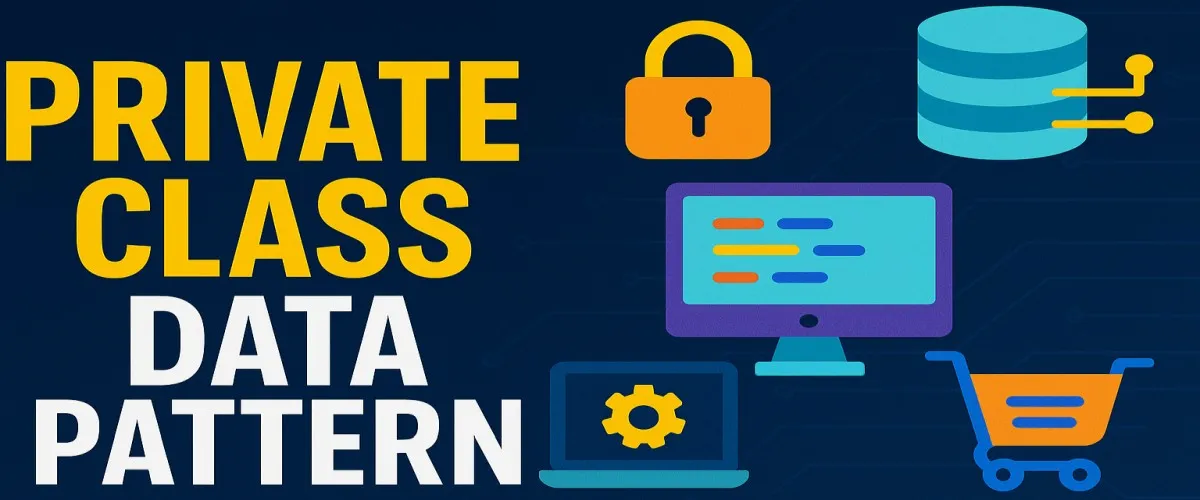
The Private Class Data Pattern: Keeping Your Classes Lean, Mean, and Clean (with C# Examples!)
Ever had a feeling your classes were sharing a bit too much information? Yeah, we’ve all been there. You start with a neat little class, add a few properties, sprinkle in some methods, and suddenly—it becomes the gossip center of your app. Every other class seems to know more about it than you’d like. But what if you could shut down the gossip entirely?
That’s exactly what the Private Class Data pattern is all about.
In this guide, we’ll deep-dive into one of the most underrated but highly effective design patterns. We’ll unpack it piece by piece, in plain, fun English, and demonstrate it thoroughly with crystal-clear, real-world examples in C#. By the time you’re done, you’ll wonder how you ever lived without it!
So, grab your coffee, lean back, and let’s get this show on the road!
What Is the Private Class Data Pattern Anyway?
Ever been to a restaurant and ordered your favorite dish? Ever noticed you never actually step into the kitchen to see exactly what goes into it? You just trust the chef. The Private Class Data pattern is exactly like that—except for your code.
Simply put, the Private Class Data pattern:
- Encapsulates the internal state of an object.
- Separates data from behavior within a class.
- Limits direct access to certain data, even from within the class itself, making sure no other class can accidentally (or maliciously!) tamper with it.
Think of it as locking your diary away. Sure, others know it exists, but only you have the key to its juicy secrets!
Principles of the Private Class Data Pattern
Every great pattern has a set of principles. Let’s break down the essential ones for this pattern:
1. Encapsulation is King
Encapsulation is the heart and soul of object-oriented design. It ensures that classes expose only what’s necessary and hide what’s sensitive or irrelevant.
2. Data Security
Private Class Data safeguards your critical class data, preventing accidental modification and misuse.
3. Single Responsibility
It separates data handling from behavioral logic, making your classes leaner and cleaner. It makes your life easier when debugging or adding new features.
4. Reducing Coupling
Fewer dependencies mean fewer headaches when changes occur. Classes become less sensitive to alterations in each other, promoting maintainability and flexibility.
When Should You Use the Private Class Data Pattern?
Great patterns don’t mean much unless you know when to use them, right? So when should you reach into your toolkit and grab this one?
Use the Private Class Data Pattern when:
- You need to protect your object’s internal data from accidental changes.
- Your class has sensitive data that must remain immutable after initialization.
- You notice too many methods directly accessing or modifying data fields.
- You have complex initialization logic that shouldn’t be modified once set.
Think of it like installing a security camera—you don’t necessarily need it everywhere, but for certain critical areas, you definitely want that extra bit of protection!
Key Components of the Private Class Data Pattern
Before we dive headfirst into our C# example, let’s quickly peek at the main ingredients needed to cook up this pattern:
- The Data Class (private): Holds the actual data, with fields usually marked as read-only to ensure immutability.
- The Wrapper Class (public): Provides controlled access and business logic, indirectly manipulating the private data without direct exposure.
Here’s a quick analogy:
- Data Class: The secret recipe stored safely in the vault.
- Wrapper Class: The trusted chef who has access to the recipe but doesn’t reveal its details.
Detailed Implementation with a Real-Life C# Example
Enough theory! Let’s roll up our sleeves and get our hands dirty. We’ll build a practical, fully working example using C#. By the end of this section, you’ll have an awesome understanding of how this pattern works in real-world code scenarios.
Example Scenario: Employee Class (Sensitive Salary Data)
Imagine you’re building an HR system. You’ve got employees, and each employee has sensitive salary data. You definitely don’t want every Tom, Dick, and Harry class modifying salaries directly. Perfect use case for our Private Class Data pattern!
Step 1: Without the Pattern (the Ugly )
First, let’s see how most developers would tackle this normally:
// The naive implementation
public class Employee
{
public string Name { get; set; }
public decimal Salary { get; set; }
public Employee(string name, decimal salary)
{
Name = name;
Salary = salary;
}
public void IncreaseSalary(decimal amount)
{
Salary += amount;
}
}
The problem? Nothing stops direct modification from anywhere. Not good.
Step 2: Introducing the Private Class Data Pattern (the Good )
We’ll split into two parts:
- Private Class: Holds sensitive data.
- Wrapper Class: Controls access.
The Private Data Class
// Step 1: Creating our locked-away, secret salary info
internal class EmployeeData
{
private readonly string _name;
private readonly decimal _salary;
public EmployeeData(string name, decimal salary)
{
_name = name;
_salary = salary;
}
public string Name => _name;
public decimal Salary => _salary;
// New instance creation to ensure immutability
public EmployeeData IncreaseSalary(decimal amount)
{
return new EmployeeData(_name, _salary + amount);
}
}
This class locks salary data away securely—once set, it’s permanent. To make changes, you create a new instance (immutability is key here!).
The Wrapper Class
public class Employee
{
private EmployeeData _employeeData;
public Employee(string name, decimal salary)
{
_employeeData = new EmployeeData(name, salary);
}
public string Name => _employeeData.Name;
public decimal Salary => _employeeData.Salary;
public void IncreaseSalary(decimal amount)
{
if (amount < 0)
throw new ArgumentException("Increase amount can't be negative!");
_employeeData = _employeeData.IncreaseSalary(amount);
}
}
Using Our Improved Employee Class
Here’s how you’d use this safer Employee class:
var employee = new Employee("John Doe", 50000m);
Console.WriteLine($"{employee.Name} earns {employee.Salary:C}");
employee.IncreaseSalary(5000m);
Console.WriteLine($"New Salary: {employee.Salary:C}");
The Magic Behind the Curtain
By separating data storage (EmployeeData
) from logic (Employee
), you’ve:
- Protected sensitive salary data from unintended modification.
- Ensured immutability and data integrity.
- Clearly separated responsibilities, making future updates simple.
Different Ways to Implement the Private Class Data Pattern (with C# Examples)
There’s always more than one way to slice a cake—or implement a design pattern. Let’s look at some popular approaches to implement the Private Class Data pattern in C#, so you can choose what works best for you.
Method 1: Immutable Private Data Class (Our Earlier Example Revisited)
You already saw this method. It’s ideal when your data should never change after initial creation.
Pros:
- Maximum data security.
- Immutable and thread-safe.
Cons:
- Slight overhead due to object creation when changes happen.
C# Quick Recap:
internal class PrivateData
{
private readonly int _secretNumber;
public PrivateData(int secretNumber)
{
_secretNumber = secretNumber;
}
public int SecretNumber => _secretNumber;
public PrivateData UpdateNumber(int newNumber) => new PrivateData(newNumber);
}
public class PublicWrapper
{
private PrivateData _privateData;
public PublicWrapper(int number)
{
_privateData = new PrivateData(number);
}
public int SecretNumber => _privateData.SecretNumber;
public void ChangeSecret(int newNumber)
{
_privateData = _privateData.UpdateNumber(newNumber);
}
}
Method 2: Mutable Private Data Class with Internal Methods
Sometimes, strict immutability can feel like overkill. If you need controlled changes without creating new objects each time, this one’s your go-to.
Pros:
- Better performance due to fewer object creations.
- Easier to manage simple updates.
Cons:
- Less secure compared to immutability, requires careful control.
Example in C#:
internal class MutableData
{
private int _secretNumber;
public MutableData(int number)
{
_secretNumber = number;
}
public int SecretNumber => _secretNumber;
internal void UpdateNumber(int number)
{
_secretNumber = number;
}
}
public class DataWrapper
{
private MutableData _data;
public DataWrapper(int number)
{
_data = new MutableData(number);
}
public int SecretNumber => _data.SecretNumber;
public void ChangeSecret(int number)
{
if (number < 0)
throw new ArgumentException("Number can't be negative!");
_data.UpdateNumber(number);
}
}
Here, you maintain some mutability internally but still keep data access tightly controlled.
Use Cases: Where Does Private Class Data Pattern Truly Shine?
This pattern might seem specialized, but you’d be surprised how many everyday scenarios benefit from it. Here are the best candidates:
Secure or Sensitive Data
- Banking applications (balances, transactions)
- HR and payroll systems (salary data, personal records)
️ Immutable State Management
- Versioning systems (document revisions)
- Game state snapshots (player health, stats)
Complex Initialization Logic
- Objects with expensive or intricate setup processes
- Configuration settings loaded once and never changed
Concurrency and Thread Safety
- Multi-threaded or asynchronous systems where data integrity matters most.
Think of this pattern as your digital safe—perfect when you have valuables you’d prefer others couldn’t tamper with.
Anti-Patterns to Avoid
Even great patterns can lead you astray if misused. Here’s how not to use Private Class Data:
Excessive Granularity
- Creating private classes for every small bit of data is like using a tank to crush peanuts—overkill and painful.
Breaking Encapsulation
- Exposing internal fields or properties publicly defeats the entire purpose of this pattern. It’s like hiding your valuables under a welcome mat marked “Valuables here!”
Confusing Immutability with Rigidity
- Forcing immutability on objects that naturally change frequently can cause unnecessary complexity and performance hits.
Advantages of the Private Class Data Pattern
Why should you fall in love with this pattern?
- Enhanced Data Security: Sensitive data stays hidden from direct tampering.
- Improved Encapsulation: Classes remain focused on behavior, not cluttered with direct data handling.
- Greater Maintainability: Easier debugging and fewer surprises down the road.
- Reduced Coupling: Classes don’t get tangled up in each other’s internal affairs.
- Clearer Intent: Makes code more readable and easier for developers to understand intentions quickly.
It’s like tidying up your room: Sure, it takes a bit of effort initially, but boy, does it feel great afterward!
Disadvantages of the Private Class Data Pattern ️
Okay, it’s powerful—but let’s face reality too:
- Added Complexity: Adds extra layers which may be unnecessary in simpler applications.
- Slight Performance Overhead: Immutability leads to new object creation, adding slight overhead.
- Potential Overuse: It’s easy to get carried away and apply this pattern where it’s just unnecessary.
- Learning Curve: Developers unfamiliar with this pattern might initially find it confusing.
Think of it as a high-security lock on your front door: excellent safety, but sometimes inconvenient if you’re in a hurry.
Anti-Patterns (Yes, Once More for Emphasis!)
Why again? Because it’s critical to avoid common pitfalls:
Overengineering
- Implementing it everywhere “just in case” leads to complexity without tangible benefits.
Data Exposure
- Accidentally exposing internal state defeats the entire purpose.
Ignoring Simplicity
- Simple problems rarely benefit from sophisticated solutions. Don’t complicate what works just fine already.
Avoid these, and you’ll keep the pattern working for you—not against you.
Quick Recap & Key Takeaways
- What is it? Protects internal state and promotes data immutability.
- Principles: Encapsulation, data security, single responsibility, reducing coupling.
- When to use? When sensitive data needs protection or immutability.
- Components: Data class (private), Wrapper class (public).
- Benefits: Improved security, maintainability, less complexity.
- Implementation: Clearly demonstrated via practical C# Employee class example.
Conclusion: Private Class Data—Your New Best Friend in Code
Wow, we’ve come a long way! You’ve learned everything from what the Private Class Data pattern is, to why and how you’d use it, complete with clear and detailed examples in C#.
Remember, the Private Class Data pattern is your secret weapon to:
- Secure sensitive data.
- Keep classes lean and purposeful.
- Improve readability and maintainability.
Sure, it’s not a one-size-fits-all solution—no pattern is—but when applied thoughtfully, it’s an absolute game-changer.
Now, go forth, keep your classes clean, your data secure, and your codebase maintainable. After all, great developers don’t just write code—they write code that keeps secrets!
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →