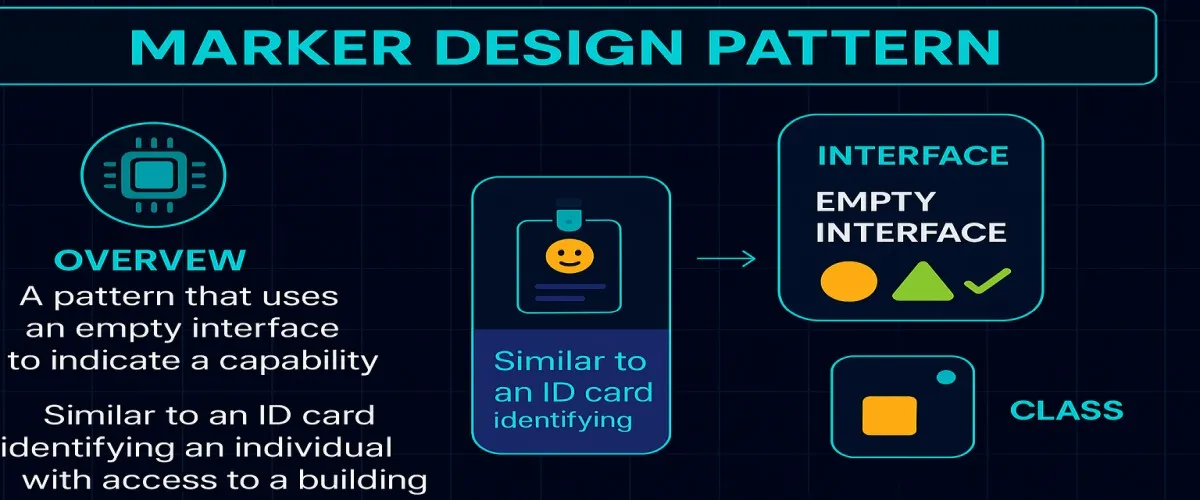
Mastering the Marker Design Pattern in C# – A Practical Guide for Software Architects
- Sudhir mangla
- Design Patterns
- 07 Apr, 2025
Imagine you’re at a bustling airport. Hundreds of travelers rush through security checkpoints every minute. Each traveler carries different kinds of luggage—some have heavy bags, others just a tiny backpack. Security doesn’t individually check every bag in detail; instead, they scan quickly for special tags. Bags with these special tags are flagged immediately, often skipping detailed checks.
Now, what if your application had a similar way to “tag” certain classes, marking them for special handling without cluttering the objects with unnecessary methods or properties? That’s exactly what the Marker Design Pattern is all about!
1. Wait, What Exactly is the Marker Design Pattern?
The Marker Design Pattern (also known as the Tag Interface Pattern) is a nifty design technique in software engineering. It helps you tag certain classes, giving you a simple way to identify them at runtime. But here’s the catch: it doesn’t add any methods or properties to the tagged classes!
Think of it as a silent signal—a code-level “stamp” that says, “Hey, treat me differently!” without explicitly changing behavior directly in the class.
In languages like Java, this pattern is typically implemented with empty interfaces. But in C#, it’s a bit more flexible. You can use interfaces, attributes, or even annotations to implement markers. Cool, right?
2. Core Principles of the Marker Design Pattern
Let’s break it down into a few key principles:
1. Simplicity (Less is More!)
The Marker Pattern doesn’t add complexity to the class structure. It keeps your classes clean, minimalistic, and free of unnecessary methods.
2. Loose Coupling (No Strings Attached)
Classes that use markers aren’t tied down to complex inheritance hierarchies or rigid structures. They’re loosely coupled, making your architecture flexible and maintainable.
3. Runtime Identification (Find it on the Fly!)
Markers allow your app to quickly identify classes at runtime, enabling conditional logic based on the presence or absence of a marker.
4. No Behavioral Intrusion (Silent Signals Only!)
The Marker Pattern should not alter the class behavior directly. If it does, then you’re probably looking at a different pattern (like Decorator or Strategy). Marker interfaces or attributes simply flag the class—they don’t modify it.
3. When Should You Use the Marker Design Pattern?
So, should you slap markers onto everything in your app? Definitely not. Here’s a simple checklist to see if markers are the right choice:
- Do you need a quick way to check if a class has a specific characteristic?
- Does your logic depend on the presence or absence of certain class attributes or categories?
- Do you want to avoid cluttering your classes with extra properties or methods just to indicate a simple characteristic?
If you answered yes to these questions, congratulations! You’re looking at a perfect scenario to use the Marker Design Pattern.
Common scenarios include:
- Serialization (tagging objects for special serialization logic).
- Access control (marking certain classes for permission handling).
- Event handling (quickly filtering event subscribers).
4. Key Components of the Marker Design Pattern
Here’s the beauty of it: the Marker Pattern is incredibly simple. Let’s look at the main players in this game:
1. Marker Interface/Attribute
A simple, empty interface or attribute that acts as a flag.
// Marker Interface
public interface ISpecialSerialization { }
// Or using Attribute
[AttributeUsage(AttributeTargets.Class)]
public class SpecialSerializationAttribute : Attribute { }
2. Concrete Class (Marked Class)
A regular class that implements the marker interface or uses the marker attribute.
// Implementing Interface
public class Customer : ISpecialSerialization
{
public string Name { get; set; }
public int Age { get; set; }
}
// Using Attribute
[SpecialSerialization]
public class Order
{
public int OrderId { get; set; }
public decimal Amount { get; set; }
}
3. Client (Runtime Checker)
A component that uses reflection or simple type checking to determine if the object is marked and handles logic accordingly.
5. Implementation with a Detailed C# Example (Step-by-Step)
Let’s dive into a fully detailed, practical example of the Marker Design Pattern using C#. We’ll build a scenario around a serialization system that handles special serialization for certain objects.
Step 1: Defining a Marker Interface and Attribute
We’ll create two ways to tag objects:
- Marker Interface
- Marker Attribute (more common in C#)
Marker Interface:
public interface ISpecialSerializable { }
Marker Attribute:
[AttributeUsage(AttributeTargets.Class)]
public class SpecialSerializableAttribute : Attribute { }
Step 2: Creating Classes to be Serialized
Let’s have two regular classes:
Product
(no special serialization)Invoice
(requires special serialization)
public class Product
{
public int ProductId { get; set; }
public string ProductName { get; set; }
}
[SpecialSerializable]
public class Invoice
{
public int InvoiceId { get; set; }
public DateTime InvoiceDate { get; set; }
}
Step 3: Serialization Helper Class (The Runtime Checker)
Here’s the exciting part—using reflection to identify marked classes at runtime and serialize accordingly.
public static class SerializationHelper
{
public static string Serialize(object obj)
{
var type = obj.GetType();
// Check for marker attribute
bool isSpecial = Attribute.IsDefined(type, typeof(SpecialSerializableAttribute));
if (isSpecial)
{
return SpecialSerialize(obj);
}
else
{
return DefaultSerialize(obj);
}
}
private static string DefaultSerialize(object obj)
{
// Simple default serialization
return $"Default Serialization: {obj}";
}
private static string SpecialSerialize(object obj)
{
// Special handling for serialization
return $" Special Serialization : {obj}";
}
}
Step 4: Using Our Marker in Action
Let’s test our serialization helper with both classes:
class Program
{
static void Main()
{
var product = new Product { ProductId = 101, ProductName = "Gadget" };
var invoice = new Invoice { InvoiceId = 5001, InvoiceDate = DateTime.Now };
Console.WriteLine(SerializationHelper.Serialize(product));
Console.WriteLine(SerializationHelper.Serialize(invoice));
}
}
Output:
Default Serialization: Product
Special Serialization : Invoice
Step 5: Why This is Powerful (The Magic Moment)
See the magic? Without cluttering Invoice
with serialization logic, we easily differentiated it at runtime. This keeps your classes clean and makes your system extendable. Need another class with special serialization later? Just mark it and move on. No sweat!
6. Different Ways to Implement Marker Pattern in C#
Now that you’re comfortable with the basics, let’s explore some practical ways to implement markers. Just like choosing between driving a car or riding a bike, different approaches fit different scenarios. Let’s dive deeper!
1. Using Marker Interfaces (Traditional Java-Style)
Marker interfaces in C# are just empty interfaces. Easy-peasy!
// Marker Interface
public interface ICacheable { }
// Class implementing marker interface
public class UserProfile : ICacheable
{
public string UserName { get; set; }
public int Age { get; set; }
}
// Runtime Check
public static bool IsCacheable(object obj)
{
return obj is ICacheable;
}
2. Using Attributes (Most Common in C#)
Attributes are like sticky notes—perfect for quickly marking your classes without changing their core structure.
[AttributeUsage(AttributeTargets.Class)]
public class CacheableAttribute : Attribute { }
// Class marked with attribute
[Cacheable]
public class ProductDetails
{
public int ProductId { get; set; }
}
// Checking attribute at runtime
public static bool IsCacheable(object obj)
{
return Attribute.IsDefined(obj.GetType(), typeof(CacheableAttribute));
}
3. Using Custom Reflection Logic (Advanced)
You can even mark classes by naming conventions. Though clever, this method isn’t recommended unless you’re very disciplined.
// Class named with special suffix
public class OrderCacheable
{
public int OrderId { get; set; }
}
// Reflection check based on naming conventions
public static bool IsCacheable(object obj)
{
return obj.GetType().Name.EndsWith("Cacheable");
}
Choose wisely! Attributes are usually best for readability and maintainability in C#, while marker interfaces work well for scenarios needing stronger type-checking.
7. Real-world Use Cases for Marker Patterns in C#
So, when exactly does the Marker Pattern shine? Here’s your handy-dandy checklist of real-world examples:
️ Serialization Control
Mark objects needing special handling (XML, JSON, custom serialization).
️ Caching Logic
Quickly identify cacheable items without cluttering business logic.
️ Access and Permission Checks
Easily mark classes needing special security checks.
️ Event Subscriptions
Identify event listeners easily, helping event-handling mechanisms remain lean and clear.
8. Marker Pattern Anti-Patterns to Avoid (Seriously!)
Ever added too much spice to a dish? Yeah—overusing Marker Patterns is just like that. Here are some common pitfalls:
Marker Overkill
- Issue: Marking everything “just in case.”
- Why It’s Bad: You dilute the value and make your code harder to follow.
Markers That Do More Than Mark
- Issue: Adding actual methods or properties into marker interfaces.
- Why It’s Bad: It violates the simplicity principle. Markers should be silent flags, nothing else.
Using Marker Instead of Inheritance or Composition
- Issue: Using markers to implement actual logic or behavior.
- Why It’s Bad: That’s not their job! Markers are labels, not workers.
9. Advantages of Using Marker Pattern (The Good Stuff!)
Let’s quickly recap why marker patterns rock:
Clean and Minimalistic
Keep your classes clean—no extra junk methods or properties.
Runtime Flexibility
Quickly differentiate classes at runtime—no complicated if-else chains or switch statements.
Loose Coupling
Avoid tight dependencies between classes. It’s like keeping your workplace drama-free!
Easy Maintenance and Extension
New behavior? Just tag a class—done. No massive refactoring needed.
10. Disadvantages of Using Marker Pattern (The Not-So-Great Stuff)
Hey, every superhero has their kryptonite, right? Marker patterns included:
Hidden Behavior
Marker patterns can obscure what happens at runtime if not documented clearly.
Potential Misuse
Because they’re simple, they’re easy to misuse. Mark carefully!
Increased Reflection Overhead
Too many marker checks at runtime might affect performance.
Reduced Clarity If Overused
Too many markers could clutter your architecture, making it tricky to understand logic flow.
11. Marker Pattern Anti-Patterns (Important Enough to Mention Twice!)
Since it’s easy to slip, here’s a quick second reminder about what to avoid:
- Marker interfaces with methods or properties. If it has behavior, it’s no longer a marker.
- Markers used to handle core logic. That’s for Strategy or Decorator patterns!
- Overusing markers in a small codebase. Seriously, markers shine in large-scale apps, not tiny hobby projects.
12. Quick Recap (Marker Pattern at a Glance):
Question | Answer |
---|---|
What is it? | Simple empty interface or attribute used for identification. |
Core Idea: | Tagging classes without adding behavior. |
Use Cases: | Serialization, event handling, permission management. |
Benefits: | Clean code, minimal coupling, runtime flexibility. |
13. Your Next Steps: Practice!
Why not challenge yourself right now?
- Task 1: Try creating another scenario (e.g., event subscription) using Marker Patterns.
- Task 2: Experiment with both interfaces and attributes. See which one fits your scenario better.
You’ll be amazed at how this simple pattern boosts your architectural decisions!
14. Wrapping It Up: The Marker Pattern Conclusion
Let’s wrap up this fun ride with a few key takeaways about the Marker Pattern:
Markers are silent but powerful.
They subtly signal special treatment at runtime without cluttering your codebase.
Best practices in C#:
Use attributes primarily, as they’re more idiomatic and clearer. Marker interfaces are second-best when strong type checks are crucial.
Perfect for these scenarios:
- Serialization
- Caching
- Security checks
- Event management
Avoiding pitfalls:
- No methods or properties in markers!
- Keep it simple and minimal.
- Clearly document what your markers signify.
Now that you’re a Marker Pattern expert, it’s time to tag your classes wisely, keep your architecture sparkling clean, and enjoy the smooth flow of easily identifiable classes in your C# apps.
Remember: Markers are your invisible, helpful stickers. Use them well, and your codebase will thank you later!