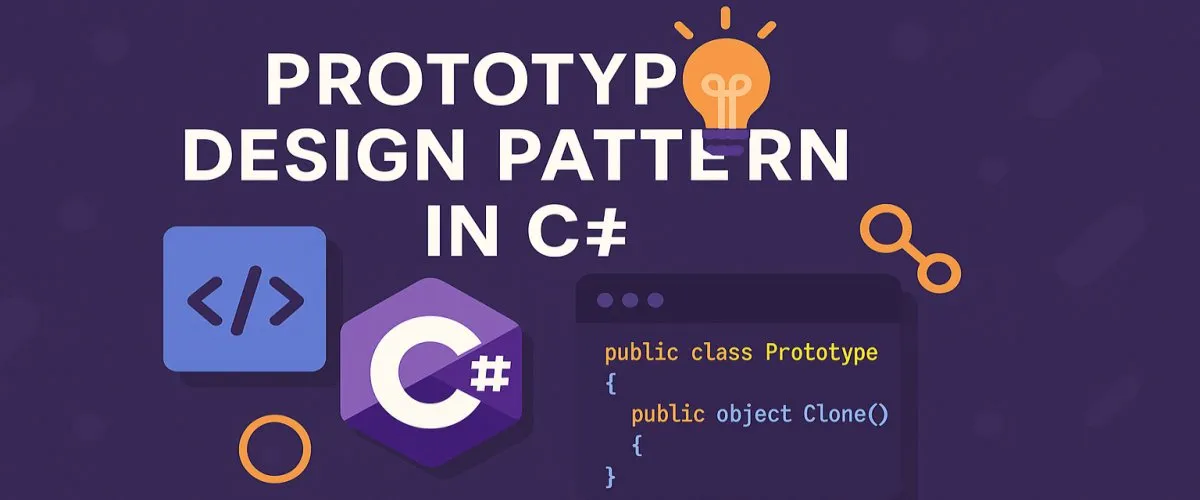
Mastering the Prototype Design Pattern in C#: Cloning Your Way to Cleaner Code
- Sudhir mangla
- Design Patterns
- 25 Mar, 2025
Ever had that moment where you wished you could just make a quick copy of an object without dealing with its messy initialization logic all over again? Yeah, me too. That’s exactly where the Prototype Design Pattern shines. Think of it like your handy photocopy machine, churning out exact copies of complex documents at the push of a button. Only this time, it’s objects we’re cloning—and it’s all happening in your beloved C#.
So, buckle up! We’re going deep into the Prototype Pattern, and by the end of this guide, you’ll know exactly when and how to use it, complete with crystal-clear C# examples. You’ll also be fully equipped to avoid common pitfalls and write cleaner, maintainable code that’s easy to understand, extend, and reuse.
Let’s get started!
What Exactly is the Prototype Design Pattern?
Let’s start with the basics. Prototype is a creational design pattern that lets you create new objects by copying an existing object, known as the “prototype,” rather than by calling constructors. Pretty simple, right? But don’t be fooled by its simplicity—this little gem can dramatically simplify your object creation logic.
Imagine you run a bakery. Baking cookies from scratch each time can be tiresome. But what if you had a magical cookie mold (the prototype) from which you could instantly clone perfect cookies whenever you needed them? That’s exactly what the Prototype pattern does for your code.
Here’s a simple analogy:
- Prototype object: Your original, fully-initialized “master cookie.”
- Cloning: Making quick, identical copies without going through the tedious baking process again.
Principles Behind the Prototype Pattern
The Prototype pattern operates on a few basic principles:
- Cloning over construction: Creating objects by copying existing instances rather than calling constructors repeatedly.
- Encapsulation of complexity: Simplifying object creation logic by hiding complex initialization behind prototypes.
- Reduced dependency: Objects created through cloning do not depend on specific classes or complex construction processes, making your system more flexible.
In short, Prototype simplifies your life by reusing existing objects to create new ones, reducing boilerplate and simplifying complex initialization.
When Should You Use the Prototype Pattern?
Good question! Here are some clear scenarios:
- Complex Object Initialization: If initializing your object is complicated, costly, or requires extensive computation, Prototype makes perfect sense.
- Limited Number of Object Variations: If you have a small set of possible object states or configurations, it’s easier to maintain prototypes rather than repeatedly configuring objects manually.
- Reducing Class Hierarchy Complexity: If subclassing for object variations results in a bloated class hierarchy, using Prototype simplifies object creation without endless subclasses.
- Improving Performance: Cloning can be much faster than repeatedly invoking constructors, especially when you’re dealing with objects loaded from external resources (like database queries or file I/O).
Key Components of the Prototype Pattern
Let’s break down the pattern into its core parts:
- Prototype Interface: Declares the cloning method, usually named something straightforward like
Clone()
. - Concrete Prototype: Implements the cloning operation.
- Client: Requests cloning from the prototype.
Think of the Prototype Interface as your cookie cutter—it defines what “copying” looks like. Concrete Prototypes are your actual cookie dough—these are the objects you’ll clone. Finally, the Client is like you, picking the cookie cutter and stamping out cookies on demand.
Implementing the Prototype Pattern in C#: Step-by-Step Example
Let’s dive straight into a real-world, clear-cut example.
Imagine you’re designing a game where different enemy characters have complex initializations, including appearance, behaviors, stats, and resources. Using the Prototype Pattern, you’ll set up a prototype enemy and clone it whenever a new instance is needed.
Step 1: Defining the Prototype Interface
public interface IEnemyPrototype
{
IEnemyPrototype Clone();
}
Step 2: Implementing the Concrete Prototype
public class Enemy : IEnemyPrototype
{
public string Name { get; set; }
public int Health { get; set; }
public string Weapon { get; set; }
// Imagine a complex constructor
public Enemy(string name, int health, string weapon)
{
Name = name;
Health = health;
Weapon = weapon;
// Complex initialization logic here...
}
// Clone method
public IEnemyPrototype Clone()
{
// Shallow copy (we'll discuss deep copy soon!)
return (IEnemyPrototype)this.MemberwiseClone();
}
public void Attack()
{
Console.WriteLine($"{Name} attacks with {Weapon}, Health: {Health}");
}
}
Step 3: Using the Prototype in the Client
public class GameClient
{
static void Main(string[] args)
{
Enemy goblinPrototype = new Enemy("Goblin", 100, "Sword");
Enemy goblin1 = (Enemy)goblinPrototype.Clone();
Enemy goblin2 = (Enemy)goblinPrototype.Clone();
goblin1.Attack();
goblin2.Attack();
}
}
Output:
Goblin attacks with Sword, Health: 100
Goblin attacks with Sword, Health: 100
Voila! Cloning made easy.
Deep Dive: Different Ways to Implement Prototype Cloning in C#
Hey, ready to get serious about cloning? Awesome! You’ve already dipped your toes into the basics, but now let’s go deeper and explore the different techniques for implementing Prototype cloning in C#. Understanding these will make you the Prototype Jedi Master of your team!
Let’s roll up our sleeves and explore three practical approaches:
1. Shallow Copy Cloning
Think of a shallow copy like taking a photocopy of a list of contacts. You’ll copy the list itself, but if the original has handwritten notes on separate sheets attached to it, your photocopy just references those same sheets rather than duplicating them. In C#, the simplest way to create a shallow copy is by using MemberwiseClone()
.
Pros and Cons:
- Pros: Quick and simple to implement, requires minimal code.
- Cons: Only copies references, not the actual objects. Changing nested objects affects both original and cloned instances.
C# Example:
public class Person : ICloneable
{
public string Name { get; set; }
public Address Address { get; set; } // nested reference object
public object Clone()
{
// This is a shallow copy!
return this.MemberwiseClone();
}
}
public class Address
{
public string Street { get; set; }
public string City { get; set; }
}
// Usage
var original = new Person
{
Name = "John",
Address = new Address { Street = "123 Main St", City = "Seattle" }
};
var clone = (Person)original.Clone();
clone.Name = "Jane";
clone.Address.City = "New York";
Console.WriteLine(original.Address.City); // Oops! Outputs "New York"
See that? Modifying the clone also changed the original’s nested object. Tricky!
2. Deep Copy via Manual Implementation
Sometimes you gotta roll your sleeves up and write the cloning logic yourself. Manual deep cloning is like carefully copying every detail from an old notebook to a new one—tedious but effective.
Pros and Cons:
- Pros: Complete control over cloning logic, very precise.
- Cons: Time-consuming, error-prone, harder to maintain if objects change frequently.
Detailed C# Example:
public class Person : ICloneable
{
public string Name { get; set; }
public Address Address { get; set; }
public object Clone()
{
// Manually deep copying each property
return new Person
{
Name = this.Name,
Address = new Address
{
Street = this.Address.Street,
City = this.Address.City
}
};
}
}
// Usage
var original = new Person
{
Name = "John",
Address = new Address { Street = "123 Main St", City = "Seattle" }
};
var clone = (Person)original.Clone();
clone.Address.City = "New York";
Console.WriteLine(original.Address.City); // Still "Seattle"—Perfect!
Much better, isn’t it? It’s more manual labor, but gives you precise control.
3. Deep Copy via Serialization
If manual deep copying seems overwhelming (and it often does), serialization is your trusty sidekick. It serializes your object into a format (JSON or binary), then instantly deserializes it back, giving you a perfect deep clone every time. Think of serialization like teleporting your object—it disappears from one place and appears perfectly duplicated somewhere else.
Pros and Cons:
- Pros: Easy to implement, especially for deeply nested objects. Automatically handles deep cloning.
- Cons: Slight performance overhead due to serialization/deserialization.
Detailed C# Example Using JSON:
using System.Text.Json;
public static class CloneHelper
{
public static T DeepClone<T>(this T obj)
{
var json = JsonSerializer.Serialize(obj);
return JsonSerializer.Deserialize<T>(json);
}
}
// Usage
var original = new Person
{
Name = "John",
Address = new Address { Street = "123 Main St", City = "Seattle" }
};
var clone = original.DeepClone();
clone.Address.City = "New York";
Console.WriteLine(original.Address.City); // Still "Seattle"—Nice!
Serialization clones deeply and reliably with minimal effort. Easy peasy!
Real-Life Use Cases of the Prototype Pattern
Now, let’s move beyond theory. When would you actually use this nifty pattern in the real world?
- Game Development: Spawn identical enemies or characters instantly by cloning a prototype. Imagine creating a legion of stormtroopers effortlessly!
- Graphic Design & Editors: Clone shapes, text boxes, or image objects. Think Adobe Illustrator’s “duplicate” feature—Prototype makes that happen seamlessly.
- Configuration Systems: Quickly copy and tweak settings for user preferences, application settings, or UI themes. Like duplicating presets in Photoshop.
- Document Editors: Rapidly clone content sections, styles, and formatting (Microsoft Word or Google Docs “copy formatting”).
- Simulation Software: Clone complex simulation scenarios to create slight variations without expensive recalculations or database calls.
Advantages of the Prototype Pattern
Prototype pattern isn’t just fancy—it’s practical and powerful. Check out these clear benefits:
- Performance Improvement: Cloning can be faster than repeatedly initializing complex objects from scratch.
- Simplified Object Creation: Hides complexity behind an easy-to-use prototype.
- Runtime Flexibility: Objects can be customized and duplicated at runtime without creating new classes.
- Reduced Class Explosion: Avoids creating numerous subclasses for minor variations, keeping your class hierarchy lean and clean.
Disadvantages of the Prototype Pattern
But wait, it’s not all sunshine and rainbows. Here’s the dark side of Prototype you should know about:
- Cloning Complexity: Deep cloning nested or complicated objects can introduce bugs or require careful maintenance.
- Memory Overhead: Cloning complex objects repeatedly might consume significant memory.
- Prototype Management Overhead: If your application grows, managing dozens of prototypes can become complicated.
- Potential for Hidden Dependencies: Clones might inadvertently share resources or references if deep copying isn’t properly handled.
Wrapping Up (Conclusion)
You made it! You’re now officially fluent in Prototype speak. Whether it’s shallow cloning (fast but tricky), manual deep copying (precise but verbose), or deep cloning via serialization (simple but resource-intensive), you’ve got the tools to pick the right approach.
Prototype isn’t just another fancy buzzword; it’s like a Swiss Army knife for complex object initialization and duplication. Sure, it’s not perfect—but then again, nothing in software ever truly is. By understanding its strengths and weaknesses, you can confidently wield Prototype to simplify your architecture, boost performance, and make your code easier to maintain.
Next time your code starts looking like spaghetti because of repetitive object creation logic, pause and ask yourself: “Can Prototype cloning save my sanity here?”
Happy coding, and may your clones always be bug-free!