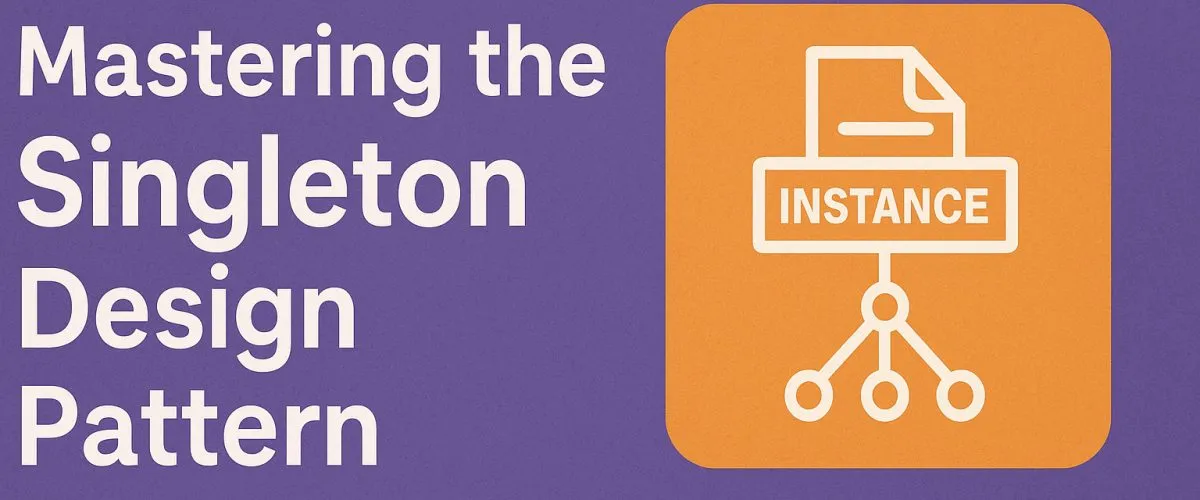
Mastering the Singleton Design Pattern in C#
- Sudhir mangla
- Design Patterns
- 22 Mar, 2025
Mastering the Singleton Design Pattern in C#
Hey there, fellow coder! Ever found yourself in a situation where you needed a class to have just one instance throughout your application? Enter the Singleton Design Pattern—a nifty solution to ensure a class has only one instance and provides a global point of access to it. Let’s dive deep into this pattern, unravel its principles, and explore how to implement it effectively in C#.
What is the Singleton Design Pattern?
Imagine you’re organizing a concert, and there’s only one backstage pass. No matter how many people want access, only one person can hold that pass at any given time. Similarly, the Singleton Pattern ensures that a class has only one instance and provides a global access point to that instance. It’s like having a single source of truth for certain operations or data in your application.
Principles Behind the Singleton Pattern
The Singleton Pattern is built on a few core principles:
- Single Instance: Ensure that only one instance of the class exists throughout the application’s lifecycle.
- Global Access Point: Provide a way to access this instance globally, so any part of the application can utilize it.
- Controlled Instantiation: Prevent external code from creating new instances of the class, typically by making the constructor private.
When to Use the Singleton Pattern
You might wonder, “When should I use a Singleton?” Here are some scenarios where it’s particularly useful:
- Configuration Management: When you have configuration settings that should be consistent across the application.
- Logging: To ensure all parts of your application write logs to the same logger instance.
- Resource Management: Managing access to resources that are expensive to create, like database connections or file handlers.
- Thread Pools: Controlling access to a pool of threads to manage concurrent operations efficiently.
Key Components of the Singleton Pattern
To implement a Singleton in C#, you’ll need:
- Private Constructor: Prevents external instantiation.
- Static Instance: Holds the single instance of the class.
- Public Static Method or Property: Provides global access to the instance.
Implementing Singleton in C#
Let’s roll up our sleeves and implement a Singleton in C#. We’ll start with a basic implementation and then explore thread-safe versions.
Basic Singleton Implementation
Here’s a straightforward way to implement a Singleton:
public sealed class Singleton
{
private static Singleton _instance = null;
// Private constructor ensures that an object is not instantiated from outside the class.
private Singleton() { }
// Public static method that returns the single instance of the class.
public static Singleton Instance
{
get
{
if (_instance == null)
{
_instance = new Singleton();
}
return _instance;
}
}
}
In this example:
- The constructor is private, so the class can’t be instantiated from outside.
- The
Instance
property checks if an instance already exists; if not, it creates one.
Thread-Safe Singleton Implementation
In a multithreaded environment, the basic implementation might lead to multiple instances. To make it thread-safe, you can use a lock
:
public sealed class Singleton
{
private static Singleton _instance = null;
private static readonly object _lock = new object();
private Singleton() { }
public static Singleton Instance
{
get
{
lock (_lock)
{
if (_instance == null)
{
_instance = new Singleton();
}
return _instance;
}
}
}
}
Here, the lock
ensures that only one thread can enter the critical section where the instance is created.
Thread-Safe Singleton with Double-Check Locking
For better performance, you can use double-check locking:
public sealed class Singleton
{
private static volatile Singleton _instance = null;
private static readonly object _lock = new object();
private Singleton() { }
public static Singleton Instance
{
get
{
if (_instance == null)
{
lock (_lock)
{
if (_instance == null)
{
_instance = new Singleton();
}
}
}
return _instance;
}
}
}
This approach reduces the overhead of acquiring a lock by first checking if the instance exists before locking.
Using Lazy<T>
for Lazy Initialization
C# provides the Lazy<T>
type, which makes implementing a lazy-loaded, thread-safe Singleton straightforward:
public sealed class Singleton
{
private static readonly Lazy<Singleton> _instance = new Lazy<Singleton>(() => new Singleton());
private Singleton() { }
public static Singleton Instance => _instance.Value;
}
With Lazy<T>
, the instance is created only when it’s first accessed, and the Lazy<T>
type ensures thread safety.
Different Ways to Implement Singleton
Beyond the implementations we’ve discussed, there are other variations:
-
Eager Initialization: The instance is created at the time of class loading. This is simple but might be unnecessary if the instance is never used.
public sealed class Singleton { private static readonly Singleton _instance = new Singleton(); private Singleton() { } public static Singleton Instance => _instance; }
-
Static Constructors: Utilize a static constructor to initialize the instance. The CLR ensures that static constructors are thread-safe.
public sealed class Singleton { private static readonly Singleton _instance; static Singleton() { _instance = new Singleton(); } private Singleton() { } public static Singleton Instance => _instance; }
Use Cases for Singleton Pattern
Let’s look at some real-world scenarios where the Singleton Pattern shines:
-
Configuration Settings: Centralizing configuration settings ensures consistency across the application. A Singleton can load settings once and provide access throughout the app.
-
Logging: A single logging instance ensures that all components log messages uniformly, and log files aren’t corrupted by concurrent writes.
-
Database Connections: Managing a single database connection instance can control access to the database and manage connection pooling effectively.
-
Caching: A Singleton can manage a cache of data that’s expensive to fetch or compute, improving application performance.
Advantages of the Singleton Pattern
- Controlled Access to Instance: The class has control over the instantiation process, ensuring only one instance exists.
- Reduced Namespace Pollution: Since the instance is accessed via a static method or property, it doesn’t clutter the global namespace.
- Permits Refinement of Operations and Representations: The Singleton class can be extended, and its operations can be refined without affecting other parts of the application.
- Saves Memory: By preventing the creation of multiple instances, it conserves memory, especially when the instance holds a significant amount of state.
Disadvantages of the Singleton Pattern
- Hidden Dependencies: Since the Singleton instance is globally accessible, it can introduce hidden dependencies across the application, making the code harder to understand and test.
- Difficulty in Unit Testing: Singletons can make unit testing challenging because they introduce global state into an application, which can lead to tests that are dependent on each other.
- Potential for Resource Contention: In multithreaded applications, if not implemented correctly, Singletons
Conclusion
The Singleton Pattern is a powerful tool when used wisely. It offers controlled, consistent access to shared resources across your application. While it has some drawbacks, understanding its principles and proper implementation in C# can help you write cleaner, more efficient, and maintainable code. Use it with intention and care!