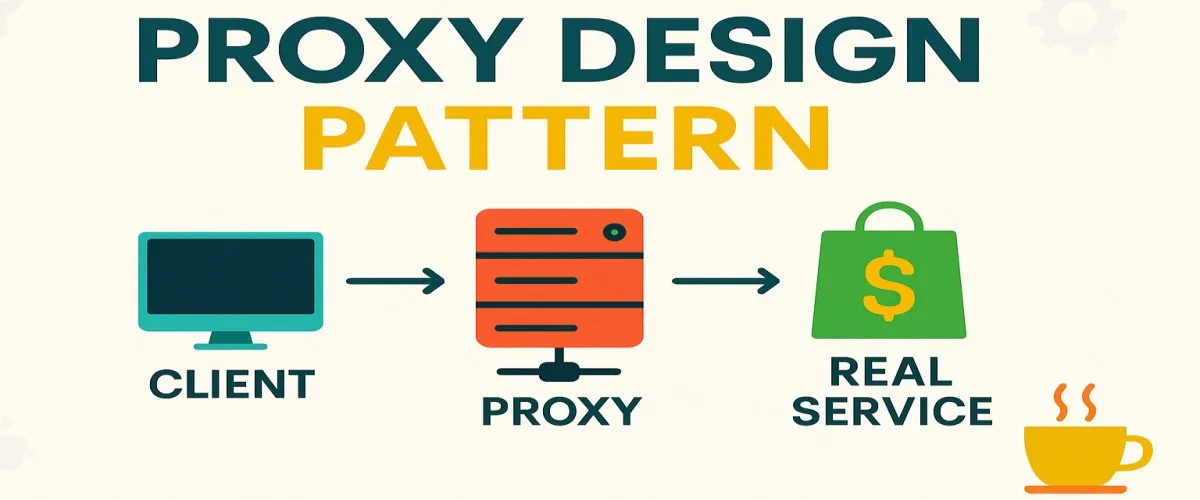
Proxy Design Pattern: Mastering the Art of Control in C#
“Ever felt like you needed a stunt double to handle the risky stuff in your application? Proxy Design Pattern does exactly that—stepping in, taking hits, and making sure your real objects stay safe and efficient.”
You’re a seasoned Microsoft technology architect (or aiming to be one!), and you need to keep your applications maintainable, robust, and efficient. Well, let me introduce you to your new best friend—the Proxy Design Pattern. Think of a proxy as your personal assistant—it helps control access, manage resources, or even optimize performance by standing in front of your actual objects.
Sounds exciting? Let’s dive in!
What Exactly Is the Proxy Design Pattern?
Before we get technical, imagine you’re the CEO of a big tech firm. Your office is constantly swamped by people—clients, reporters, employees—all wanting a piece of your attention. If you dealt with everyone directly, you’d never get any actual work done. So, what do you do? You hire an assistant to manage your meetings, screen your calls, and basically be the gatekeeper to your time. Your assistant proxies your interactions.
In software terms, the Proxy Design Pattern does the exact same thing. It creates an intermediary object (the proxy) to control or manage access to another object (the real subject).
Let’s break this down further:
- Proxy: Acts on behalf of another object. Controls access, manages additional responsibilities like logging, security, caching, or lazy loading.
- Real Subject: The actual object the proxy represents or manages access to.
- Client: Your application or any component that interacts with the proxy object instead of directly talking to the real subject.
A Quick Example:
Imagine a large video file that takes ages to load. Instead of loading it immediately (and slowing down your application), you create a proxy that loads the file only when necessary. This is known as Lazy Loading—one of the most common proxy use-cases.
Principles of the Proxy Pattern
Now that we’ve met our new best friend, let’s peek under the hood. The proxy pattern follows some essential principles:
1. Control and Protection
Your proxy acts as a gatekeeper. Just as your assistant filters your calendar, a proxy ensures objects are accessed correctly and securely.
2. Transparency
Your client should never know the difference between accessing a proxy or the real subject. As far as they’re concerned, they’re directly dealing with the original object.
3. Single Responsibility Principle (SRP)
Proxies ensure the main object doesn’t get overwhelmed with extra responsibilities. Let the proxy handle extra stuff like logging, caching, security, etc.
4. Decoupling and Encapsulation
A proxy separates the client from the real object, which helps keep the implementation details hidden and easily changeable later on.
When Should You Use a Proxy?
“Sounds neat,” you say, “but when exactly should I call in a proxy?” Great question! Let’s see a few common scenarios:
1. Virtual Proxy (Lazy Loading)
- When you want to defer object creation until it’s absolutely needed.
- For example, loading large images or videos only when required.
2. Protection Proxy
- To control access to sensitive or expensive resources based on access rights.
- Great for role-based security scenarios.
3. Remote Proxy
- If your object exists on a different server or machine, a remote proxy handles the complex communication (think WCF services or REST APIs).
4. Logging Proxy
- For adding logging, tracking usage, or monitoring actions without cluttering the main class logic.
5. Caching Proxy
- To cache expensive method calls or results, improving performance significantly.
Key Components of the Proxy Pattern
Every good pattern has its cast. Meet the actors of your proxy drama:
- Subject Interface: Defines methods implemented by both the real object and its proxy.
- Real Subject: Implements the actual business logic.
- Proxy Class: Controls access to the real subject. Typically implements the same interface as the real subject.
- Client: Interacts exclusively with the proxy.
Visualizing the Proxy Pattern:
Client --> Proxy --> RealSubject
Simple, right?
Implementing the Proxy Pattern in C#: Step-by-Step Deep Dive
Enough talk—let’s code! We’ll create a comprehensive, highly detailed example of a Virtual Proxy (Lazy Loading) to demonstrate clearly how this pattern works in practice.
Scenario:
Suppose you have an application that displays videos. Loading a video is expensive and slow. We’ll use a proxy to ensure videos only load when absolutely necessary.
Step 1: Define the Interface
public interface IVideo
{
void Play();
}
Simple and clean! This interface represents the common contract.
Step 2: Implement the Real Subject
Our real subject is RealVideo
. It represents a heavyweight object that actually loads and plays the video.
public class RealVideo : IVideo
{
private readonly string _fileName;
public RealVideo(string fileName)
{
_fileName = fileName;
LoadFromDisk();
}
private void LoadFromDisk()
{
Console.WriteLine($"Loading video '{_fileName}' from disk...");
Thread.Sleep(3000); // Simulate slow loading
Console.WriteLine("Video loaded!");
}
public void Play()
{
Console.WriteLine($"Playing video '{_fileName}'...");
}
}
Here, the video loads immediately upon creation—exactly what we want to avoid without the proxy.
Step 3: Implementing the Proxy Class
Here’s the magic. The proxy handles lazy loading by delaying the creation of the RealVideo
until it’s actually needed.
public class VideoProxy : IVideo
{
private RealVideo _realVideo;
private readonly string _fileName;
public VideoProxy(string fileName)
{
_fileName = fileName;
}
public void Play()
{
if (_realVideo == null)
{
Console.WriteLine("Initializing the real video object (lazy loading)...");
_realVideo = new RealVideo(_fileName);
}
_realVideo.Play();
}
}
Notice: The real video doesn’t load until you explicitly call Play()
. Clever, isn’t it?
Step 4: The Client—Putting It All Together
Let’s see our proxy in action:
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Creating proxy (doesn't load video yet)");
IVideo video = new VideoProxy("example_video.mp4");
Console.WriteLine("Video created. Hasn't loaded yet, right?\n");
Console.WriteLine("Now calling Play():");
video.Play();
Console.WriteLine("\nCalling Play() again—video should be already loaded:");
video.Play();
}
}
Output:
Creating proxy (doesn't load video yet)
Video created. Hasn't loaded yet, right?
Now calling Play():
Initializing the real video object (lazy loading)...
Loading video 'example_video.mp4' from disk...
Video loaded!
Playing video 'example_video.mp4'...
Calling Play() again—video should be already loaded:
Playing video 'example_video.mp4'...
Perfect! Lazy loading works beautifully. Notice how the second call to Play()
doesn’t reload the video. That’s efficiency!
Recap and Benefits
Here’s what we achieved with our Proxy Pattern:
- Performance boost through lazy loading.
- Clean, maintainable code with clear separation of concerns.
- Efficiency because resources load only when needed.
- Transparency—client code remains completely unaffected.
Different Ways to Implement the Proxy Pattern in C#
Now that we’ve got our feet wet, let’s explore a few more practical ways to implement proxies. Different proxies, different jobs—each with their unique style!
1. Protection Proxy (Security Proxy)
Imagine you’re throwing an exclusive party—only VIPs get through the door. A Protection Proxy works exactly like a bouncer at your club, ensuring only authorized users access your precious resources.
Implementation Example:
public interface IDatabase
{
void QueryData();
}
public class RealDatabase : IDatabase
{
public void QueryData()
{
Console.WriteLine("Fetching sensitive data from the database...");
}
}
public class ProtectionProxy : IDatabase
{
private RealDatabase _realDatabase;
private readonly string _userRole;
public ProtectionProxy(string userRole)
{
_userRole = userRole;
}
public void QueryData()
{
if (_userRole == "Admin")
{
if (_realDatabase == null)
_realDatabase = new RealDatabase();
_realDatabase.QueryData();
}
else
{
Console.WriteLine("Access Denied: Insufficient privileges!");
}
}
}
// Client usage
class Program
{
static void Main(string[] args)
{
IDatabase adminProxy = new ProtectionProxy("Admin");
adminProxy.QueryData(); // Access granted
IDatabase userProxy = new ProtectionProxy("Guest");
userProxy.QueryData(); // Access denied
}
}
2. Remote Proxy
If your objects live in another galaxy (or server!), the Remote Proxy will handle the tedious details of communication. Think WCF, RESTful APIs, and remote service calls.
Example Skeleton:
public interface IRemoteService
{
string GetData();
}
// Real subject (Remote server logic)
public class RemoteService : MarshalByRefObject, IRemoteService
{
public string GetData()
{
return "Data from remote service!";
}
}
// Proxy to remote object
public class RemoteProxy : IRemoteService
{
private readonly IRemoteService _remoteService;
public RemoteProxy(IRemoteService remoteService)
{
_remoteService = remoteService;
}
public string GetData()
{
Console.WriteLine("Calling remote service...");
return _remoteService.GetData();
}
}
(You’d typically set up the proxy using WCF or gRPC to communicate over a network.)
Real-world Use Cases for Proxy Patterns
“Got it,” you say. “But where exactly can I drop proxies in real life?”
1. Lazy-loading of Resources
- Loading large images/videos or database-heavy objects only when needed.
2. Security Enforcement
- Controlling access to sensitive resources (admin dashboards, financial systems).
3. Remote Method Invocation
- Communicating with objects across networks (think distributed systems or microservices).
4. Monitoring and Logging
- Track object access, monitor usage patterns, or audit trails.
5. Caching Expensive Operations
- Store and reuse previously fetched results to boost performance.
Anti-patterns to Avoid (Seriously, Don’t Do These!)
Now, let’s chat briefly about traps architects fall into with proxies:
1. Overusing Proxies (Proxy Hell!)
Ever heard, “too much of anything is bad”? It applies here. Use proxies only when you genuinely need them.
2. Non-transparent Proxies
A proxy should always be invisible to the client. If your proxy leaks implementation details, rethink your strategy!
3. Proxy Spaghetti
Don’t chain too many proxies together! Multiple layers of proxies can confuse the heck out of everyone (including future-you!).
Advantages of the Proxy Design Pattern
Let’s talk superpowers again:
- Control Access: Easily control or limit resource access.
- Performance Improvement: Lazy loading and caching improve speed significantly.
- Security Enforcement: Restrict access based on roles or permissions seamlessly.
- Transparency: Clients don’t need to be aware of proxies—life stays simple.
- Separation of Concerns: Keeps business logic clean by separating auxiliary tasks.
Disadvantages of Proxy Pattern (Yes, They’re There!)
Nothing is perfect, so let’s see some limitations:
- Increased Complexity: Extra classes might make understanding or debugging trickier for new devs.
- Possible Performance Hit: Misuse (or excessive chaining) can introduce overhead.
- Overhead of Maintaining Proxies: Keeping proxies and subjects synchronized adds a layer of complexity.
Anti-patterns to Avoid (Extra Emphasis, Because It’s Crucial!)
Wait, didn’t we cover anti-patterns already? Yes, but this is important enough to repeat:
1. The God Proxy (Doing Too Much!)
If your proxy becomes a Swiss Army Knife (handling logging, caching, security, lazy loading all at once), it’s time to refactor!
2. Confused Identity
A proxy should clearly represent exactly one object. If your proxy tries managing multiple unrelated objects, you’ll soon regret it.
3. Silent Failure
If your proxy silently swallows exceptions or hides errors, debugging becomes a nightmare. Let the errors bubble clearly.
Conclusion: Proxy Pattern—Your Secret Weapon!
We started this journey by asking, “Wouldn’t it be great if your code had a stunt double?” The Proxy Pattern is exactly that—a dedicated assistant, protector, and stand-in for your actual objects.
By now, you’ve got:
- A deep understanding of what proxy patterns are.
- Multiple implementation strategies in C#.
- Clear use cases from security to performance.
- Solid knowledge of advantages and disadvantages.
- Awareness of anti-patterns to avoid at all costs!
In short, proxies help you create code that is:
- Safer (protecting resources)
- Faster (lazy-loading and caching)
- Easier to maintain (clear separation of concerns)
So next time you’re tackling complex scenarios in your Microsoft stack, don’t forget the power of proxies. They’re like having your own private security team, personal assistant, and efficient cache manager—all in one tidy package.
Happy coding! You’ve just added another powerful pattern to your architect’s toolkit. Use it wisely, and watch your codebase thrive.
Share this article
Help others discover this content
About Sudhir mangla
Content creator and writer passionate about sharing knowledge and insights.
View all articles by Sudhir mangla →