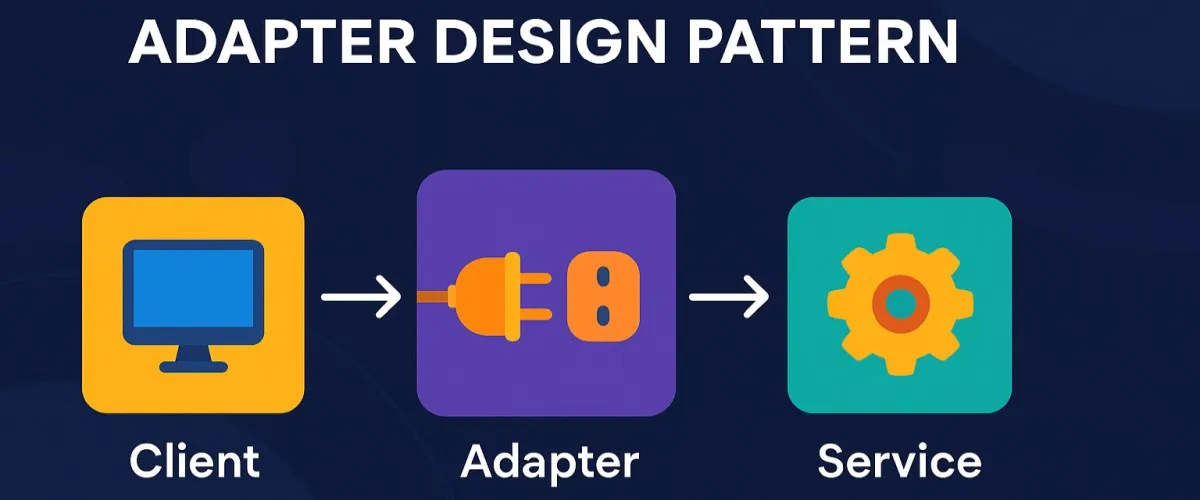
Adapter Design Pattern in C# | Master Incompatible Interfaces Integration
- Sudhir mangla
- Design Patterns
- 28 Mar, 2025
Ever tried plugging your laptop charger into an outlet in a foreign country without an adapter? It’s a frustrating experience! You have the device (your laptop) and the source (the power outlet), but without a proper adapter, nothing happens.
In the software world, we face similar problems. You have existing code, and you have new code—both perfectly good in isolation—but they refuse to talk to each other. So how do you make these different systems cooperate without rewriting entire applications?
Say hello to the Adapter Pattern! It’s like that handy travel adapter that lets you power your devices no matter where you are in the world.
This article is your one-stop shop for everything about the Adapter Design Pattern—specifically in C#, tailored for software architects working with Microsoft technologies. Grab a coffee, put on your coding hat, and let’s get adapting!
What Exactly is the Adapter Design Pattern?
In the simplest terms, the Adapter Pattern is a structural design pattern that allows two incompatible interfaces to work together without changing their existing code.
Imagine you’re integrating legacy software (think old-school ERP systems) with modern APIs or cloud services. The legacy system’s code can’t directly interact with your new fancy API. Instead of rewriting everything (a headache nobody wants), you use an adapter—a layer that bridges these two different worlds.
Principles Behind the Adapter Pattern (Why Does It Work So Well?)
The Adapter Pattern leverages key principles from SOLID—especially the “Open/Closed Principle,” which says:
“Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.”
Here’s how the Adapter Pattern respects this principle:
- Single Responsibility: The adapter handles only the task of converting interfaces.
- Open/Closed: Instead of changing existing code, you add a new adapter, leaving existing interfaces untouched.
- Interface Segregation: Adapters encourage minimal interfaces that clients actually use.
Think of the Adapter Pattern like hiring an interpreter at the UN. You don’t teach everyone a new language; instead, you bring in a specialist who understands both languages fluently.
When Should You Use the Adapter Pattern?
You should reach for the Adapter Pattern when:
- Integrating legacy code: You need to use old libraries or codebases with modern frameworks.
- Third-party libraries: Your system needs to interface with third-party APIs, whose interfaces you can’t (or don’t want to) change.
- Multiple implementations: You have several classes with incompatible interfaces, but you want to standardize them to a common interface.
Real-World Scenario:
Imagine you’re a software architect at a large enterprise using Microsoft tech. You have a legacy SOAP service, but your new code is built using modern RESTful APIs. Instead of rebuilding the entire legacy service, you build an adapter to seamlessly connect the two.
Key Components of the Adapter Pattern
Before jumping into code, let’s quickly understand the building blocks of the Adapter Pattern:
- Target Interface: Defines the domain-specific interface that clients use.
- Adaptee: The existing class that needs adapting (usually legacy or third-party code).
- Adapter: Implements the Target interface and internally uses the Adaptee, converting requests accordingly.
- Client: Interacts with objects through the Target interface.
Let’s illustrate this visually first:
Client → Target Interface → Adapter → Adaptee
Implementing the Adapter Pattern with a Detailed C# Example
Time to roll up your sleeves and get coding!
Scenario:
Suppose you’re developing a business application that processes payroll using a modern payroll interface. But your enterprise uses a legacy payroll system that doesn’t match the modern interface.
Step 1: Define the Target Interface
This is what your modern application expects to use.
public interface IPayrollProcessor
{
void ProcessPayroll(string employeeId, decimal salary);
}
Step 2: The Legacy (Adaptee) Class
This class exists in your legacy system, and you can’t modify its interface.
public class LegacyPayrollSystem
{
public void ExecutePayment(string empCode, double amount)
{
Console.WriteLine($"Legacy system: Payment of {amount:C} executed for employee {empCode}.");
}
}
Notice the mismatch (employeeId
vs empCode
, decimal
vs double
).
Step 3: Create the Adapter
This adapter will bridge the legacy system to your new interface.
public class PayrollAdapter : IPayrollProcessor
{
private readonly LegacyPayrollSystem _legacySystem;
public PayrollAdapter(LegacyPayrollSystem legacySystem)
{
_legacySystem = legacySystem;
}
public void ProcessPayroll(string employeeId, decimal salary)
{
// Convert decimal to double, and employeeId to legacy system format if needed
double legacySalary = Convert.ToDouble(salary);
_legacySystem.ExecutePayment(employeeId, legacySalary);
}
}
Step 4: Use the Adapter in Client Code
Here’s how your modern client code looks:
class Program
{
static void Main(string[] args)
{
IPayrollProcessor payrollProcessor = new PayrollAdapter(new LegacyPayrollSystem());
payrollProcessor.ProcessPayroll("E123", 4500.00m);
}
}
Output:
Legacy system: Payment of $4,500.00 executed for employee E123.
Bingo! Your old and new systems are now happily talking to each other.
Best Practices When Implementing the Adapter Pattern in C#
- Keep it Simple: Adapters should be lean—just enough code to make things work.
- Clearly Define Boundaries: It should be clear what is adapter code and what is core business logic.
- Exception Handling: Ensure adapters handle exceptions from adaptees gracefully.
- Performance Considerations: Be aware of performance overhead due to interface translation—usually negligible, but test your code thoroughly.
Common Pitfalls (and How to Avoid Them)
- Creating Unnecessary Complexity: Don’t use adapters everywhere—only when genuinely needed.
- Breaking Single Responsibility: Keep adapters solely focused on interface translation.
- Tight Coupling: Always depend on interfaces, not concrete implementations.
Adapter Pattern in Microsoft .NET Framework and Libraries
Microsoft itself frequently uses Adapter Patterns—think of DbDataAdapter
in ADO.NET:
DbDataAdapter
bridges betweenDataSet
and various databases. Whether SQL Server, Oracle, or MySQL, you only interact with a consistent interface. That’s the Adapter Pattern at its best!
Different Ways to Implement the Adapter Pattern in C#
You’re feeling pretty confident with your newfound adapter knowledge, but hold on—there’s always more than one way to build a bridge, right? Let’s look at two popular ways you can implement the Adapter Pattern in C#:
1. Object Adapter (Composition-based)
We already tackled this one, but let’s do a quick recap—this approach uses composition. The Adapter contains a reference to the Adaptee and implements the target interface.
Example (Object Adapter):
// Target Interface
public interface IModernPrinter
{
void Print(string content);
}
// Adaptee (Legacy class)
public class OldDotMatrixPrinter
{
public void PrintDotMatrix(string data)
{
Console.WriteLine($"Printing with legacy dot matrix: {data}");
}
}
// Adapter class (Object Adapter)
public class PrinterAdapter : IModernPrinter
{
private readonly OldDotMatrixPrinter _oldPrinter;
public PrinterAdapter(OldDotMatrixPrinter oldPrinter)
{
_oldPrinter = oldPrinter;
}
public void Print(string content)
{
_oldPrinter.PrintDotMatrix(content);
}
}
// Client Code
class Program
{
static void Main()
{
IModernPrinter printer = new PrinterAdapter(new OldDotMatrixPrinter());
printer.Print("Hello, Adapter!");
}
}
Output:
Printing with legacy dot matrix: Hello, Adapter!
Simple and effective. Composition provides flexibility because the adapter and adaptee remain loosely coupled.
2. Class Adapter (Inheritance-based)
Class Adapter relies on inheritance. However, C# doesn’t directly support multiple inheritance from classes, so we creatively mix inheritance and interfaces to achieve similar results.
Example (Class Adapter using Interfaces and Inheritance):
// Target Interface
public interface INewLogger
{
void LogInfo(string message);
}
// Legacy class (Adaptee)
public class LegacyLogger
{
public void WriteLog(string logMessage)
{
Console.WriteLine($"Legacy Log Entry: {logMessage}");
}
}
// Class Adapter using Inheritance
public class LoggerAdapter : LegacyLogger, INewLogger
{
public void LogInfo(string message)
{
WriteLog(message);
}
}
// Client Code
class Program
{
static void Main()
{
INewLogger logger = new LoggerAdapter();
logger.LogInfo("Adapter Pattern with Class Adapter");
}
}
Output:
Legacy Log Entry: Adapter Pattern with Class Adapter
Inheritance simplifies the adapter structure, but you sacrifice flexibility as the Adapter tightly couples itself with the Adaptee.
Use Cases for the Adapter Pattern (Real-Life Scenarios)
Still wondering, “Great theory, but where would I actually use this pattern?” Well, here are some realistic scenarios:
1. Legacy System Integration
Imagine integrating a decades-old COBOL accounting system with a modern .NET web application—sounds scary, right? The Adapter Pattern makes it manageable without rewriting the entire COBOL monster.
2. Third-party API Integration
Integrating with multiple payment gateways like PayPal, Stripe, and Authorize.Net? Each has a different API. Use adapters to standardize payment processing logic.
3. Refactoring
Got a messy legacy interface scattered across your codebase? Use adapters to gradually refactor your system without breaking existing functionality.
4. Framework Upgrades
Migrating from older versions of .NET (e.g., .NET Framework to .NET Core)? Adapters allow incremental upgrades without immediate breaking changes.
Advantages of the Adapter Pattern (Why You’ll Love It)
Adapters are like superheroes—quietly solving compatibility issues. Here’s why you’ll become a fan:
- Simplicity in Complexity: Hide messy integration details behind a clean interface.
- Open/Closed Compliance: Extend functionality without changing existing, tested code.
- Improved Maintainability: Clean adapters mean less tangled spaghetti code.
- Reusable Components: Standardized interfaces allow you to swap implementations without headaches.
- Integration Magic: Seamlessly integrate legacy systems, third-party libraries, or diverse APIs.
Disadvantages of the Adapter Pattern (Wait…There’s a Catch?)
Nothing’s perfect—adapters included. Let’s be honest:
- Increased Complexity (if abused): Too many adapters can create extra layers, reducing readability.
- Performance Overhead: Adapters introduce minimal overhead due to interface translation. Usually trivial, but measure carefully in high-performance scenarios.
- Debugging Challenges: Adding extra layers might complicate debugging sessions. “Wait, why am I seeing this method call again?”
Always ask yourself, “Does my project really need an adapter?” If yes—proceed boldly. If not—avoid unnecessary complexity!
Final Thoughts and Conclusion: Adapting Like an Architect!
You’ve come a long way in mastering the Adapter Pattern in C#. You know the what, why, when, and especially the how. You’ve navigated the good, the bad, and even a bit of the ugly.
Adapters are invaluable tools that solve real-world problems—whether integrating old systems, adapting APIs, or refactoring with minimal pain. Yet, they aren’t a silver bullet. Use them wisely and sparingly, and they’ll reward you with maintainable, flexible, and robust software.
So next time you’re faced with incompatible code—remember that trusty travel adapter metaphor. Without tearing apart the hotel wall socket (the legacy system), or rebuilding your laptop charger (your new codebase), just plug in that handy Adapter Pattern, and watch as everything smoothly powers up.
Now, go forth and adapt confidently, architect—because great software isn’t about forcing pieces together; it’s about seamlessly connecting them.
Happy adapting!