple interface defines a common action every notifier must perform: sending a message.
🧩 Step 2: Implement Leaf Components (Concrete Notifiers)
Let’s make concrete implementations for each notification type:
Email Notifier:
public class EmailNotifier : INotifier
{
private string emailAddress;
public EmailNotifier(string email)
{
emailAddress = email;
}
public void Send(string message)
{
Console.WriteLine($"📧 Sending EMAIL to {emailAddress}: {message}");
// SMTP logic here...
}
}
SMS Notifier:
public class SmsNotifier : INotifier
{
private string phoneNumber;
public SmsNotifier(string phone)
{
phoneNumber = phone;
}
public void Send(string message)
{
Console.WriteLine($"📱 Sending SMS to {phoneNumber}: {message}");
// SMS gateway logic here...
}
}
Push Notification:
public class PushNotifier : INotifier
{
private string deviceId;
public PushNotifier(string device)
{
deviceId = device;
}
public void Send(string message)
{
Console.WriteLine($"📲 Sending PUSH notification to device {deviceId}: {message}");
// Push notification logic here...
}
}
🧩 Step 3: Implementing the Composite Component
Now let’s build a composite class (NotifierComposite
) to manage multiple notification channels simultaneously!
public class NotifierComposite : INotifier
{
private List<INotifier> notifiers = new List<INotifier>();
public void AddNotifier(INotifier notifier)
{
notifiers.Add(notifier);
}
public void RemoveNotifier(INotifier notifier)
{
notifiers.Remove(notifier);
}
public void Send(string message)
{
foreach(var notifier in notifiers)
{
notifier.Send(message);
}
}
}
🧩 Step 4: Putting it All Together (Client Code)
Now, here’s how your client code easily combines these:
class Program
{
static void Main(string[] args)
{
var emailNotifier = new EmailNotifier("user@example.com");
var smsNotifier = new SmsNotifier("+123456789");
var pushNotifier = new PushNotifier("device123");
var compositeNotifier = new NotifierComposite();
compositeNotifier.AddNotifier(emailNotifier);
compositeNotifier.AddNotifier(smsNotifier);
compositeNotifier.AddNotifier(pushNotifier);
compositeNotifier.Send("Your order has shipped! 🎉");
}
}
🧩 Output:
📧 Sending EMAIL to user@example.com: Your order has shipped! 🎉
📱 Sending SMS to +123456789: Your order has shipped! 🎉
📲 Sending PUSH notification to device device123: Your order has shipped! 🎉
💡 Why This Example Rocks!
- Decoupled: Change your notification logic independently.
- Extendable: Easily add new notification types like Slack, Teams, or WhatsApp.
- Maintainable: Single responsibility principle adhered beautifully. Changes in SMS don’t impact Email!
🚧 6. Different Ways to Implement Object Composition (with C# Examples)
Now that you’re familiar with object composition basics, let’s dig into different implementation strategies. Yep—there’s more than one way to snap those Lego blocks together!
Here are three popular composition strategies with C# examples:
🌀 1. Aggregation (Weak “has-a” relationship)
Aggregation is when your composed object uses other objects, but each can exist independently. Think of aggregation like a friendship: you hang out together, but each of you still lives separate lives.
Example: A Department
has Employees
, but if the department closes, employees still exist.
public class Employee
{
public string Name { get; set; }
public Employee(string name)
{
Name = name;
}
}
public class Department
{
private List<Employee> employees = new List<Employee>();
public void AddEmployee(Employee emp)
{
employees.Add(emp);
}
public void RemoveEmployee(Employee emp)
{
employees.Remove(emp);
}
public void DisplayEmployees()
{
Console.WriteLine("🏢 Department Employees:");
foreach (var emp in employees)
{
Console.WriteLine($"👤 {emp.Name}");
}
}
}
🧲 2. Composition (Strong “has-a” relationship)
Composition represents a stronger relationship where the life of child objects is tied to the parent object. If the parent is destroyed, children vanish too! It’s like organs in your body—without you, no heartbeat!
Example: An Engine
exists only as part of a Car
.
public class Engine
{
public void Start() => Console.WriteLine("🚗 Engine starts...");
}
public class Car
{
private Engine engine;
public Car()
{
engine = new Engine(); // Created inside Car constructor
}
public void StartCar()
{
engine.Start();
Console.WriteLine("🚘 Car started!");
}
}
⚙️ 3. Dependency Injection (DI)
Dependency Injection (DI) provides objects their dependencies from external sources, promoting flexibility and easy testing.
Example: Injecting logging dependencies in services.
public interface ILogger
{
void Log(string message);
}
public class ConsoleLogger : ILogger
{
public void Log(string message)
{
Console.WriteLine($"🔍 LOG: {message}");
}
}
public class OrderProcessor
{
private readonly ILogger logger;
public OrderProcessor(ILogger logger) // Dependency injection here!
{
this.logger = logger;
}
public void ProcessOrder(int orderId)
{
// Process order logic...
logger.Log($"Order {orderId} processed successfully.");
}
}
Usage:
var logger = new ConsoleLogger();
var processor = new OrderProcessor(logger);
processor.ProcessOrder(101);
🎯 7. Use Cases for Object Composition
Wondering where to use object composition effectively? Here’s your cheat sheet:
- Plugin Architectures: Dynamically adding features without modifying core code.
- UI Components: Building UI from modular, reusable widgets.
- Gaming: Entities composed dynamically (a player has-a weapon, armor, skills).
- Workflow Systems: Complex tasks composed of smaller, reusable operations.
- Middleware Pipelines: ASP.NET middleware components composed for flexibility.
🚫 8. Anti-patterns to Avoid (Composition Pitfalls)
Even Lego experts make mistakes. Avoid these common composition pitfalls:
⚠️ 1. God Object
- Issue: One massive object trying to do everything (violates SRP).
- Solution: Break responsibilities down into focused, composed components.
⚠️ 2. Tight Coupling
- Issue: Components that directly reference each other tightly.
- Solution: Always depend on abstractions (interfaces), never concrete implementations.
⚠️ 3. Deeply Nested Composition
- Issue: Objects composed of excessively nested layers—hard to manage.
- Solution: Limit nesting levels; use flatter, modular structures.
⚠️ 4. Overcomposition (Excessive Granularity)
- Issue: Breaking every small behavior into tiny components can cause complexity.
- Solution: Strike balance—compose logically related responsibilities only.
🎉 9. Advantages of Object Composition
Let’s quickly highlight why you’ll love object composition:
- ✅ Flexibility: Swap, remove, or add parts without hassle.
- ✅ Reusability: Reuse simple components across multiple systems.
- ✅ Maintainability: Easy to debug, maintain, and update independently.
- ✅ Testability: Easier unit testing due to clearly defined responsibilities.
- ✅ Dynamic Behavior: Easily adjust functionality at runtime.
🥲 10. Disadvantages of Object Composition
Composition isn’t flawless (though it’s close!). Consider these trade-offs:
- ❌ Complex Initialization: Sometimes requires more setup and configuration.
- ❌ Performance Overhead: Can introduce slight performance hits from extra layers.
- ❌ Indirection Complexity: Excessive abstraction can reduce readability for newcomers.
- ❌ Management Complexity: Lots of small components can be challenging to manage if not handled carefully.
🔄 11. Anti-patterns Revisited (More Pitfalls!)
Because repeating makes perfect! Here’s a quick reminder of what to avoid:
- Leaky Abstractions: Exposing implementation details through composed objects.
- Rigid Composition: Hard-coding dependencies instead of DI.
- Inappropriate Composition: Forcing composition where inheritance might actually be simpler.
Remember: Composition is powerful, but it’s not always the only tool in your toolbox!
🏁 12. Conclusion (Bringing It All Home!)
Congratulations! 🎉 You’ve reached the finish line. By now, you should have a solid understanding of how to leverage object composition effectively:
- You know composition vs. inheritance clearly.
- You’ve mastered different implementation strategies (aggregation, composition, DI).
- You’ve avoided composition anti-patterns (God objects, tight coupling).
- You’re aware of advantages and disadvantages, making informed architectural decisions.
Now, when you design your next enterprise-level C# project, think like a Lego master builder—compose your software from well-defined, reusable parts that are flexible, scalable, and easy to maintain.
**Go forth and compose amazing things!