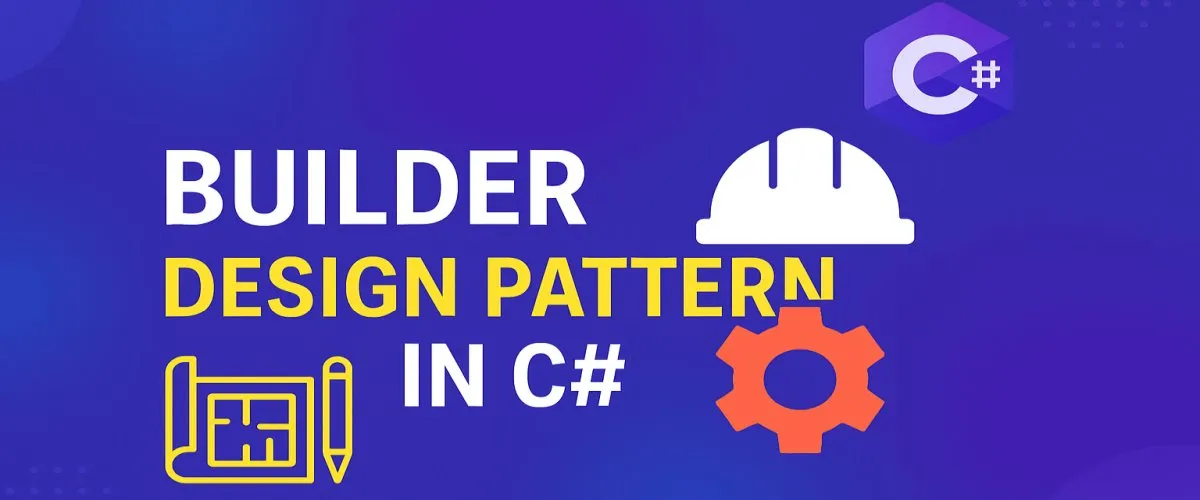
Mastering the Builder Design Pattern in C# — Simplifying Complex Object Construction!
- Sudhir mangla
- Design Patterns
- 24 Mar, 2025
Ever felt overwhelmed by complex object construction? Ever found yourself lost in a maze of overloaded constructors? Or worse—ending up with code so messy it makes spaghetti jealous? If yes, then you’re in the right place! Let’s dive into the Builder Design Pattern, a magical tool that can turn the chaos of object creation into something beautiful and easy.
🚧 What Exactly is the Builder Design Pattern?
Think of the Builder Design Pattern like your favorite sandwich shop. You walk in, and instead of yelling out your 15-ingredient sandwich all at once, you carefully choose each ingredient step-by-step—bread, veggies, meat, sauce, cheese—exactly how you like it. This pattern follows a similar philosophy: breaking down the process of building a complicated object into small, manageable steps.
The Builder pattern separates the construction of a complex object from its representation, letting you produce different representations of an object using the same construction process.
In other words, separation of concerns is the name of the game!
🧩 Principles Behind the Builder Pattern
Here’s what makes the Builder Design Pattern tick:
-
Single Responsibility Principle (SRP):
- Every class or module has only one reason to change. Builders handle object creation, and your main class doesn’t have to worry about it.
-
Open/Closed Principle (OCP):
- You can extend object construction without changing existing code.
-
Step-by-step construction:
- Objects are built incrementally. No overload soup here!
-
Separation of Construction and Representation:
- The client can create different representations with the same construction process.
🎯 When Should You Use the Builder Pattern?
Wondering when to whip out the Builder pattern? Here’s your checklist:
- ✅ Complex Object Creation: When your objects require numerous parameters or steps to construct.
- ✅ Readable and Maintainable Code: If readability is critical, the Builder is your friend.
- ✅ Multiple Representations: Need various ways to build the same object? Builder’s got your back.
- ✅ Avoiding Telescoping Constructors: If you find yourself drowning in constructor overloads—time to build!
📌 Key Components of the Builder Pattern
There are four main characters in this story:
- Product: The final object you want to create.
- Builder Interface: Defines methods to build parts of the product.
- Concrete Builder: Implements the builder interface, assembling the product.
- Director: Uses the builder interface to construct an object.
Let’s visualize it:
- 🎁 Product → The Sandwich
- 🧑🍳 Builder Interface → Menu listing the sandwich-building steps
- 👨🍳 Concrete Builder → Sandwich Artist
- 📢 Director → The Customer ordering a sandwich step-by-step
🛠️ Implementing the Builder Pattern with C# (Detailed Example)
Let’s create a real-world example—building a computer configuration. You’ll see the magic unfold clearly in C#.
Step 1: Define the Product (Computer)
// The product class
public class Computer
{
public string CPU { get; set; }
public string GPU { get; set; }
public string RAM { get; set; }
public string Storage { get; set; }
public override string ToString() =>
$"Computer [CPU={CPU}, GPU={GPU}, RAM={RAM}, Storage={Storage}]";
}
Step 2: Builder Interface
public interface IComputerBuilder
{
void BuildCPU();
void BuildGPU();
void BuildRAM();
void BuildStorage();
Computer GetComputer();
}
Step 3: Concrete Builder Implementation
// Gaming Computer Builder
public class GamingComputerBuilder : IComputerBuilder
{
private Computer _computer = new Computer();
public void BuildCPU() => _computer.CPU = "Intel i9-14900K";
public void BuildGPU() => _computer.GPU = "NVIDIA RTX 5090";
public void BuildRAM() => _computer.RAM = "64GB DDR5";
public void BuildStorage() => _computer.Storage = "2TB NVMe SSD";
public Computer GetComputer() => _computer;
}
Step 4: Director Class
public class ComputerDirector
{
public void ConstructComputer(IComputerBuilder builder)
{
builder.BuildCPU();
builder.BuildGPU();
builder.BuildRAM();
builder.BuildStorage();
}
}
Putting It All Together:
class Program
{
static void Main(string[] args)
{
ComputerDirector director = new ComputerDirector();
// Building a gaming computer
IComputerBuilder gamingBuilder = new GamingComputerBuilder();
director.ConstructComputer(gamingBuilder);
Computer gamingComputer = gamingBuilder.GetComputer();
Console.WriteLine(gamingComputer);
// Output: Computer [CPU=Intel i9-14900K, GPU=NVIDIA RTX 5090, RAM=64GB DDR5, Storage=2TB NVMe SSD]
}
}
See how clean and neat that was? Welcome to Builder-land!
🚧 Diving Deeper: Different Ways to Implement the Builder Pattern in C#
You’ve seen the basics, but let’s expand on three practical and powerful ways to implement the Builder Pattern in C#. This time, we’re diving deep, complete with detailed explanations and solid examples, so get comfortable!
🔸 Method 1: Fluent Builder (Method Chaining)
The Fluent Builder approach is about creating objects using readable, chained method calls. It’s like ordering at Starbucks: “I’ll have a tall, extra-hot latte with almond milk and caramel drizzle.”
Example Implementation:
// Fluent Builder for a Pizza
public class Pizza
{
public string Dough { get; set; }
public string Sauce { get; set; }
public string Cheese { get; set; }
public List<string> Toppings { get; set; } = new List<string>();
public override string ToString()
{
return $"Pizza [Dough={Dough}, Sauce={Sauce}, Cheese={Cheese}, Toppings={string.Join(", ", Toppings)}]";
}
}
public class PizzaBuilder
{
private Pizza _pizza = new Pizza();
public PizzaBuilder SetDough(string dough)
{
_pizza.Dough = dough;
return this;
}
public PizzaBuilder SetSauce(string sauce)
{
_pizza.Sauce = sauce;
return this;
}
public PizzaBuilder SetCheese(string cheese)
{
_pizza.Cheese = cheese;
return this;
}
public PizzaBuilder AddTopping(string topping)
{
_pizza.Toppings.Add(topping);
return this;
}
public Pizza Build()
{
return _pizza;
}
}
// Usage
var myPizza = new PizzaBuilder()
.SetDough("Thin crust")
.SetSauce("Tomato basil")
.SetCheese("Mozzarella")
.AddTopping("Olives")
.AddTopping("Mushrooms")
.Build();
Console.WriteLine(myPizza);
// Output: Pizza [Dough=Thin crust, Sauce=Tomato basil, Cheese=Mozzarella, Toppings=Olives, Mushrooms]
Pros:
- Highly readable.
- Easy to extend.
- Simple object creation process.
🔸 Method 2: Builder with Nested Inner Class
Here, your builder lives right inside the class it builds, like nesting dolls. It feels compact, clean, and self-contained.
Example Implementation:
// Inner class Builder for User
public class User
{
public string FirstName { get; private set; }
public string LastName { get; private set; }
public string Email { get; private set; }
public int Age { get; private set; }
private User() { }
public override string ToString()
{
return $"User [FirstName={FirstName}, LastName={LastName}, Email={Email}, Age={Age}]";
}
public class Builder
{
private readonly User _user = new User();
public Builder WithFirstName(string firstName)
{
_user.FirstName = firstName;
return this;
}
public Builder WithLastName(string lastName)
{
_user.LastName = lastName;
return this;
}
public Builder WithEmail(string email)
{
_user.Email = email;
return this;
}
public Builder WithAge(int age)
{
_user.Age = age;
return this;
}
public User Build()
{
return _user;
}
}
}
// Usage
var user = new User.Builder()
.WithFirstName("Alice")
.WithLastName("Johnson")
.WithEmail("alice@example.com")
.WithAge(30)
.Build();
Console.WriteLine(user);
// Output: User [FirstName=Alice, LastName=Johnson, Email=alice@example.com, Age=30]
Pros:
- Encapsulated within the product class.
- Easy-to-understand structure.
- Self-documenting object creation.
🔸 Method 3: Abstract Builder Pattern with Director
This version adds more abstraction, perfect for scenarios needing multiple representations using a standard construction procedure. Imagine building houses with standardized processes but different blueprints.
Example Implementation:
// Abstract Product
public class House
{
public string Foundation { get; set; }
public string Structure { get; set; }
public string Roof { get; set; }
public override string ToString()
{
return $"House [Foundation={Foundation}, Structure={Structure}, Roof={Roof}]";
}
}
// Builder Interface
public interface IHouseBuilder
{
void BuildFoundation();
void BuildStructure();
void BuildRoof();
House GetHouse();
}
// Concrete Builders
public class WoodenHouseBuilder : IHouseBuilder
{
private House house = new House();
public void BuildFoundation() => house.Foundation = "Wooden Foundation";
public void BuildStructure() => house.Structure = "Wooden Structure";
public void BuildRoof() => house.Roof = "Wooden Roof";
public House GetHouse() => house;
}
public class BrickHouseBuilder : IHouseBuilder
{
private House house = new House();
public void BuildFoundation() => house.Foundation = "Concrete Foundation";
public void BuildStructure() => house.Structure = "Brick Structure";
public void BuildRoof() => house.Roof = "Tile Roof";
public House GetHouse() => house;
}
// Director
public class ConstructionDirector
{
public void ConstructHouse(IHouseBuilder builder)
{
builder.BuildFoundation();
builder.BuildStructure();
builder.BuildRoof();
}
}
// Usage
var director = new ConstructionDirector();
IHouseBuilder woodenBuilder = new WoodenHouseBuilder();
director.ConstructHouse(woodenBuilder);
House woodenHouse = woodenBuilder.GetHouse();
Console.WriteLine(woodenHouse);
// Output: House [Foundation=Wooden Foundation, Structure=Wooden Structure, Roof=Wooden Roof]
IHouseBuilder brickBuilder = new BrickHouseBuilder();
director.ConstructHouse(brickBuilder);
House brickHouse = brickBuilder.GetHouse();
Console.WriteLine(brickHouse);
// Output: House [Foundation=Concrete Foundation, Structure=Brick Structure, Roof=Tile Roof]
Pros:
- Supports multiple representations.
- Highly flexible and extendable.
- Ideal for scalable scenarios.
🚀 Detailed Use Cases of Builder Pattern
Here are some real-world scenarios to help visualize where the Builder Pattern truly shines:
-
1️⃣ Complex UI Building
- Creating customizable forms or views (e.g., WPF/XAML builders or fluent APIs in ASP.NET MVC).
-
2️⃣ Report Generation Systems
- Dynamically building complex reports with headers, footers, charts, and tables.
-
3️⃣ Query Builders
- Dynamically constructing SQL or LINQ queries based on user inputs.
-
4️⃣ Game Development
- Creating complex characters or game assets with numerous configurations, abilities, or attributes.
-
5️⃣ Document Processing Applications
- Generating PDF, Word, or Excel files where each step (header, footer, content, images) is customizable.
-
6️⃣ Configuration Builders
- Creating configuration objects for applications or microservices with multiple parameters and defaults.
-
7️⃣ Test Data Generation
- Building mock data for testing scenarios, where data structures are complex and customizable.
-
8️⃣ SDKs and APIs
- Providing easy-to-use client APIs that require complex initialization and configuration steps.
🌟 Advantages of Using Builder Pattern
- ✅ Improved Readability: Step-by-step construction is easy to read and understand.
- ✅ Reduced Complexity: Avoids constructor overloads.
- ✅ Flexibility: Allows the same construction process to create different representations.
- ✅ Maintainability: Easy to modify or extend the builder without affecting existing code.
⚠️ Disadvantages of Builder Pattern
- ❌ Overhead: Introducing more classes can increase complexity for simple objects.
- ❌ Verbose: Sometimes it adds more code than direct constructors.
- ❌ Learning Curve: Initial understanding might be tricky for new developers.
But don’t worry—these disadvantages usually become trivial in complex scenarios!
🎓 Wrapping it Up: Why Builder Pattern is Your Next Best Friend
If you’ve survived the constructor jungle, the Builder pattern feels like a refreshing oasis. It lets you construct complex objects elegantly, maintainably, and flexibly—without losing your sanity.
Next time you face overwhelming constructors or object creation nightmares, ask yourself: “Isn’t there a simpler way to do this?” Then reach for your trusty Builder pattern toolkit and start building like a pro!